Node.js Express Basic Server Setup
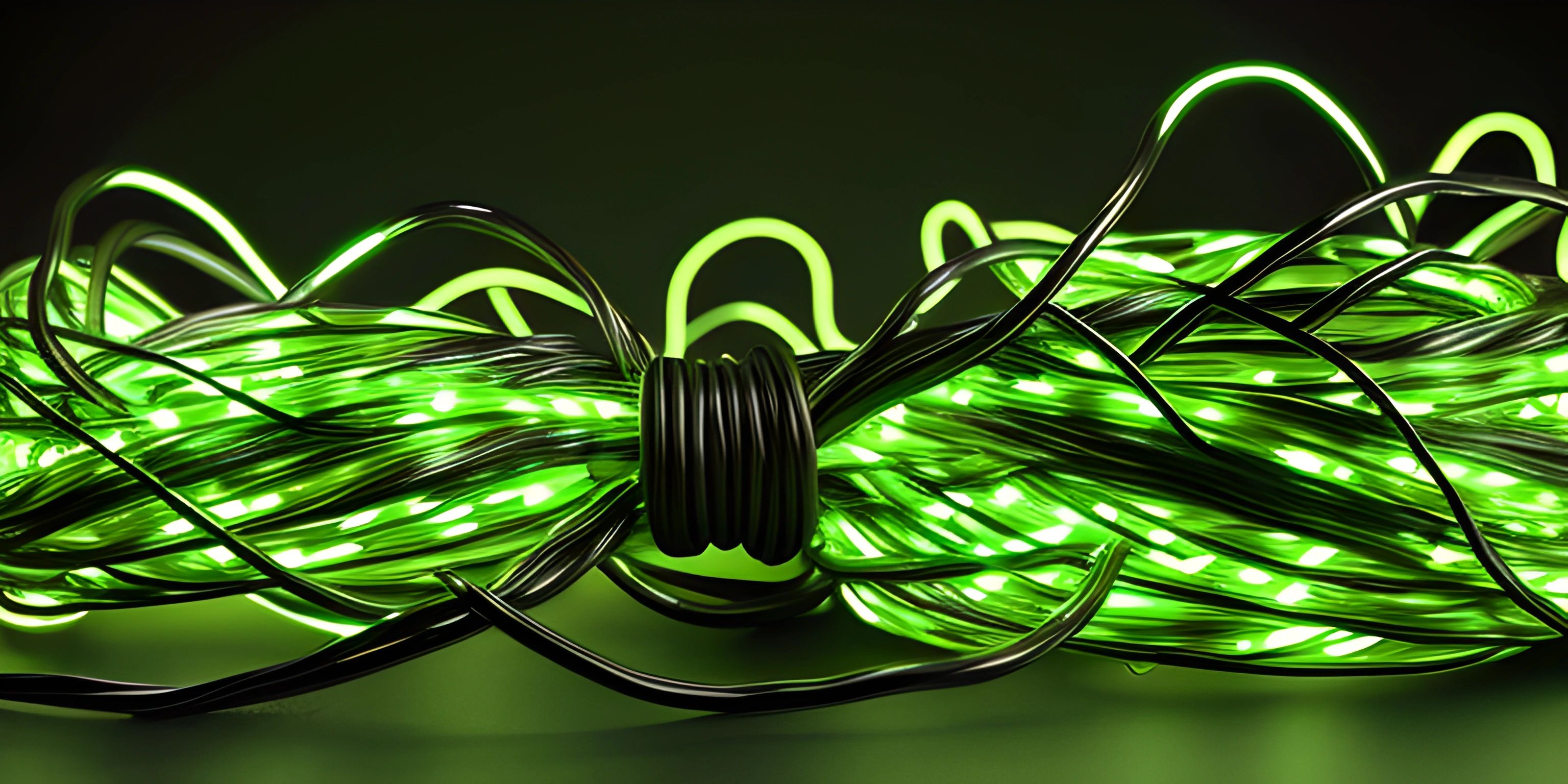
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In this article, we will learn how to create a simple web server using Node.js and Express. Express is a popular web application framework for Node.js, designed for building web applications and APIs.
Prerequisites
Before we get started, make sure you have the following installed on your system:
Step 1: Create a new project
Create a new directory for your project and navigate to it in your terminal.
mkdir nodejs-express-server cd nodejs-express-server
Initialize a new npm project by running:
npm init -y
This will create a package.json
file in your project directory.
Step 2: Install Express
Install Express using npm:
npm install express
This will add Express as a dependency in your package.json
file.
Step 3: Create the server
Create a new file named server.js
in your project directory and add the following code:
const express = require("express"); const app = express(); const port = 3000; app.get("/", (req, res) => { res.send("Hello World!"); }); app.listen(port, () => { console.log(`Server running at http://localhost:${port}`); });
Here's a breakdown of the code above:
- We import the Express module using
require
. - We create an instance of the Express app by calling
express()
. - We define a port for our server to listen on.
- We create a route for the root path ("/") that sends a "Hello World!" response.
- We start the server using
app.listen()
and log a message to the console.
Step 4: Start the server
To start the server, run the following command in your terminal:
node server.js
You should see the following output:
Server running at http://localhost:3000
Open your web browser and navigate to http://localhost:3000 to see your server in action. You should see the "Hello World!" message displayed on the page.
Congratulations! You have successfully created a simple web server using Node.js and Express. You can now build upon this foundation to create more complex web applications and APIs.
FAQ
What is Node.js and Express?
Node.js is an open-source, cross-platform runtime environment that allows you to run JavaScript on the server-side. Express is a minimalist web framework for Node.js, which makes it easy to create and manage web applications and APIs.
How do I install Node.js and Express?
First, you need to install Node.js on your system. Visit the official Node.js website (https://nodejs.org/) and download the appropriate version for your OS. After installing Node.js, you can install Express using the following command in your terminal or command prompt:
npm install express --save
How do I create a basic web server using Node.js and Express?
Once you have Node.js and Express installed, follow these steps to create a basic web server:
- Create a new folder for your project and navigate to it in your terminal or command prompt.
- Run
npm init
to create apackage.json
file and follow the prompts. - Create a new file named
app.js
in your project folder. - Write the following code inside
app.js
:const express = require("express"); const app = express(); const port = 3000; app.get("/", (req, res) => { res.send("Hello World!"); }); app.listen(port, () => { console.log(`Server running at http://localhost:${port}`); });
- Save the file and run
node app.js
in your terminal or command prompt. - Open your browser and navigate to
http://localhost:3000
. You should see "Hello World!" displayed on the page.
How can I serve static files (e.g., images, stylesheets, etc.) using Express?
To serve static files, you need to use the express.static
middleware. First, create a folder named public
in your project directory and put your static files (e.g., images, CSS files, JavaScript files, etc.) inside it. Then, add the following line of code in your app.js
file:
app.use(express.static("public"));
Now, your static files will be accessible through your server. For example, if you have an image named logo.png
in the public
folder, you can access it through the URL http://localhost:3000/logo.png
.