Docstrings in Python
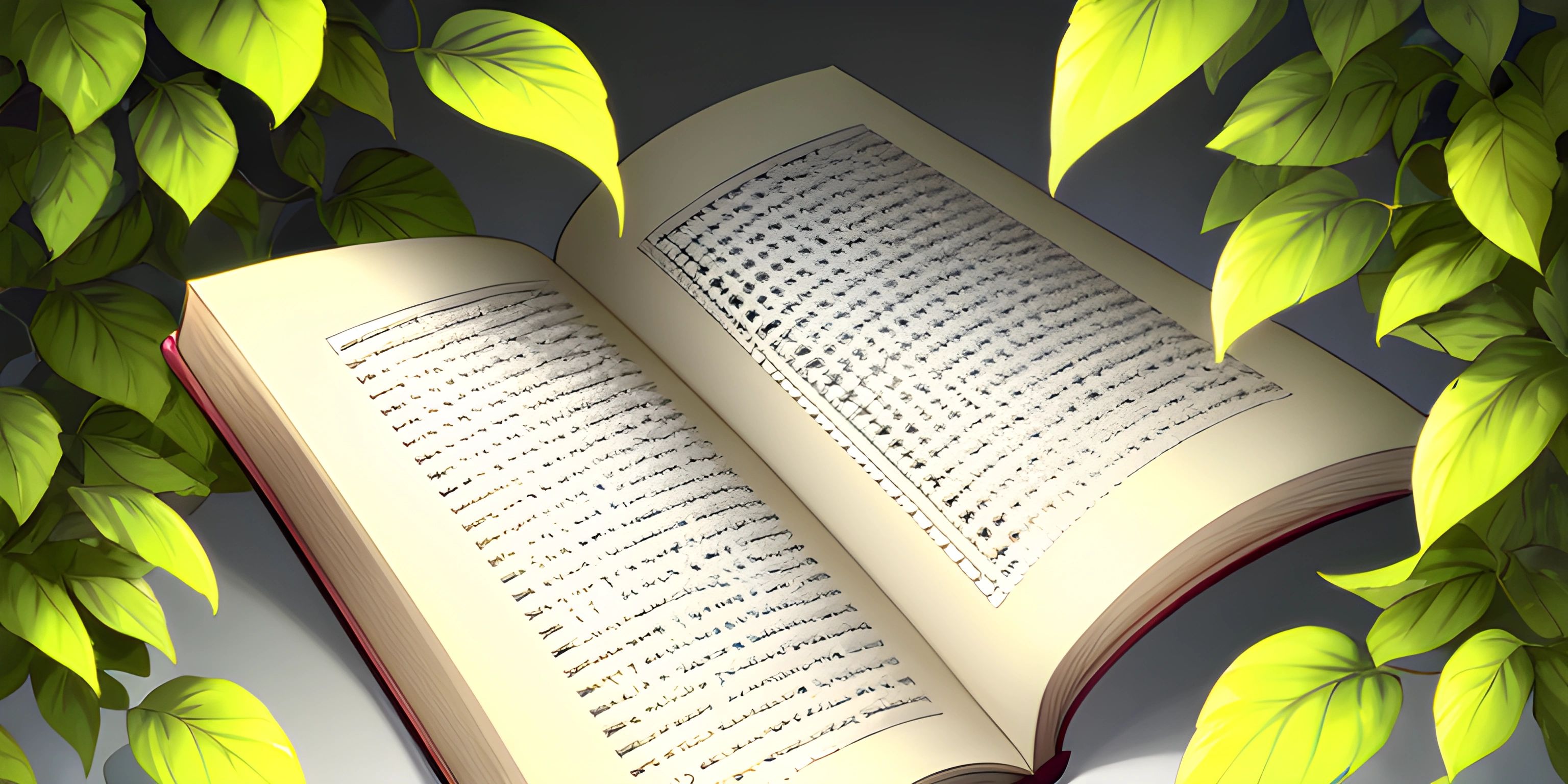
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ah, documentation! The part of programming that often gets neglected, but is crucial for helping others (including our future selves) understand what our code is doing. In Python, we have a neat way to document our code: docstrings. Grab a cup of tea, and let's dive into the wonderful world of Python docstrings.
What are Docstrings?
Docstrings, short for "documentation strings," are special strings in Python that provide a concise yet informative description of what a function, class, or module does. Docstrings are enclosed in triple quotes (either """
or '''
), and are placed immediately after a function, class, or module definition. They are not only useful for humans but can also be accessed by Python programs using the help()
function or other tools, like IDEs and documentation generators.
For example, here's a simple function with a docstring:
def greet(name): """Greets the given name with a friendly message.""" return f"Hello, {name}! Nice to meet you!"
Writing Good Docstrings
Good docstrings are essential for making your code more accessible and maintainable. Here are some best practices to follow when writing docstrings in Python:
-
Keep it concise and informative. A good docstring should tell the reader what the function, class, or module does, without diving into implementation details.
-
Use the appropriate docstring style. There are several widely-accepted docstring styles, such as PEP 257, NumPy, and Google. Choose the one that best suits your project and stick to it consistently.
-
Document parameters, return values, and exceptions. When applicable, include information about the function's parameters, return values, and any exceptions it may raise.
-
Keep your docstrings up to date. As your code evolves, make sure to update your docstrings accordingly to prevent confusion or inconsistencies.
Here's an example of a well-written docstring using the Google style:
def add(a, b): """Adds two numbers and returns the result. Args: a (float): The first number to add. b (float): The second number to add. Returns: float: The sum of the two input numbers. Raises: TypeError: If either of the input arguments is not a number. """ if not (isinstance(a, (int, float)) and isinstance(b, (int, float))): raise TypeError("Both input arguments must be numbers.") return a + b
Accessing Docstrings
Python provides the built-in help()
function to access the docstrings of functions, classes, and modules. Here's how you can use it:
help(add) # Displays the docstring of the 'add' function
You can also access a docstring directly using the __doc__
attribute:
print(add.__doc__) # Prints the docstring of the 'add' function
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Common Programming Pitfalls (psst, it's free!).
FAQ
What are docstrings in Python?
Docstrings are special strings in Python that provide a concise and informative description of what a function, class, or module does. They are enclosed in triple quotes (either """
or '''
) and are placed immediately after a function, class, or module definition.
Why are docstrings important?
Docstrings are important because they help make your code more accessible and maintainable. They provide valuable information to other developers (and your future self) about the purpose and usage of your code. Docstrings can also be accessed by Python programs and tools, such as IDEs and documentation generators.
How can I access a docstring in Python?
You can access a docstring in Python using the built-in help()
function or by accessing the __doc__
attribute of the function, class, or module. For example, help(function_name)
or function_name.__doc__
.
What are some best practices for writing docstrings in Python?
Some best practices for writing docstrings in Python include keeping them concise and informative, using an appropriate docstring style, documenting parameters, return values, and exceptions, and keeping docstrings up to date as your code evolves.