Hash Tables 101
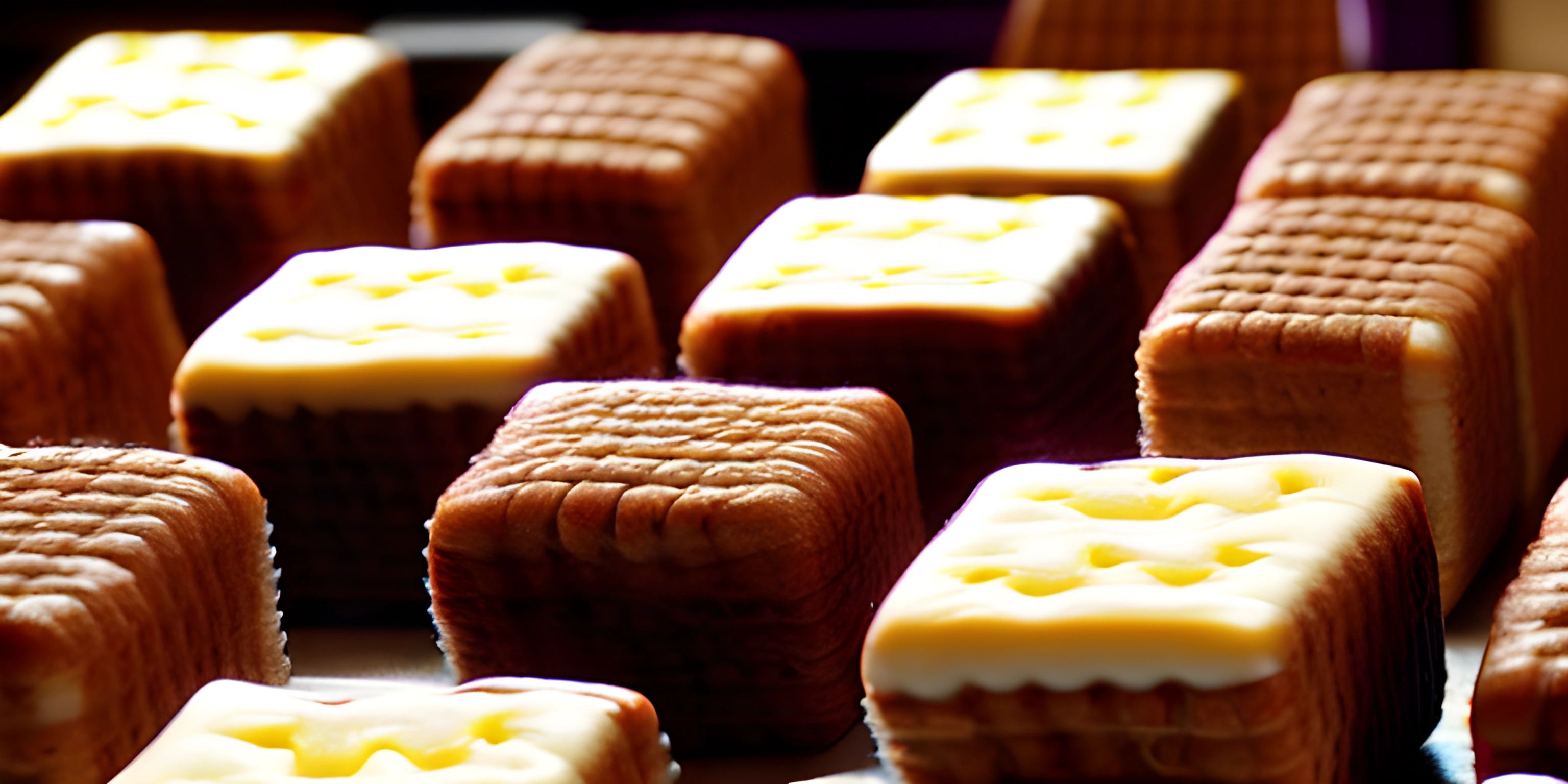
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine you have a magical filing cabinet. Instead of flipping through each folder to find what you need, you say a word, and the cabinet just hands you the correct folder. That's the essence of hash tables. They're like magical filing cabinets in the world of programming, allowing for quick access, insertion, and deletion of data.
What Is a Hash Table?
A hash table is a data structure that stores data in key-value pairs. Each piece of data (value) is associated with a unique identifier (key). The magic behind hash tables lies in the hash function, which takes a key and returns an index in an array where the value is stored. This allows for rapid data retrieval, as you can directly access the index instead of searching through all the data.
The Hash Function
The hash function is the core of the hash table. It's like the sorting hat in Harry Potter, determining the "house" (index) each key belongs to. The hash function must be deterministic, meaning it should always produce the same index for the same key. Here's a simple example in Python:
def simple_hash(key): return sum(ord(char) for char in key) % 10 print(simple_hash("apple")) # Might output: 6 print(simple_hash("banana")) # Might output: 9
In this example, the simple_hash
function converts each character in the string to its ASCII value using ord()
, sums these values, and then takes the remainder when divided by 10. The result is an index where the key-value pair will be stored in the hash table.
Handling Collisions
Collisions occur when two keys hash to the same index. Imagine our magical cabinet tries to place two folders in the same slot. There are several strategies to handle this:
- Chaining: Each slot in the array points to a list (or chain) of entries. If multiple keys hash to the same index, their values are stored in this list.
- Open Addressing: When a collision occurs, the hash table searches for the next available slot. There are different probing sequences, such as linear probing, quadratic probing, and double hashing.
Here's a basic example of chaining in Python:
class HashTable: def __init__(self): self.table = [[] for _ in range(10)] def hash_function(self, key): return sum(ord(char) for char in key) % 10 def insert(self, key, value): index = self.hash_function(key) self.table[index].append((key, value)) def get(self, key): index = self.hash_function(key) for k, v in self.table[index]: if k == key: return v return None hash_table = HashTable() hash_table.insert("apple", 5) hash_table.insert("banana", 3) print(hash_table.get("apple")) # Outputs: 5 print(hash_table.get("banana")) # Outputs: 3 print(hash_table.get("cherry")) # Outputs: None
Applications of Hash Tables
Hash tables are incredibly versatile and are used in various applications:
- Dictionaries and Maps: These are perhaps the most common usage. In languages like Python, dictionaries are implemented using hash tables.
- Database Indexing: Hash tables are used to index database records for quick retrieval.
- Caching: Hash tables can be used to store recently accessed data to minimize the time taken for future requests.
Advantages and Disadvantages
Advantages
- Fast Data Access: The average time complexity for search, insert, and delete operations is O(1).
- Simple Implementation: Hash tables are relatively easy to implement and understand.
Disadvantages
- Collision Handling: Collisions can degrade performance, and handling them can add complexity.
- Memory Usage: Hash tables may require more memory than other data structures, especially if the hash table is sparsely populated.
Choosing Hash Functions
Selecting a good hash function is crucial for the efficiency of hash tables. A good hash function should:
- Minimize Collisions: The fewer the collisions, the better the performance.
- Be Fast: Hashing should be quick to compute.
- Distribute Keys Uniformly: Keys should be spread across the array evenly.
Conclusion
Hash tables are a powerful tool in a programmer's toolkit, providing efficient data storage and retrieval. By understanding their inner workings and how to handle collisions, you can harness their full potential. Whether you're building a dictionary or a complex caching system, hash tables are there to make your life easier and your programs faster.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Data Types (psst, it's free!).
FAQ
What is a hash table?
A hash table is a data structure that stores data in key-value pairs, allowing for fast data access, insertion, and deletion.
What is a hash function?
A hash function takes a key and returns an index in an array where the associated value is stored, enabling quick data retrieval.
How do hash tables handle collisions?
Collisions can be handled using strategies like chaining (storing collided items in a list) or open addressing (finding the next available slot).
What are some common applications of hash tables?
Hash tables are used in dictionaries, database indexing, and caching systems.
What are the advantages of using hash tables?
Hash tables offer fast data access (average O(1) time complexity), simplicity, and efficiency in various applications.