Mastering Express for Node.js Web Development
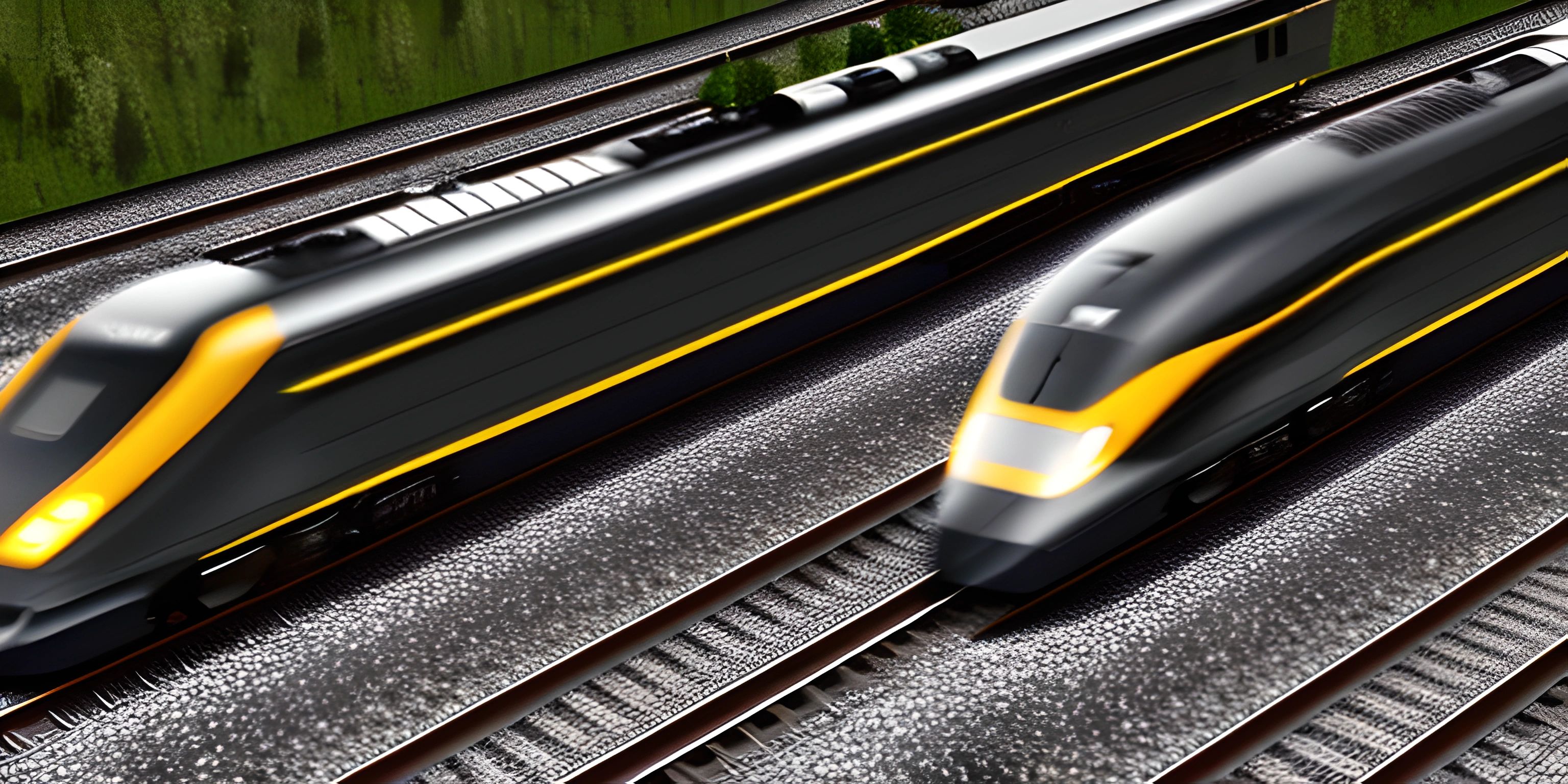
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Dive into the world of web development with Express, an incredibly popular and powerful framework for Node.js. Express simplifies the process of creating web applications and APIs, making it easier than ever to build robust and feature-rich web experiences.
Setting Up Express
To get started with Express, you'll first need to have Node.js installed. If you haven't yet, head over to the official Node.js website and download the latest version.
Once Node.js is installed, you can create a new directory for your Express project and run the following command to initialize a new Node.js application:
npm init -y
Now, let's install Express using npm:
npm install express
With Express installed, we can start building our web application.
Creating a Simple Express Server
In the project directory, create a new file called app.js
. This will be the entry point for our Express application. Open the file and add the following code:
const express = require("express"); const app = express(); const PORT = process.env.PORT || 3000; app.get("/", (req, res) => { res.send("Hello, world!"); }); app.listen(PORT, () => { console.log(`Server is running at http://localhost:${PORT}`); });
Let's break down this code:
- We
require
the Express module and create a new Express application by calling theexpress()
function. - We define a
PORT
constant to determine the port our server will listen on. - We create a simple route handler for the root route (
/
) using theapp.get()
method. When a request comes in for this route, the server will send back "Hello, world!" as the response. - We start the server using the
app.listen()
method, which takes the port number and a callback function that gets executed once the server is up and running.
To start the server, run the following command:
node app.js
You should see the message "Server is running at http://localhost:3000" in your terminal. Open your browser and navigate to the URL, and you'll be greeted with "Hello, world!".
Routing and Middleware
Express makes it easy to handle different routes and HTTP methods. For example, to create a new route for a user profile, you could write:
app.get("/profile/:userId", (req, res) => { res.send(`Profile page for user with ID ${req.params.userId}`); });
In this example, we use a route parameter (:userId
) to capture the user ID from the URL. We can access this value using req.params.userId
.
Express also supports middleware, which are functions that have access to the request and response objects and can modify them before they reach the route handler. Middleware functions can be added globally (applied to all routes) or locally (applied to specific routes). For example, to log the time and method for each request, you could create a middleware function like this:
const requestLogger = (req, res, next) => { console.log(`[${new Date().toISOString()}] ${req.method} ${req.url}`); next(); }; app.use(requestLogger);
We define the requestLogger
middleware and then use the app.use()
method to apply it to all routes. The next()
function is called to pass control to the next middleware in the chain or to the route handler if there are no more middleware functions.
With your newfound knowledge of Express, you're ready to embark on your journey into web development. Remember to check out the official Express documentation for more advanced features, and happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is Express and why should I use it for Node.js web development?
Express is a popular and powerful web application framework for Node.js. It simplifies the development of web applications by providing a robust set of features and tools that make it easy to create, maintain, and scale web applications. Some advantages of using Express include:
- Simplified routing and middleware management
- Support for template engines and static content
- Easy integration with databases and other third-party services
- High-performance and lightweight architecture
How do I install Express and start building my first web app?
To install Express, you'll first need to have Node.js and npm (Node Package Manager) installed on your system. Then, you can install Express using the following command:
npm install express --save
Now, you can create a simple Express app using the following code in a file named app.js
:
const express = require("express"); const app = express(); const port = 3000; app.get("/", (req, res) => { res.send("Hello, World!"); }); app.listen(port, () => { console.log(`App is listening at http://localhost:${port}`); });
Finally, run your app using the command:
node app.js
Visit http://localhost:3000
in your browser to see your Express app in action!
How can I use middleware in an Express app?
Middleware functions are essential in Express apps as they help manage the request-response cycle. You can use built-in middleware, third-party middleware, or create your own custom middleware. To use middleware in your app, simply use the app.use()
method. Here's an example of using the built-in express.json()
middleware to parse incoming JSON data:
const express = require("express"); const app = express(); app.use(express.json()); app.post("/data", (req, res) => { console.log(req.body); res.send("Data received!"); }); // ... other routes and middleware ... app.listen(3000, () => { console.log("App is listening on port 3000"); });
How do I create and use routes in an Express app?
Routes in Express define how your application responds to different client requests. You can create a route using various HTTP methods, such as GET, POST, PUT, and DELETE. To create a route, use the app.METHOD()
function, where METHOD
is an HTTP method. For example:
const express = require("express"); const app = express(); app.get("/", (req, res) => { res.send("Hello, World!"); }); app.post("/submit", (req, res) => { // Handle form submission logic }); app.put("/update", (req, res) => { // Handle data update logic }); app.delete("/delete", (req, res) => { // Handle data deletion logic }); app.listen(3000, () => { console.log("App is listening on port 3000"); });
How can I serve static files like images and stylesheets using Express?
Express provides a built-in middleware called express.static()
to serve static files like images, CSS files, and JavaScript files. To use it, simply include the following line in your code, replacing 'public'
with the name of your directory containing static files:
app.use(express.static("public"));
Now, you can place your static files in the designated directory, and they will be served automatically based on their file paths. For example, if you have a file named style.css
in the public
folder, it will be accessible at http://localhost:3000/style.css
.