Assembly Control Structures
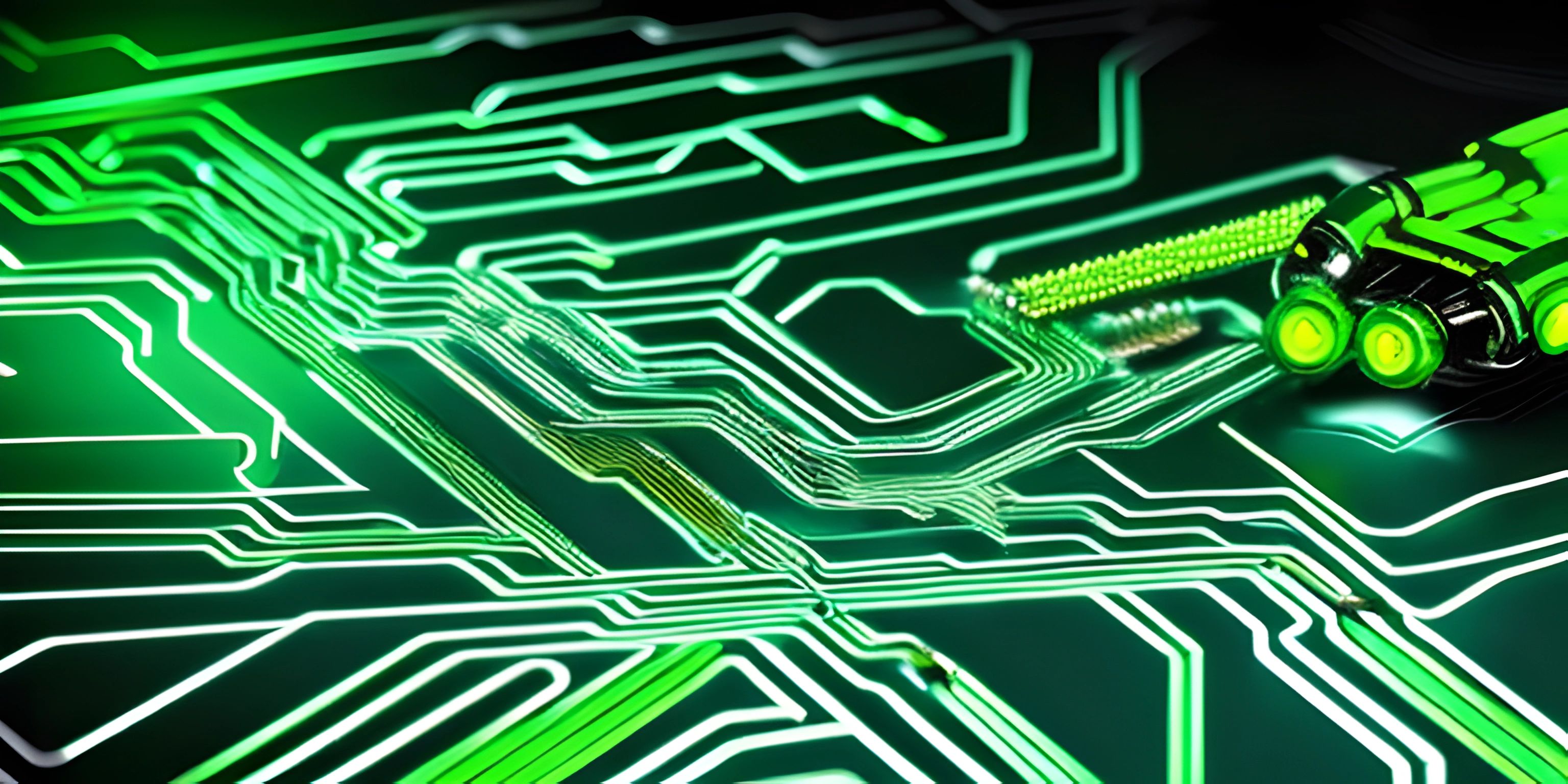
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Programming in assembly language presents unique challenges that often require a different approach than what we might be used to in high-level languages. Control structures, such as loops and conditionals, are fundamental in shaping the flow of a program. In this journey, we'll navigate the world of control structures and flow control in assembly language, discovering how to bend the program's path to our will.
Assembly Loops
In high-level languages, we have the luxury of using built-in loop structures like for
and while
to repeat code blocks. However, assembly language doesn't provide these structures directly. Instead, we utilize a combination of jumps and conditionals to create loops.
Counted Loops
A counted loop repeats a code block a specific number of times. Let's illustrate this with an example in x86 assembly:
mov ecx, 5 ; Set the loop counter to 5 start_loop: ; Code to repeat 5 times goes here dec ecx ; Decrease the loop counter by 1 jnz start_loop ; If the loop counter is not zero, jump back to start_loop
Here, we use the mov
instruction to set the loop counter (ecx
) to 5. The jnz
(jump if not zero) instruction checks whether ecx
is zero or not. If it's not zero, the program jumps back to the start_loop
label and repeats the code block inside the loop.
Infinite Loops
An infinite loop repeats code indefinitely until an external event, like an interruption, breaks it. Here's an example:
infinite_loop: ; Your code here jmp infinite_loop ; Jump back to the beginning of the loop
The jmp
(jump) instruction sends the program back to the infinite_loop
label, creating an endless loop.
Assembly Conditionals
Conditionals are another essential control structure that allows a program to make decisions based on conditions. In assembly language, we implement conditionals using jump instructions and flags.
If-Else Structure
Let's create an if-else structure to compare two numbers:
cmp eax, ebx ; Compare eax and ebx jg greater_than ; If eax > ebx, jump to greater_than ; Code for eax <= ebx here jmp end_if_else ; Jump to end_if_else greater_than: ; Code for eax > ebx here end_if_else:
We use the cmp
instruction to compare eax
and ebx
and set flags accordingly. The jg
(jump if greater) instruction checks if the result of the comparison is greater and jumps to the greater_than
label if true. If the condition is false, the program continues executing the next instructions.
Conclusion
Control structures and flow control are integral to any programming language. In assembly language, we achieve these using jumps, labels, and conditionals. With a good understanding of assembly loops and conditionals, you can craft intricate and efficient low-level programs. Now, go forth and bend the assembly to your will!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
What are the basic control structures in assembly language?
Assembly language provides a set of basic control structures that allow you to create more complex programs. These control structures include:
- Loops: Used to execute a sequence of instructions multiple times.
- Conditionals: Used to execute a sequence of instructions based on specific conditions.
- Jumps: Used to change the flow of execution to a different part of the program.
How do I create loops in assembly language?
Loops in assembly language are created using jump instructions and comparison operations. Here's an example of a simple loop that counts from 0 to 9:
mov ecx, 10 ; Initialize counter to 10 loop_start: ; Your loop body code goes here dec ecx ; Decrease counter by 1 jnz loop_start ; Jump to loop_start if counter is not zero
How do I implement conditional statements in assembly?
Conditional statements can be implemented using comparison operations and jump instructions. Here's an example of a simple if-else statement in assembly:
cmp eax, ebx ; Compare eax and ebx jge if_true ; Jump to if_true block if eax >= ebx ; else block ; Your 'else' code goes here jmp end_if ; Jump to the end of the if-else construct if_true: ; Your 'if' code goes here end_if:
Can I use switch-case statements in assembly language?
While there's no direct equivalent to switch-case statements in assembly language, you can implement a similar construct using jump tables. Jump tables are arrays of memory addresses that correspond to different cases. You'll need to use an indirect jump instruction to jump to the appropriate case based on the value of a register.
How can I create subroutines in assembly?
Subroutines, also known as functions or procedures, can be created in assembly language using the call
and ret
instructions. The call
instruction pushes the current address onto the stack and jumps to the subroutine. The ret
instruction pops the address from the stack and jumps back to the caller. Here's an example of a simple subroutine:
; Main program ; Your main program code goes here call my_subroutine ; Code after the subroutine call ; Subroutine definition my_subroutine: ; Your subroutine code goes here ret ; Return to the caller