Hash Tables Explained
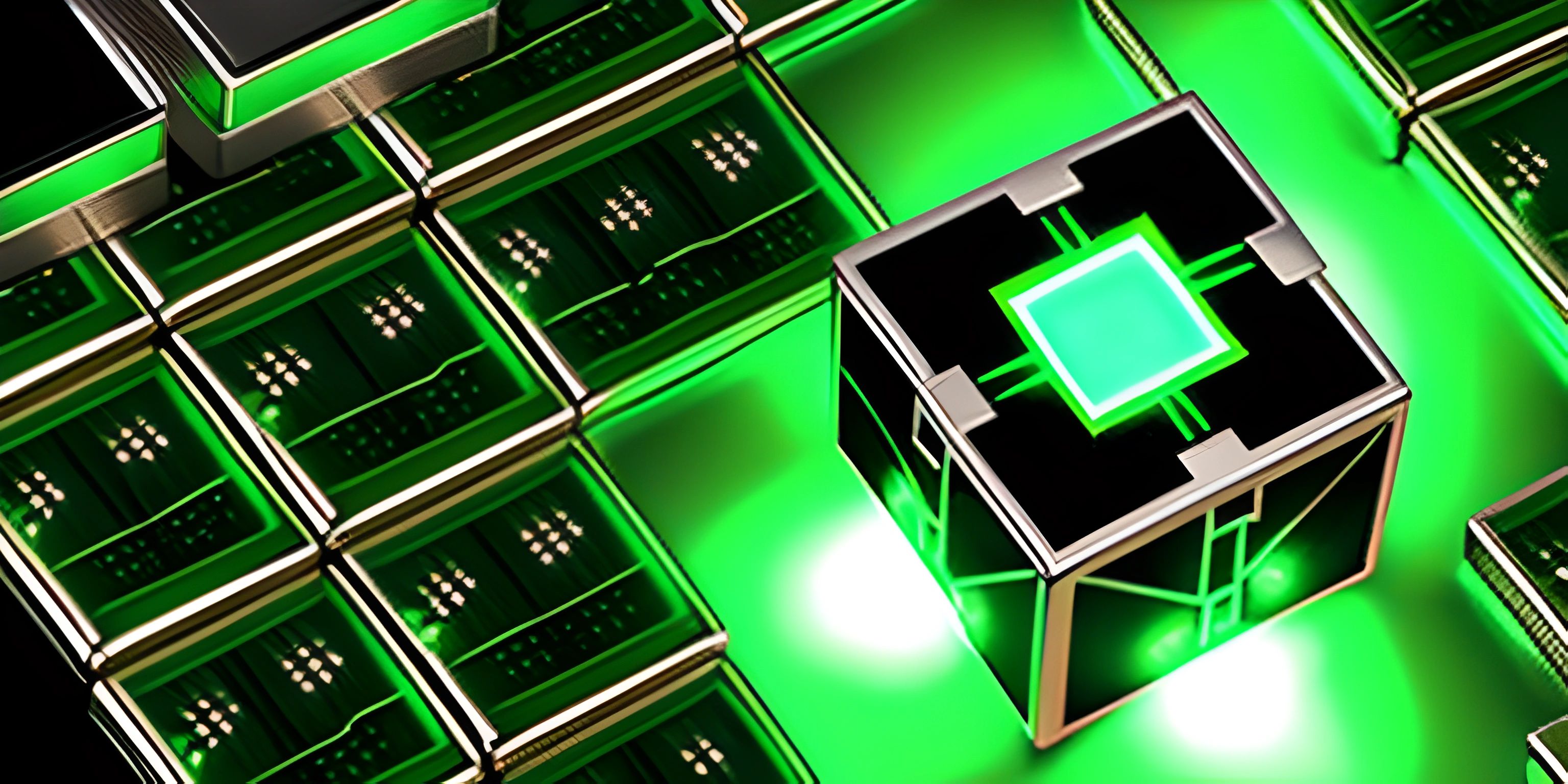
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine you're a librarian, and you have thousands of books to organize. You could place them in random shelves, but that would make finding a specific book quite challenging. Instead, you want to create a system that allows you to quickly find a book whenever someone asks for it. In computer programming, we face similar scenarios when dealing with large amounts of data, and one solution is the impressive and efficient hash table.
What is a Hash Table?
A hash table is a data structure that allows us to store and retrieve values based on a unique key. It enables constant-time access, insertion, and deletion of elements, making it a popular choice for many applications.
To understand how hash tables work, let's break down the main components:
Hash Function
At the heart of a hash table is the hash function. It takes a given key as input and produces a numeric value, known as the hash value. The hash function should be deterministic, meaning it always returns the same hash value for the same input. However, it's possible for two different keys to produce the same hash value, which is called a collision.
Buckets
The hash table uses the hash value to determine where to store the corresponding data. The data structure allocates a fixed amount of memory, divided into slots or "buckets." The hash function maps the keys to these buckets, ensuring a fairly even distribution of keys across the available memory.
Collision Resolution
As mentioned earlier, collisions can occur when two keys have the same hash value. To handle these situations, hash tables use collision resolution techniques, such as chaining or open addressing.
- Chaining involves creating a linked list at each bucket. If multiple keys map to the same bucket, they are stored in the linked list.
- Open addressing involves finding an alternative bucket when a collision occurs. This can be done using methods like linear probing, quadratic probing, or double hashing.
Operations in a Hash Table
Hash tables support basic operations like:
- Insertion: Adding a new key-value pair to the table.
- Lookup: Retrieving the value associated with a given key.
- Deletion: Removing a key-value pair from the table.
These operations can generally be performed in constant time, making hash tables incredibly efficient.
Applications of Hash Tables
Hash tables are versatile and widely used in various applications, such as:
- Programming language interpreters for symbol tables
- Database indexing for faster lookup
- Caching systems to store and retrieve data quickly
- Implementing sets and dictionaries in various programming languages
Limitations and Alternatives
While hash tables are incredibly useful and efficient, they do have some limitations:
- They require a good hash function to avoid the detrimental effects of collisions.
- They may suffer from poor performance if the table becomes too full, necessitating resizing.
- They are not suitable for scenarios where the order of elements matters, as they don't maintain the insertion order.
Despite these limitations, hash tables are an essential tool in a programmer's arsenal. In cases where a hash table might not be the best fit, alternative data structures like trees or tries can be considered.
In conclusion, hash tables are an efficient and widely-used data structure for organizing and accessing data based on unique keys. They offer constant-time operations and are utilized in various applications, making them an indispensable part of computer programming.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Using Constants (psst, it's free!).
FAQ
What is a hash table and why is it important in computer programming?
A hash table is a data structure that allows you to store and retrieve values based on a unique key. It is highly efficient in terms of time complexity, offering fast insertion, deletion, and lookup operations. In computer programming, hash tables are crucial for tasks that require quick access to data, such as search engines, databases, and caching systems.
How does a hash table work?
A hash table uses a hashing function to map a key to an index in an underlying array. When you want to store a value, the hash function calculates the index for that key, and the value is stored in the array at that index. When you need to retrieve a value, the same hashing function is used to find the index, and the value can be fetched directly from the array. If multiple keys have the same hash (a collision), they are typically stored in a linked list at the same index, and a linear search is performed to find the correct value.
What is a hashing function and what are its characteristics?
A hashing function is a function that takes a key as input and returns an integer (the hash) that represents the index where the value associated with the key should be stored in the hash table's array. A good hashing function should have the following characteristics:
- Deterministic: It should always return the same hash for the same key.
- Uniform distribution: It should distribute the keys evenly across the array to minimize collisions.
- Fast computation: It should be quick to compute for efficient operations.
How can hash table collisions be resolved?
There are multiple techniques to resolve hash table collisions, including:
- Chaining: Store the colliding elements in a linked list at the same index. When a collision occurs, traverse the list to find the correct value.
- Open addressing: When a collision occurs, find the next available slot in the array using a probing sequence (e.g., linear probing, quadratic probing, or double hashing) and store the value there.
What are some real-world applications of hash tables?
Hash tables are widely used in various real-world applications, such as:
- Databases: Storing and retrieving records based on unique keys.
- Search engines: Implementing inverted indexes to map keywords to relevant documents.
- Caching: Storing and retrieving data based on unique keys for faster access.
- Symbol tables in compilers: Storing and retrieving identifiers and their associated information during compilation.