Java Javadoc
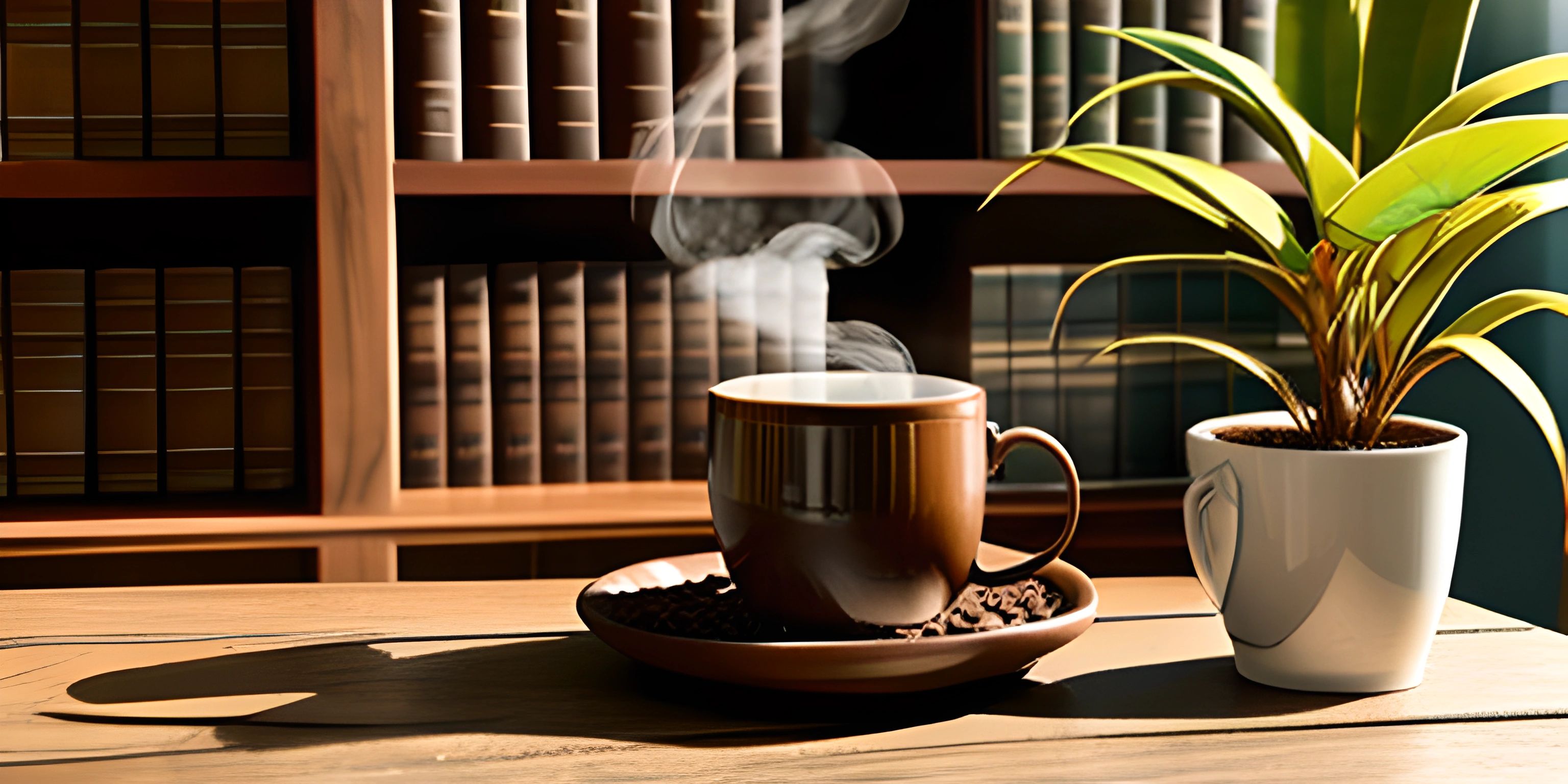
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Javadoc is an essential tool for every Java developer, as it enables the creation of API documentation from comments embedded within the source code. This documentation is invaluable for understanding how a piece of code works and how it's meant to be used, making maintaining and collaborating on projects much more manageable.
Javadoc Basics
Javadoc comments are special multiline comments that start with /**
and end with */
. They can be placed before any class, interface, method, or field declaration. Inside these comments, we can use HTML tags and Javadoc-specific tags for formatting and generating the final documentation.
Let's take a look at a simple Javadoc comment for a class:
/** * This class represents a simple geometric point in a 2D space. * It provides methods to manipulate and retrieve point coordinates. */ public class Point { // ... }
Javadoc Tags
Javadoc provides a set of tags to include specific information in the generated documentation. Let's explore some commonly used tags:
@author
This tag is used to specify the author of the code. You can place it inside the Javadoc comment for a class or interface:
/** * A utility class for performing mathematical operations. * * @author John Doe */ public class MathUtils { // ... }
@param
Used to describe the parameters of a method. Place it inside the Javadoc comment for the method, and it will appear in the generated documentation:
/** * Adds two numbers together. * * @param a the first number * @param b the second number * @return the sum of the two numbers */ public int add(int a, int b) { return a + b; }
@return
Used to describe the return value of a method:
/** * Calculates the factorial of a given number. * * @param n the number to calculate the factorial of * @return the factorial of the given number */ public long factorial(int n) { // ... }
@throws / @exception
Used to document the exceptions that a method might throw:
/** * Parses an integer from a given string. * * @param input the string to parse the integer from * @return the parsed integer * @throws NumberFormatException if the input string is not a valid integer representation */ public int parseInt(String input) throws NumberFormatException { // ... }
Generating Javadoc Documentation
To generate the Javadoc documentation, you can use the javadoc
command-line tool that comes with the JDK. Run it from the terminal with the following syntax:
javadoc -d output_directory source_files
For example, to generate documentation for the Point.java
and MathUtils.java
files and save it in a directory called docs
, you'd run:
javadoc -d docs Point.java MathUtils.java
This will generate a set of HTML files with the documentation that you can open in a web browser.
Conclusion
Javadoc comments are a powerful way to generate and maintain documentation for your Java projects. By using Javadoc tags and proper formatting, you can create clear, concise, and informative documentation that will be invaluable for understanding and collaborating on projects. Make sure to include Javadoc comments in your projects and encourage others to do so as well.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Common Programming Pitfalls (psst, it's free!).
FAQ
What is Javadoc in Java?
Javadoc is a documentation tool that comes with the Java Development Kit (JDK). It is used to generate API documentation in HTML format from Java source code, using specially formatted comments called Javadoc comments. Javadoc comments provide developers with a standard way to document their code, making it easier to understand and maintain.
How do I write Javadoc comments in Java?
Javadoc comments begin with /**
and end with */
. They are placed immediately before the class, interface, method, or field they're documenting. Here's an example of a Javadoc comment for a method:
/** * Calculates the area of a rectangle. * * @param width The width of the rectangle. * @param height The height of the rectangle. * @return The area of the rectangle. */ public double calculateArea(double width, double height) { return width * height; }
What are some common Javadoc tags, and how do I use them?
Javadoc tags provide specific information about the code being documented. Some common Javadoc tags include:
@param
: Describes a method parameter.@return
: Describes the return value of a method.@throws
(or@exception
): Describes the exceptions a method can throw.@author
: Indicates the author of the code.@since
: Specifies the version when the code was added. Here's an example using multiple Javadoc tags:
/** * Divides two numbers. * * @param a The dividend. * @param b The divisor. * @return The result of the division. * @throws ArithmeticException If the divisor is zero. * @author John Doe * @since 1.0 */ public double divide(double a, double b) throws ArithmeticException { if (b == 0) { throw new ArithmeticException("Division by zero is not allowed"); } return a / b; }
How do I generate Javadoc HTML files from my Java source code?
To generate Javadoc HTML files, open a command prompt or terminal and navigate to the directory containing your Java source files. Run the following command, replacing "output_directory" with your desired output directory and "source_files" with your Java source files:
javadoc -d output_directory source_files
For example:
javadoc -d docs MyClass.java
This will generate the Javadoc HTML files in the "docs" directory.
Can I reference other classes or methods in my Javadoc comments?
Yes, you can reference other classes, methods, or members in your Javadoc comments using the {@link}
and {@linkplain}
tags. The {@link}
tag creates a hyperlink to the referenced class or member, while the {@linkplain}
tag creates a plain-text reference without a hyperlink. Here's an example:
/** * Adds two numbers together. This is equivalent to calling * {@link #multiply(double, double)} with one of the arguments as 1. * * @param a The first number. * @param b The second number. * @return The sum of the two numbers. */ public double add(double a, double b) { return multiply(a, 1) + multiply(b, 1); }