Control Structures
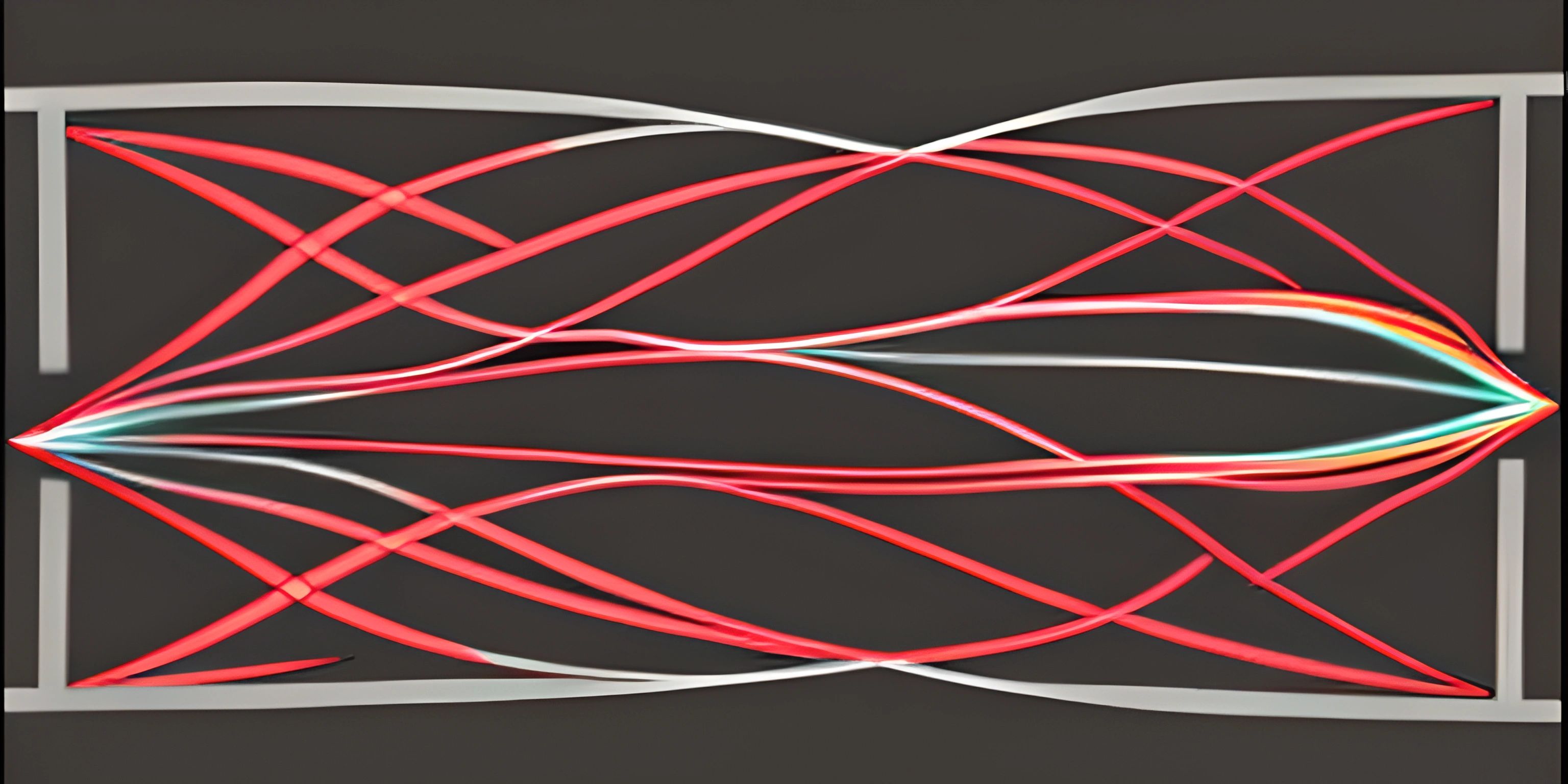
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Programming is like cooking a delicious meal. You mix and match ingredients, follow a recipe, and use precise techniques to create a culinary masterpiece. But, what if you want to add some flair to your dish, or need to accommodate different taste preferences? Control structures are the spices and seasonings that add variety and flexibility to your code. In other words, control structures help you control the flow of your program.
Conditionals
The most common control structure is the conditional. A conditional allows you to execute a block of code only if a certain condition is met. Think of it as a fork in the road – you can choose which path to take based on specific criteria. The simplest form of a conditional is the if
statement.
If Statements
An if
statement checks whether a condition is true
. If so, it executes the code within its block. Here's an example in Python:
temperature = 75 if temperature > 70: print("It's warm outside!")
In this example, if the temperature
is greater than 70, the program will print "It's warm outside!". If the condition is not met, the program skips the print
statement and continues.
Else Statements
To add an alternative path, you can use the else
statement. It will execute a block of code if the condition in the if
statement is false
. Here's the previous example with an added else
statement:
temperature = 60 if temperature > 70: print("It's warm outside!") else: print("It's chilly outside!")
In this case, since the temperature
is not greater than 70, the program prints "It's chilly outside!" instead.
Loops
Sometimes, you need to repeat a block of code multiple times. That's where loops come in handy. Loops allow you to execute a block of code repeatedly based on a condition or a predetermined number of times.
For Loops
A for
loop iterates over a sequence (like a list or a range) and executes the code within its block for each item in the sequence. Here's an example in JavaScript:
for (let i = 1; i <= 5; i++) { console.log("Iteration", i); }
This for
loop will print the word "Iteration" followed by the current value of i
(from 1 to 5) on each iteration.
While Loops
A while
loop continues to execute the code within its block as long as a certain condition is true
. It's like a repeating if
statement. Here's an example in Python:
counter = 1 while counter <= 5: print("Counter:", counter) counter += 1
This while
loop will print "Counter:" followed by the current value of counter
(from 1 to 5) as long as counter
is less than or equal to 5.
Recap
Control structures, such as conditionals and loops, allow you to add flexibility and control to your program's flow. With if
, else
, for
, and while
statements, you can create versatile code recipes that adapt to different situations – just like a master chef!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
What are control structures in programming?
Control structures are fundamental building blocks in programming that help you manage the flow of your code. They allow you to make decisions, repeat tasks, and create more efficient and effective programs. There are two main types of control structures: conditionals and loops. Conditionals let you execute specific code based on certain conditions, while loops enable you to perform tasks repeatedly until a certain condition is met.
How do conditionals work in programming?
Conditionals are a type of control structure that allows you to execute specific code based on whether a certain condition is true or false. The most common conditional is the if
statement. The basic structure of an if
statement looks like this (in Python):
if condition: # Code to execute if the condition is true else: # Code to execute if the condition is false
You can also use elif
(short for "else if") to test multiple conditions in a single conditional structure.
Can you give an example of a loop in programming?
Sure! Loops are control structures that allow you to perform a set of tasks repeatedly until a certain condition is met. There are two main types of loops: for
loops and while
loops. Here's an example of a for
loop in Python:
for i in range(5): print("Hello, World!")
This code will print "Hello, World!" five times. The for
loop iterates over the range of numbers from 0 to 4 (inclusive), executing the print()
function at each iteration.
What is the difference between a for loop and a while loop?
The main difference between for
and while
loops is how they determine when to stop repeating the tasks. A for
loop iterates through a predefined set of values or a range, while a while
loop continues to execute as long as a specified condition remains true.
Here's an example of a while
loop in Python:
counter = 0 while counter < 5: print("Hello, World!") counter += 1
This code will also print "Hello, World!" five times. The while
loop continues to execute as long as the counter
variable is less than 5. At each iteration, the value of counter
is incremented by 1, and once it reaches 5, the loop stops.