Elixir Variables and Data Types
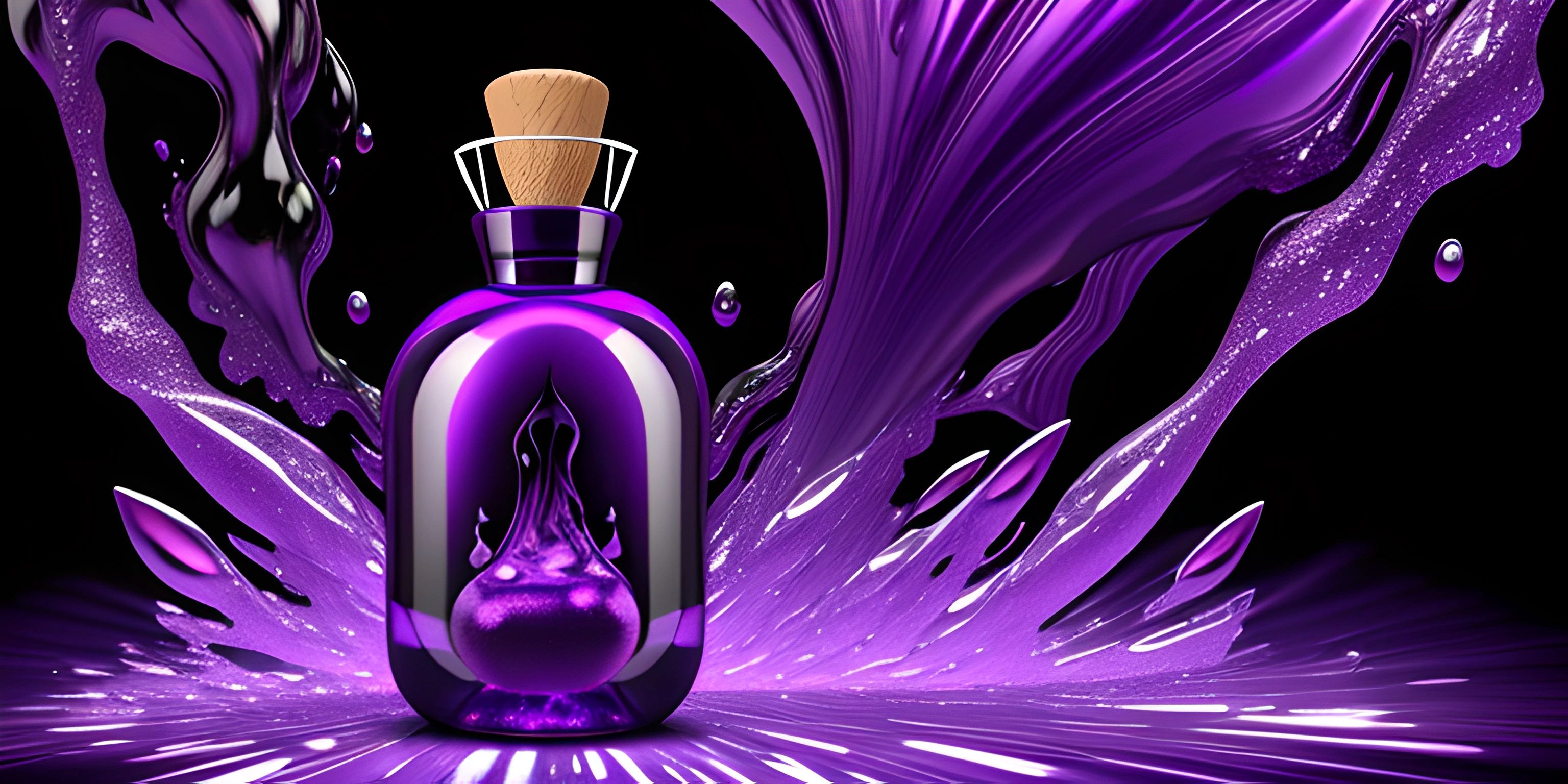
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Elixir, a functional programming language built on top of the Erlang VM, comes with some unique features and syntax that set it apart from other languages. One of the fundamental aspects of any programming language is how it handles variables and data types. So, let's dive into Elixir's world of variables and data types!
Variables
In Elixir, variables are used to store data and can be bound to values using the =
operator. Once a variable is assigned, its value can be used throughout the program. Here's a simple example:
name = "Alice" age = 30
In this example, the variable name
gets assigned the value "Alice", and the variable age
gets assigned the value 30.
But what's interesting about Elixir is that variables can be reassigned. This means we can change the value of a variable after it has been initialized:
name = "Alice" name = "Bob"
Now, the value of name
is "Bob", even though it was initially assigned to "Alice".
Data Types
Elixir supports several data types, which determine the kind of values a variable can hold. Let's explore some of the most common ones:
Integer
Integers are whole numbers, both positive and negative. In Elixir, integers can be written in different bases, like binary, octal, and hexadecimal. Here's a quick example:
decimal = 42 binary = 0b101010 octal = 0o52 hexadecimal = 0x2A
All of these variables represent the same value, 42, but in different bases.
Float
Floating-point numbers, or floats, represent real numbers with a decimal point. They provide a way to represent fractional values in Elixir:
pi = 3.141592
Atom
Atoms are constants that represent their own name. They are denoted by a colon, followed by the atom's name:
:ok :error :alice
Atoms are often used as identifiers, status codes, or keys in maps (which we'll see later).
String
Strings are sequences of characters enclosed in double quotes:
greeting = "Hello, Elixir!"
Elixir strings are encoded in UTF-8, which means they can include any Unicode character.
Tuple
Tuples are ordered collections of values, enclosed in curly braces:
coordinates = {42, 87}
Tuples can contain different data types and can be indexed using the elem/2
function:
elem(coordinates, 0) # returns 42
List
Lists are ordered collections of values, enclosed in square brackets:
languages = ["Elixir", "Erlang", "Ruby"]
Lists can also contain different data types and can be manipulated using built-in functions such as hd/1
and tl/1
:
hd(languages) # returns "Elixir" tl(languages) # returns ["Erlang", "Ruby"]
Map
Maps are key-value stores, enclosed in percent signs and curly braces:
person = %{name: "Alice", age: 30}
You can access a map's values using the Map.get/2
function or by using the dot notation:
Map.get(person, :name) # returns "Alice" person.name # also returns "Alice"
Now that you've been introduced to Elixir's variables and data types, you're ready to start exploring its pattern matching, control structures, and other powerful features. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What are the basic data types in Elixir?
Elixir has several basic data types, including:
- Integers: Whole numbers, such as 5, -10, or 42
- Floats: Decimal numbers, such as 3.14, 0.001, or -7.89
- Booleans: True or false values, represented by
true
andfalse
- Atoms: Constants that represent their own name, such as
:ok
,:error
, or:hello
- Strings: Sequences of characters, such as "Hello, world!" or "Elixir is awesome!"
- Lists: Ordered collections of values, such as [1, 2, 3] or ["apple", "banana", "cherry"]
- Tuples: Similar to lists, but with a fixed size, such as {1, 2, 3} or {:ok, "message"}
How do you declare and use variables in Elixir?
In Elixir, you create variables by assigning a value to a name using the =
operator. For example:
number = 42 message = "Hello, world!" fruits = ["apple", "banana", "cherry"]
Once declared, you can use variables in expressions, functions, or data structures.
How do you perform type conversion in Elixir?
Elixir provides several functions to convert between data types. Some common examples include:
- To convert an integer to a float, use
float/1
:float(42)
→42.0
- To convert a float to an integer, use
trunc/1
:trunc(3.14)
→3
- To convert a number to a string, use
Integer.to_string/1
orFloat.to_string/1
:Integer.to_string(42)
→"42"
- To convert a string to an integer or float, use
String.to_integer/1
orString.to_float/1
:String.to_integer("42")
→42
What are the differences between lists and tuples in Elixir?
Lists and tuples in Elixir are both ordered collections of values, but they have some key differences:
- Lists: Allow dynamic resizing and are implemented as linked lists, making them suitable for operations like adding or removing elements. However, accessing an element by index is slower.
my_list = [1, 2, 3] new_list = [0 | my_list] # Adds 0 to the beginning of the list Enum.at(my_list, 1) # Returns the second element (2)
- Tuples: Have a fixed size and are stored contiguously in memory, allowing for faster element access. However, adding or removing elements requires copying and modifying the entire tuple.
my_tuple = {1, 2, 3} updated_tuple = Tuple.put_elem(my_tuple, 1, 42) # Replaces the second element with 42 elem(my_tuple, 1) # Returns the second element (2)
Can Elixir variables be reassigned?
Yes, variables in Elixir can be reassigned. When you reassign a variable, it takes on a new value and loses its previous value. For example:
count = 5 count = count + 1 # Reassigns count to 6