Using and Creating Express Middleware for Flexible Routing
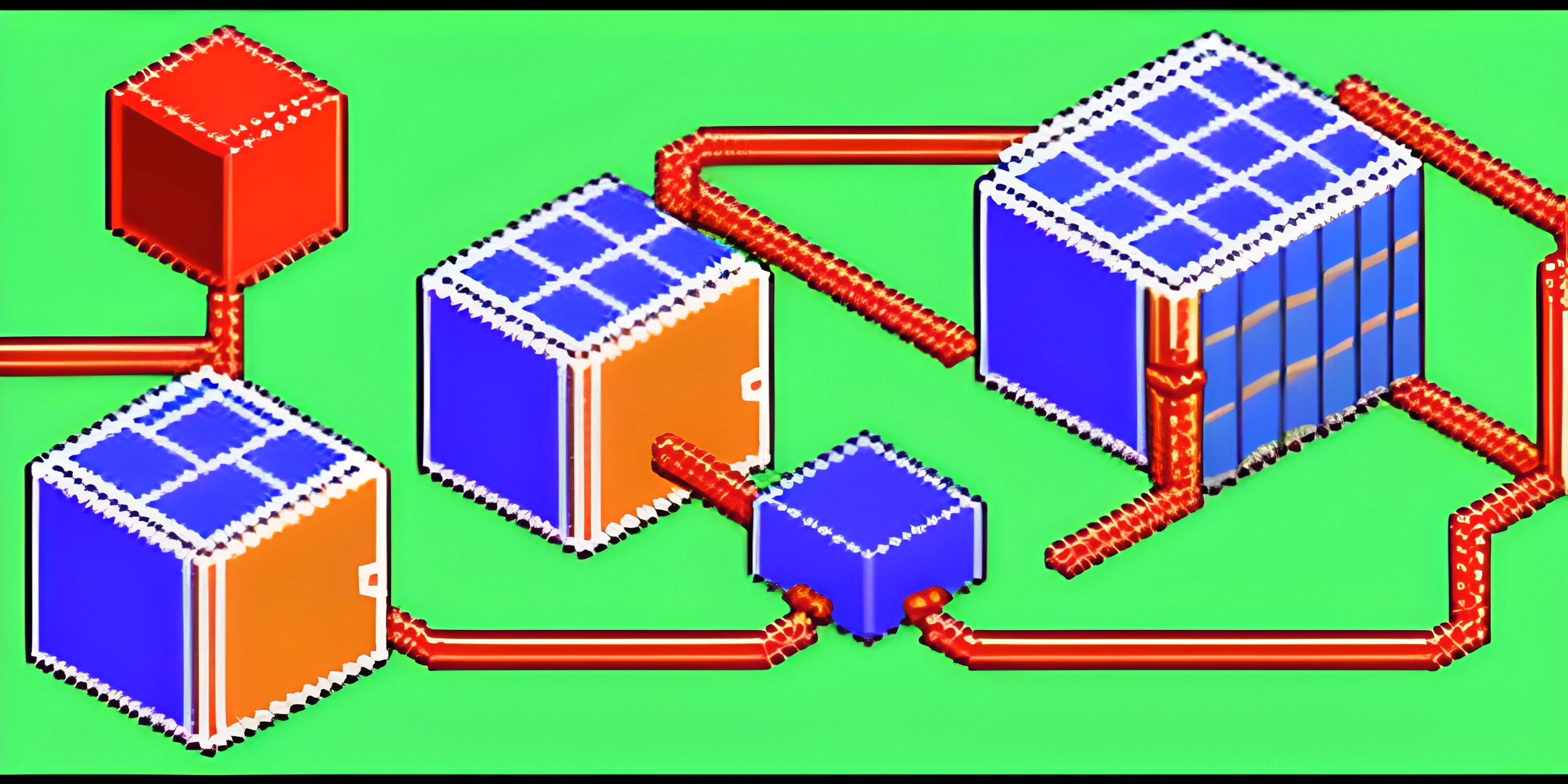
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Express middleware are functions that have access to the request object (req), the response object (res), and the next middleware function in the application’s request-response cycle. Middleware can execute any code, make changes to the request and response objects, and end the request-response cycle, or call the next middleware function. Middleware functions are essential for creating flexible and modular routing in a Node.js application using Express.
Using Built-In Express Middleware
Express provides various built-in middleware functions, such as express.json()
and express.urlencoded()
, which are used for parsing incoming request bodies.
To use built-in middleware, you need to call the app.use()
function and pass the middleware function as an argument. For example, to use the express.json()
middleware, you would do the following:
const express = require("express"); const app = express(); app.use(express.json()); // Your routes go here app.listen(3000, () => { console.log("Server is running on port 3000"); });
Creating Custom Middleware
You can also create custom middleware functions to perform specific tasks. To create a custom middleware, define a function with three parameters: req
, res
, and next
. The next
parameter is a function that tells Express to move on to the next middleware function in the stack.
Here's an example of a custom middleware function that logs the request method and URL:
function logRequest(req, res, next) { console.log(`${req.method} ${req.url}`); next(); }
To use this custom middleware, pass it as an argument to the app.use()
function:
app.use(logRequest);
Using Middleware for Specific Routes
You can also apply middleware functions to specific routes by passing them as arguments before the route's handler function. For example, if you have a middleware function authMiddleware
that checks if a user is authenticated, you can apply it to a protected route like this:
app.get("/protected", authMiddleware, (req, res) => { res.send("Welcome to the protected route!"); });
In this example, the authMiddleware
function will be executed before the route handler function, and if the user is authenticated, the next()
function will be called to proceed to the route handler.
By using and creating Express middleware, you can create flexible and modular routing in your Node.js applications, allowing you to organize and manage your code more effectively.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is Express middleware and why is it important?
Express middleware is a powerful feature in the Express.js framework that allows you to add functionality to the request-response cycle. Middleware functions have access to the request object (req), the response object (res), and the next middleware function in the application’s request-response cycle. They provide a flexible way to control the flow of the application, handle errors, and perform various tasks such as authentication, logging, and data processing.
How do I use built-in Express middleware in my Node.js application?
To use built-in Express middleware, you first need to install the middleware package (if it's an external package) and then require it in your application. Next, you need to use the app.use()
function to add the middleware to your application. For example, to use the body-parser
middleware for handling JSON payloads, you would do the following:
const express = require("express"); const bodyParser = require("body-parser"); const app = express(); app.use(bodyParser.json()); // Your routes and other middleware here app.listen(3000, () => { console.log("App listening on port 3000"); });
How do I create a custom Express middleware function?
Creating custom Express middleware is easy! You just need to define a function that takes three arguments: the request object (req), the response object (res), and the next function (next). Here's an example of a simple custom middleware function that logs the request method and URL:
function logRequest(req, res, next) { console.log(`${req.method} ${req.url}`); next(); }
To use your custom middleware, add it to your application using the app.use()
function:
app.use(logRequest); // Your routes and other middleware here
Can I use middleware for specific routes only?
Yes, you can! Instead of using the app.use()
function, you can apply middleware to specific routes by passing the middleware function as an argument before the route handler. For example, if you only want to use the logRequest
middleware for the /user
route, you would do:
app.get("/user", logRequest, (req, res) => { res.send("User information"); });
How do I handle errors in Express middleware?
To handle errors in Express middleware, you can create a custom error-handling middleware function with four arguments: the error (err), the request object (req), the response object (res), and the next function (next). When you pass an error to the next()
function in a middleware, Express will skip all remaining middleware functions and jump to the error-handling middleware. Here's an example of an error-handling middleware function:
function errorHandler(err, req, res, next) { console.error(err.message); res.status(500).send("Oops! Something went wrong."); }
To use the error-handling middleware, add it at the end of your middleware stack using the app.use()
function:
// Your routes and middleware here app.use(errorHandler);