Mastering URL Routing in Django
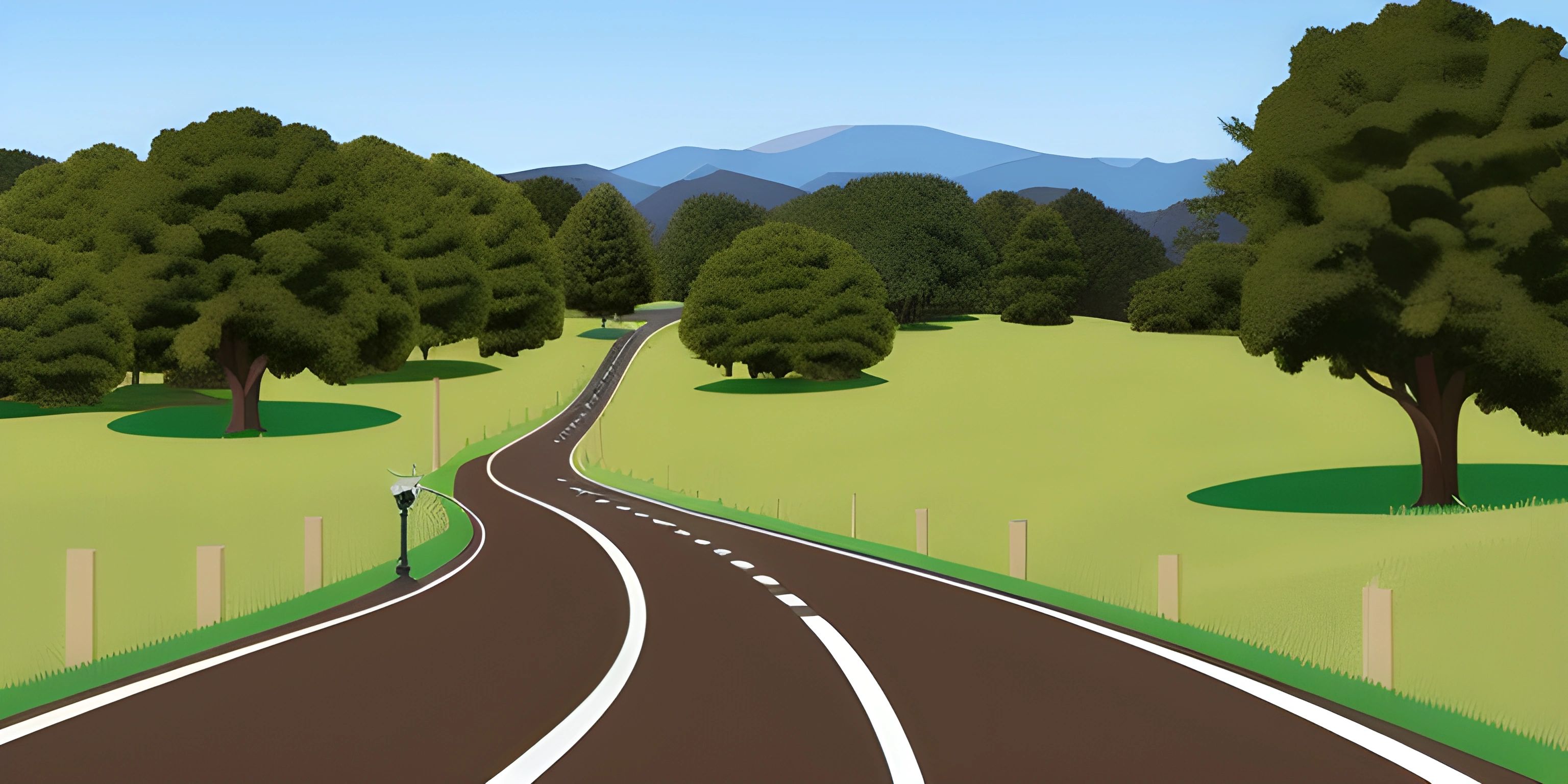
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Before we dive into the nitty-gritty of URL routing in Django, let's paint a picture. Imagine you're building a sprawling mansion. Each room has a specific purpose, and the mansion itself is connected by a series of hallways. You don't want your guests to get lost, so you create a map with clear directions. In Django, URL routing is that map—it guides users and requests to the right places in your web application.
The Basics of URL Routing
In Django, URL routing is managed through a configuration file named urls.py
. This file acts as the central hub for directing traffic to your views. Think of it as the mansion's main hallway that branches off into various rooms.
Here's a simple example to get us started:
# mysite/urls.py from django.contrib import admin from django.urls import path from myapp import views urlpatterns = [ path('admin/', admin.site.urls), path('home/', views.home, name='home'), ]
In this example, we've created a URL route for the admin site and a custom route for a home
view in our application named myapp
. The path
function takes several arguments:
- The route: A string representing the URL pattern.
- The view: A callable (usually a function) that handles the request.
- The name: An optional name for the route, useful for URL reversing.
Path Converters
Django provides a set of path converters that let you capture URL parameters and pass them to your views. This is akin to placing signposts in your mansion's hallways that give additional information about the rooms.
Built-in Path Converters
Here are some common path converters:
str
: Matches any non-empty string, excluding the path separator/
.int
: Matches any integer.slug
: Matches any slug string (letters, numbers, hyphens, and underscores).uuid
: Matches a UUID string.path
: Matches any non-empty string, including the path separator/
.
Let's see them in action:
# myapp/urls.py from django.urls import path from . import views urlpatterns = [ path('article/<int:id>/', views.article_detail, name='article_detail'), path('user/<slug:username>/', views.user_profile, name='user_profile'), ]
In this example, the article_detail
view takes an integer parameter id
, and the user_profile
view takes a slug parameter username
.
Custom Path Converters
You can also create custom path converters if the built-in ones don't meet your needs. Custom path converters give you the flexibility to define your own URL patterns. It's like designing specialized rooms in your mansion that serve unique purposes.
# myapp/converters.py class FourDigitYearConverter: regex = '[0-9]{4}' def to_python(self, value): return int(value) def to_url(self, value): return f'{value:04d}' # myapp/urls.py from django.urls import path, register_converter from . import views, converters register_converter(converters.FourDigitYearConverter, 'yyyy') urlpatterns = [ path('archive/<yyyy:year>/', views.archive, name='archive'), ]
Here, we've created a custom path converter FourDigitYearConverter
that matches a four-digit year.
Namespaces
Django also supports namespaces to organize your URL patterns. Think of namespaces as separate wings in your mansion, each with its own set of rooms. This is particularly useful when you have multiple apps within a project.
Application Namespaces
You define an application namespace in your app's urls.py
file:
# myapp/urls.py from django.urls import path from . import views app_name = 'myapp' urlpatterns = [ path('home/', views.home, name='home'), ]
Then, include it in your project's urls.py
:
# mysite/urls.py from django.contrib import admin from django.urls import include, path urlpatterns = [ path('admin/', admin.site.urls), path('myapp/', include('myapp.urls')), ]
When you want to reverse a URL, you can use the namespace:
from django.urls import reverse url = reverse('myapp:home')
Instance Namespaces
Instance namespaces allow you to include the same application multiple times with different configurations. It's like having identical guest rooms in different wings of your mansion.
# mysite/urls.py from django.urls import include, path urlpatterns = [ path('shop1/', include('shop.urls', namespace='shop1')), path('shop2/', include('shop.urls', namespace='shop2')), ]
Conclusion
URL routing in Django is a powerful tool that helps you map out your web application. Understanding how to use path converters and namespaces can make your routing more organized and intuitive. It's like having a well-planned mansion where every room is easily accessible.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What happens if two URL patterns overlap?
If two URL patterns overlap, Django uses the first matching pattern it finds in the urlpatterns
list. Therefore, the order of URLs in the list is crucial. Make sure to place more specific patterns before more general ones.
Can I use regular expressions in Django URL routing?
As of Django 2.0, the re_path
function is available if you need to use regular expressions in your URL patterns. However, for most cases, the built-in path converters should suffice.
How can I handle 404 errors in Django?
You can create a custom 404 error view by defining a function in your views and setting the handler404
in your urls.py
file. Django will use this view whenever a 404 error occurs.
What is URL reversing?
URL reversing is a way to generate URLs based on the name of the view instead of hardcoding them. This makes your code more maintainable and less error-prone, especially when URL patterns change.
How do I include URL patterns from an app in my project?
Use the include()
function in your project's urls.py
file to include URL patterns from an app. This helps in keeping your URL routing modular and organized.