Understanding Arrays and Their Applications
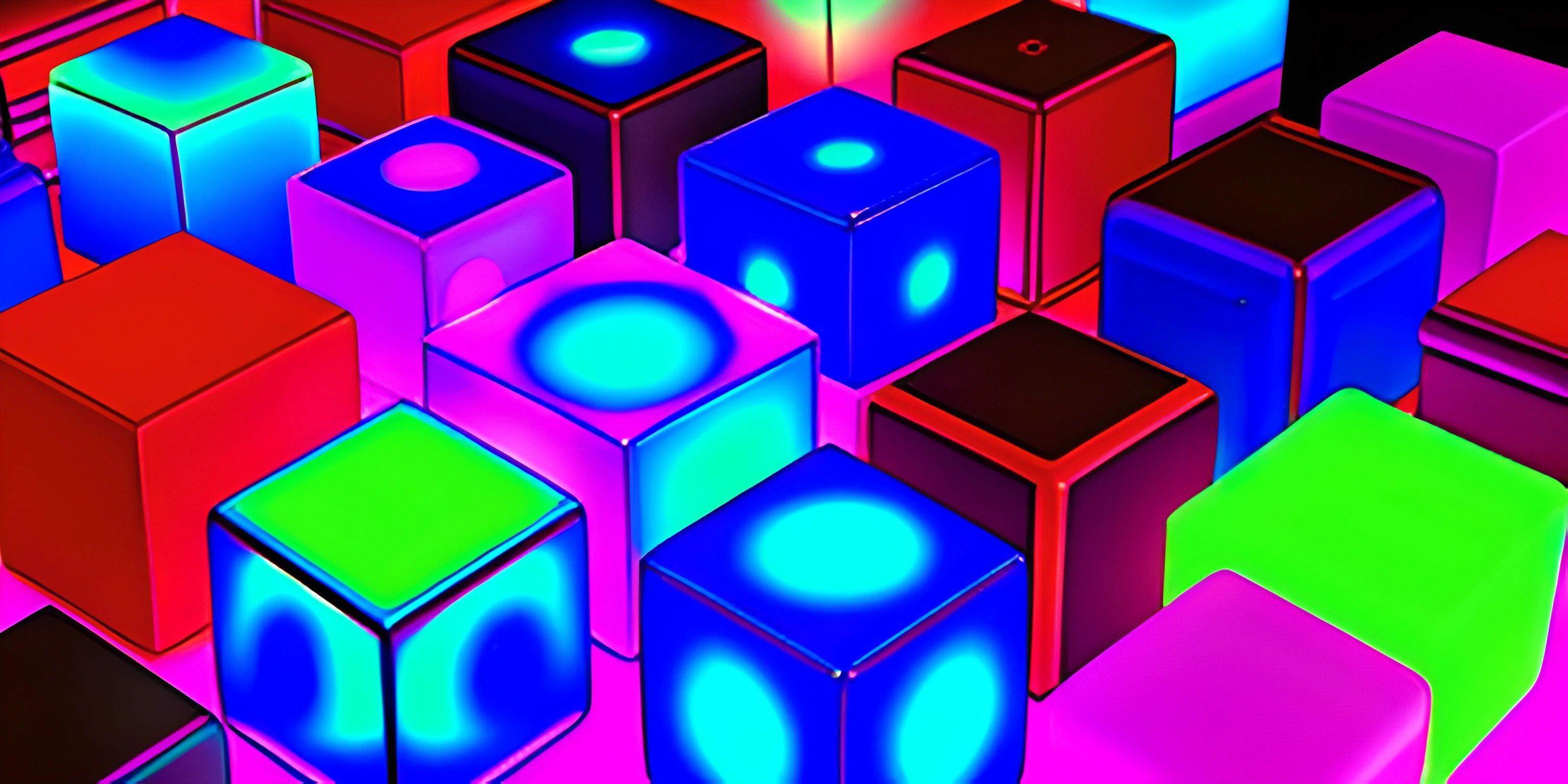
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Arrays are like the Swiss Army knives of data structures. They're versatile, efficient, and easy to use. Let's dive into the world of arrays, learn about their purpose, and see how they're used in various programming languages.
What is an Array?
An array is a collection of elements, typically of the same data type, stored in contiguous memory locations. That's a fancy way of saying it's a neat and tidy way to store multiple values in a single box. Each element in an array is identified by its index, which is a numerical value starting from zero.
Imagine you're a squirrel and you have a collection of acorns. You could scatter them around and bury them individually, or you could store them all neatly in a single wooden box with compartments. Each compartment holds an acorn, and you can easily track them down by their position in the box. That's essentially what an array does for your data!
Creating an Array
In most programming languages, creating an array is simple. Here's an example using Python:
acorns = [3, 7, 12, 4, 9]
This creates an array called acorns
with five integer values. The first value is at index 0, the second at index 1, and so on.
Accessing Elements in an Array
To access an element in an array, simply refer to its index. Here's how to grab the first acorn in our Python array:
first_acorn = acorns[0] # This gives us the value 3
Remember, the index starts at 0, not 1. So the last element in our acorns
array would be accessed like this:
last_acorn = acorns[4] # This gives us the value 9
Modifying Elements in an Array
Changing the value of an element in an array is as simple as accessing it. Let's say we want to add 2 to the third acorn in our Python array:
acorns[2] = acorns[2] + 2 # This changes the value at index 2 from 12 to 14
Looping Through an Array
Now that we know how to access and modify elements in an array, we can use loops to iterate through them. Here's an example using a for loop in Python:
for acorn in acorns: print(acorn)
This will print out:
3 7 12 4 9
Applications of Arrays
Arrays are useful in a wide range of applications. Here are a few examples:
- Storing data: Arrays can store sequences of data, such as scores on a scoreboard or temperatures throughout the day.
- Sorting and searching: Arrays can be sorted and searched, making it easy to find specific elements or sort them in a particular order.
- Matrix representation: Arrays can represent two-dimensional data structures like matrices, which are useful in math and graphics programming.
In conclusion, arrays are a fundamental data structure that will help you organize and manage your data efficiently. Mastering arrays will give you a strong foundation for tackling more complex data structures and programming tasks. So go ahead, channel your inner squirrel, and start organizing your acorns (data) with arrays!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).
FAQ
What is an array in programming languages?
An array is a data structure used in programming languages to store and organize a collection of elements, usually of the same data type. Arrays are indexed, meaning each element has a unique integer index that allows for easy access and manipulation. Arrays are widely used in various programming languages like JavaScript, Python, and Java.
How do I create an array in different programming languages?
Here are examples of creating an array in different programming languages:
// JavaScript let myArray = ["apple", "banana", "cherry"];
# Python my_array = ["apple", "banana", "cherry"]
// Java String[] myArray = {"apple", "banana", "cherry"};
Can I store different data types in a single array?
In most programming languages, arrays are designed to store elements of the same data type. However, some languages, like JavaScript and Python, allow you to store elements of different data types in a single array. For example:
// JavaScript let mixedArray = [1, "hello", true, 3.14];
# Python mixed_array = [1, "hello", True, 3.14]
In strongly-typed languages like Java, you would need to use a different data structure, such as an ArrayList<Object>
, to store mixed data types.
How do I access and modify elements in an array?
You can access and modify elements in an array using their index. The index is usually a non-negative integer that represents the position of the element in the array. Keep in mind that most programming languages use zero-based indexing, which means the first element is at index 0. Here's an example in JavaScript:
// Access the first element in the array let firstElement = myArray[0]; // Returns "apple" // Modify the second element in the array myArray[1] = "grape"; // Changes the second element from "banana" to "grape"
How do I find the length of an array in different programming languages?
The method to find the length of an array varies depending on the programming language. Here are some examples:
// JavaScript let arrayLength = myArray.length; // Returns the length of the array
# Python array_length = len(my_array) # Returns the length of the array
// Java int arrayLength = myArray.length; // Returns the length of the array