JavaScript JSDoc Annotations
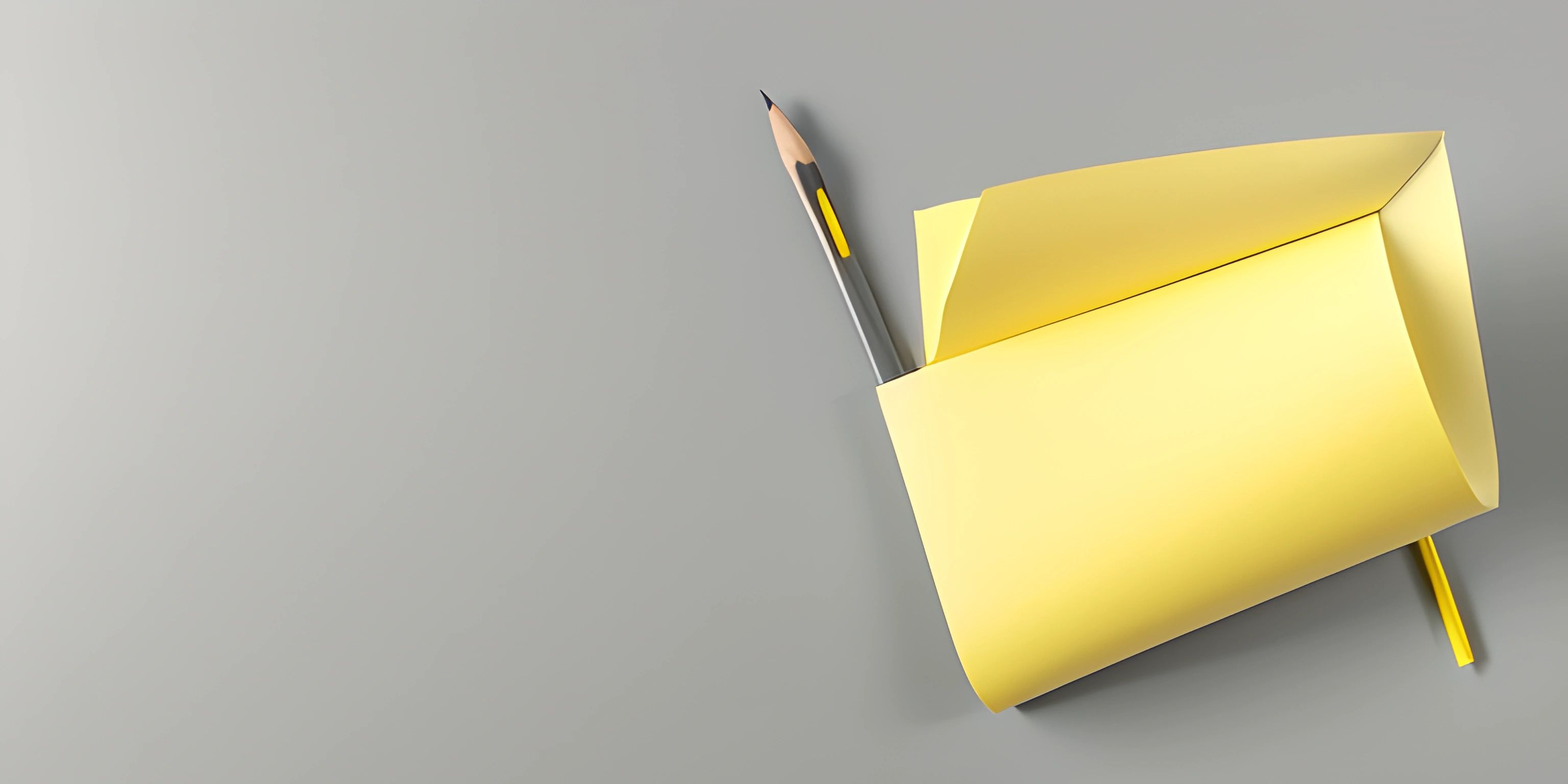
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
JSDoc is a popular documentation generator for JavaScript, allowing developers to annotate their code with comments and JSDoc-specific tags that make it easy to generate documentation pages. By harnessing the power of JSDoc, you can create clear, helpful, and easy-to-understand documentation for your projects.
JSDoc Basics
To use JSDoc, you'll need to write specially-formatted comments above your functions, classes, and other code elements. These comments begin with /**
and end with */
. Within these comments, you can use JSDoc-specific tags to further describe your code. Let's start with a simple example:
/** * Adds two numbers together. * @param {number} a - The first number. * @param {number} b - The second number. * @returns {number} The sum of a and b. */ function add(a, b) { return a + b; }
In this example, we've written a simple add()
function and provided a JSDoc comment above it. The comment begins with a brief description of the function, followed by specific JSDoc tags:
@param
: Describes an input parameter for the function. It includes the type (number
, in this case), the parameter's name (a
), and a description.@returns
: Describes the return value of the function. It includes the type (number
) and a description.
Common JSDoc Tags
There are many other JSDoc tags that you can use to document your JavaScript code. Here are some of the most common:
@constructor
: Indicates that a function is a constructor for a class.@class
: Indicates that the function is a class (useful if you're not using theclass
keyword).@property
: Describes a property of a class or object.@extends
: Indicates that a class or object inherits from another class or object.@private
: Indicates that a member is private and should not be used outside of its containing class.@public
: Indicates that a member is public and can be used freely.@deprecated
: Indicates that a function, method, or property is deprecated and should not be used.
Documenting Classes
JSDoc is also great at documenting classes and their properties and methods. Let's take a look at an example:
/** * Represents a point in two-dimensional space. * @constructor * @param {number} x - The x-coordinate. * @param {number} y - The y-coordinate. */ class Point { constructor(x, y) { this.x = x; this.y = y; } /** * Calculate the distance between this point and another point. * @param {Point} other - The other point. * @returns {number} The distance between the two points. */ distance(other) { const dx = this.x - other.x; const dy = this.y - other.y; return Math.sqrt(dx * dx + dy * dy); } }
In this example, we've documented a Point
class with a constructor and a distance()
method. We use the @constructor
tag to indicate that the Point
function is a class constructor, and the @param
and @returns
tags are used similarly to the previous example.
Generating Documentation
Once you've added JSDoc comments to your code, you can use the JSDoc command-line tool to generate an HTML documentation site. Install the tool using npm
:
npm install -g jsdoc
Then, run it on your JavaScript files:
jsdoc myfile.js
This will generate an out
folder containing the HTML documentation site, which you can open in your browser to view the generated documentation.
JSDoc is a powerful tool for making your JavaScript code more understandable, maintainable, and professional. By adopting JSDoc in your projects, you're investing in the future of your codebase and helping your fellow developers. Happy documenting!
FAQ
What is JSDoc and why should I use it in my JavaScript code?
JSDoc is a documentation generator for JavaScript code. It uses specially formatted comments to generate easy-to-read, customizable documentation that helps developers understand your code better. By using JSDoc, you can make your code more maintainable, reduce the learning curve for new developers, and create an organized, professional-looking documentation.
How do I add JSDoc annotations to my JavaScript code?
To add JSDoc annotations, you'll use specially formatted comments that start with /**
followed by your annotations on each line using an asterisk *
. Here's an example:
/** * This function adds two numbers. * @param {number} a - The first number to add. * @param {number} b - The second number to add. * @return {number} - The sum of the two numbers. */ function add(a, b) { return a + b; }
What are some common JSDoc tags and their syntax?
There are many JSDoc tags available, but some common ones include:
@param
: Documents a function parameter with its type, name, and description.@return
or@returns
: Documents the return value of a function with its type and description.@type
: Documents the type of a variable, property, or object.@namespace
: Documents a collection of related methods and properties.@module
: Documents a module with a description.
How can I generate HTML documentation from my JSDoc-annotated JavaScript code?
You'll need to install the JSDoc tool using npm, like this:
npm install -g jsdoc
Then, to generate the HTML documentation, run the following command:
jsdoc yourJavaScriptFile.js
This will generate an out
directory containing the HTML documentation.
Can I customize the appearance of my generated documentation?
Yes, you can customize the appearance of your generated documentation by using templates. There are numerous JSDoc templates available, or you can create your own. To use a template, install it via npm and then pass the -t
or --template
option when running the jsdoc
command, like this:
jsdoc yourJavaScriptFile.js -t /path/to/your/template