Python Docstrings
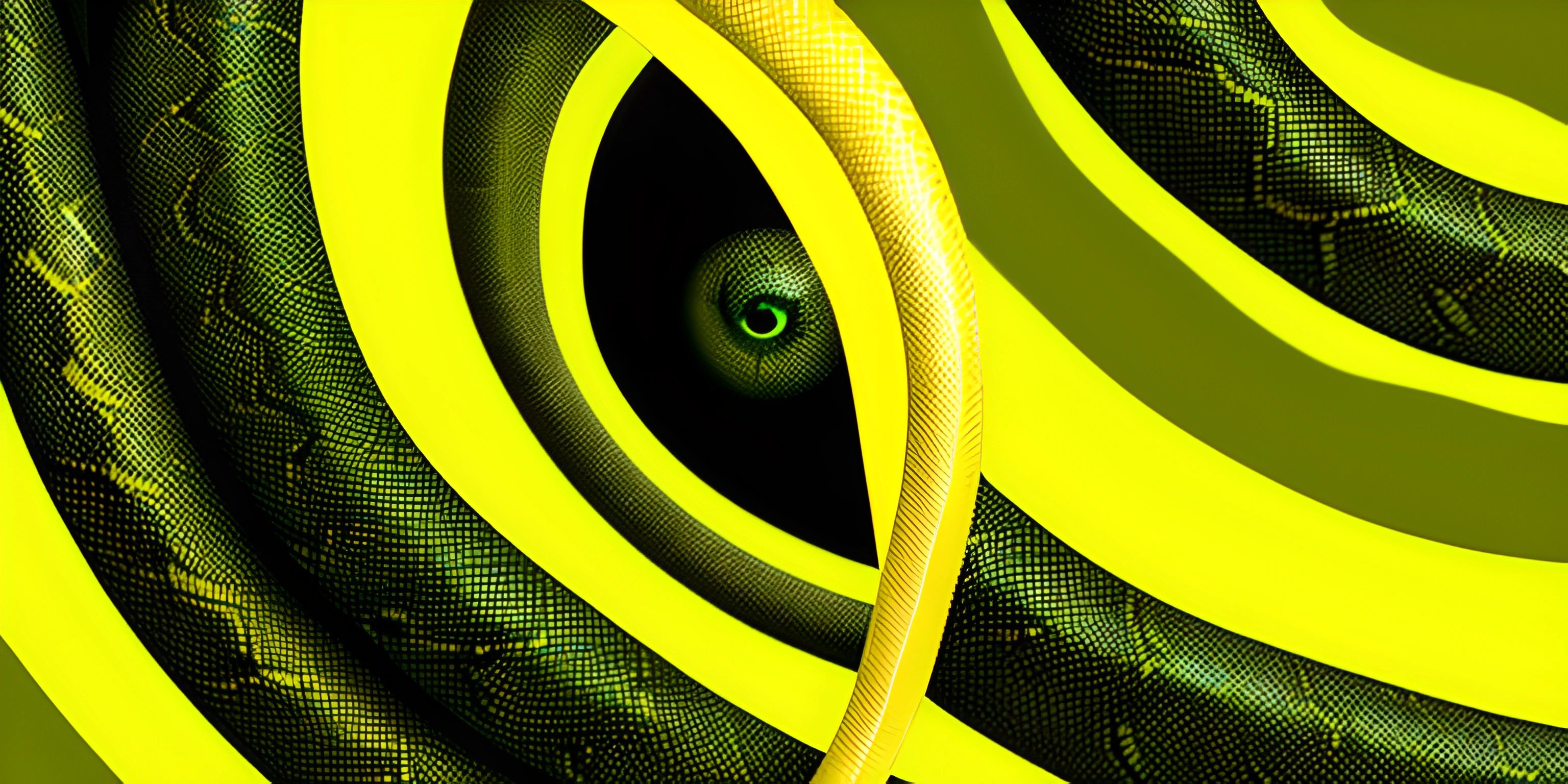
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Every great story has a beginning, a middle, and an end. When it comes to writing code, the story begins with writing clean and efficient code, the middle consists of adding useful comments, and the end is all about proper documentation. In this tale, we will focus on Python docstrings, which help weave a story of understanding for those who read and use your code.
What are Docstrings?
In Python, docstrings or documentation strings are special strings enclosed between triple quotes (''' ''') or triple-double quotes (""" """). They provide a way for you to add comments, explanations, or usage details for your Python functions, classes, and modules. The main idea behind docstrings is to make it easy to understand what a function or a class does, how to use it, and what to expect from it.
Creating Docstrings for Functions
Let's dive into creating docstrings for functions. A docstring should be placed right after the function definition, before the actual code begins. Here's a simple example:
def add_numbers(a, b): """Add two numbers and return the result.""" return a + b
In this example, the docstring explains that the add_numbers
function takes two arguments and returns their sum. Notice how the docstring is located immediately after the function definition line, and is enclosed in triple quotes.
Creating Docstrings for Classes
Docstrings can also be added to classes and their methods. The same rules apply: place the docstring immediately after the class or method definition. Here's an example using a class:
class Dog: """A class representing a dog.""" def __init__(self, name, breed): """Initialize the dog with a name and breed.""" self.name = name self.breed = breed def bark(self): """Make the dog bark.""" print("Woof! Woof!")
In this example, we have a Dog
class with a constructor method (__init__
) and a bark
method. Each method has its own docstring explaining its purpose.
Accessing Docstrings
You might wonder how to access the information stored in docstrings. Python provides a built-in function called help()
that displays the docstring of a function or a class. You can also access the docstring directly using the __doc__
attribute. Here's an example:
help(add_numbers) print(add_numbers.__doc__)
This code will display the docstring we defined for the add_numbers
function.
Docstring Conventions
Now that you know how to create docstrings, it's important to follow some conventions so that your documentation remains consistent and easy to read. Python's official style guide, PEP 257, provides guidelines and best practices for writing docstrings. Some key points include:
- Use triple quotes for docstrings, even if they span only one line.
- Write the docstring as a command, not a description (e.g., "Return the sum" instead of "Returns the sum").
- Include any necessary information about arguments, return values, and potential exceptions.
- Keep the docstring concise and clear.
With these conventions in mind, you'll be able to create well-documented and easy-to-understand Python code. And that, dear reader, is the key to a happy ending in the story of writing code.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).