Hash Tables: An Introduction
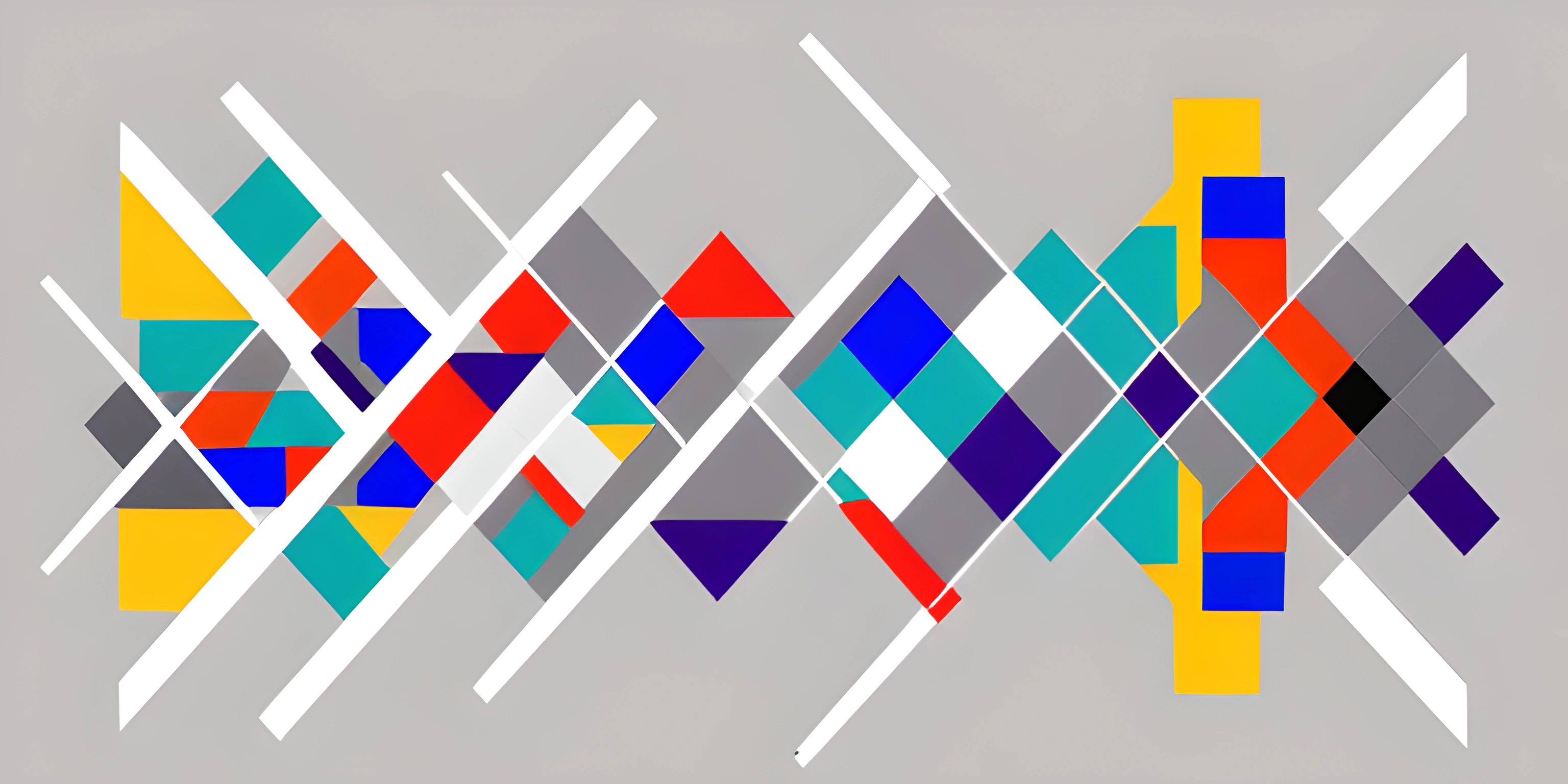
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine you're a librarian in a vast library, with millions of books. A visitor comes in and asks for a specific book. What do you do? Do you search through every single book one by one? That would be incredibly slow and inefficient. Instead, you would use a catalog system that helps you find the book quickly. Hash tables are similar to that catalog system, but for programming.
What is a Hash Table?
A hash table is a data structure that allows you to store and retrieve values based on a given key. It does this by using a hash function to convert the key into an index in an array, where the value is stored. This allows for fast and efficient data retrieval, making hash tables a popular choice for many programming tasks.
Hash Function
The hash function is the heart of a hash table. Its job is to take the key (which can be any data type) and convert it into a numeric index in the array. The ideal hash function should distribute the keys uniformly across the array, which helps minimize collisions (when two keys have the same index).
Here's a simple example of a hash function, using pseudocode:
function hash(key) { index = 0 for each character in key { index = index + character ASCII value } return index % array size }
This hash function adds up the ASCII values of the characters in the key and then takes the remainder when divided by the array size. This ensures that the index will always be within the bounds of the array.
Collisions
Collisions are when two or more keys have the same index in the array. Since each array index can only store one value, we need a way to resolve these collisions. There are several methods to do this, such as chaining (using a linked list to store multiple values at the same index) and open addressing (probing for the next available index).
Here's an example of chaining using pseudocode:
function insert(key, value) { index = hash(key) if array[index] is empty { array[index] = new linked list } array[index].add(value) }
Applications of Hash Tables
Hash tables are incredibly versatile and can be used in a wide variety of programming tasks. Some common applications include:
- Dictionaries: Hash tables can be used to implement dictionaries or maps, where you can store and retrieve values based on unique keys.
- Caching: Hash tables are often used in caching systems, where the quick retrieval of data is crucial for performance.
- Frequency counting: By using keys to represent unique items and values to store their frequency, hash tables can be used to efficiently count the occurrences of items in a data set.
In conclusion, hash tables are an essential data structure in programming, offering efficient storage and retrieval of data. By understanding the underlying concepts of hash tables, you'll be well-equipped to tackle a wide range of programming challenges.
FAQ
What is a hash table?
A hash table is a data structure that allows you to store and retrieve values based on their associated keys. It uses a hash function to convert the keys into indices, which determine the location of the associated value in the table. Hash tables are widely used in programming due to their fast lookup, insertion, and deletion capabilities.
How does a hash function work?
A hash function is responsible for converting the key into an index, which is then used to locate the value in the hash table. It takes the key as input and generates a consistent and unique output (the index) for the same key. However, multiple keys may sometimes generate the same index, resulting in a collision. Good hash functions minimize the chance of collisions while ensuring an even distribution of indices.
What is a collision in hash tables and how can it be resolved?
A collision occurs when two or more keys generate the same index through the hash function, causing a conflict when trying to store or retrieve their associated values. There are several methods to handle collisions, including:
- Chaining: Each index in the hash table points to a linked list, which can store multiple key-value pairs.
- Open Addressing: When a collision occurs, the algorithm searches for the next available slot (linear probing), or uses another probing sequence (quadratic probing or double hashing) to find an empty space.
Why are hash tables important in programming?
Hash tables are essential in programming due to their efficiency in performing operations like lookup, insertion, and deletion. They provide fast access to data, often with constant time complexity (O(1)). This makes hash tables a popular choice for various applications, such as implementing caches, symbol tables in compilers, and databases.
What are some common use cases for hash tables?
Hash tables are versatile and can be used in a wide range of applications, such as:
- Implementing caches to store temporary data for quick access.
- Creating dictionaries or maps where keys are associated with values, like a phonebook.
- Implementing sets, where you can store unique elements without duplicates.
- Building symbol tables in compilers to store variable names and their corresponding memory locations.
- Storing data in databases and providing efficient searching capabilities.