Quicksort Demystified
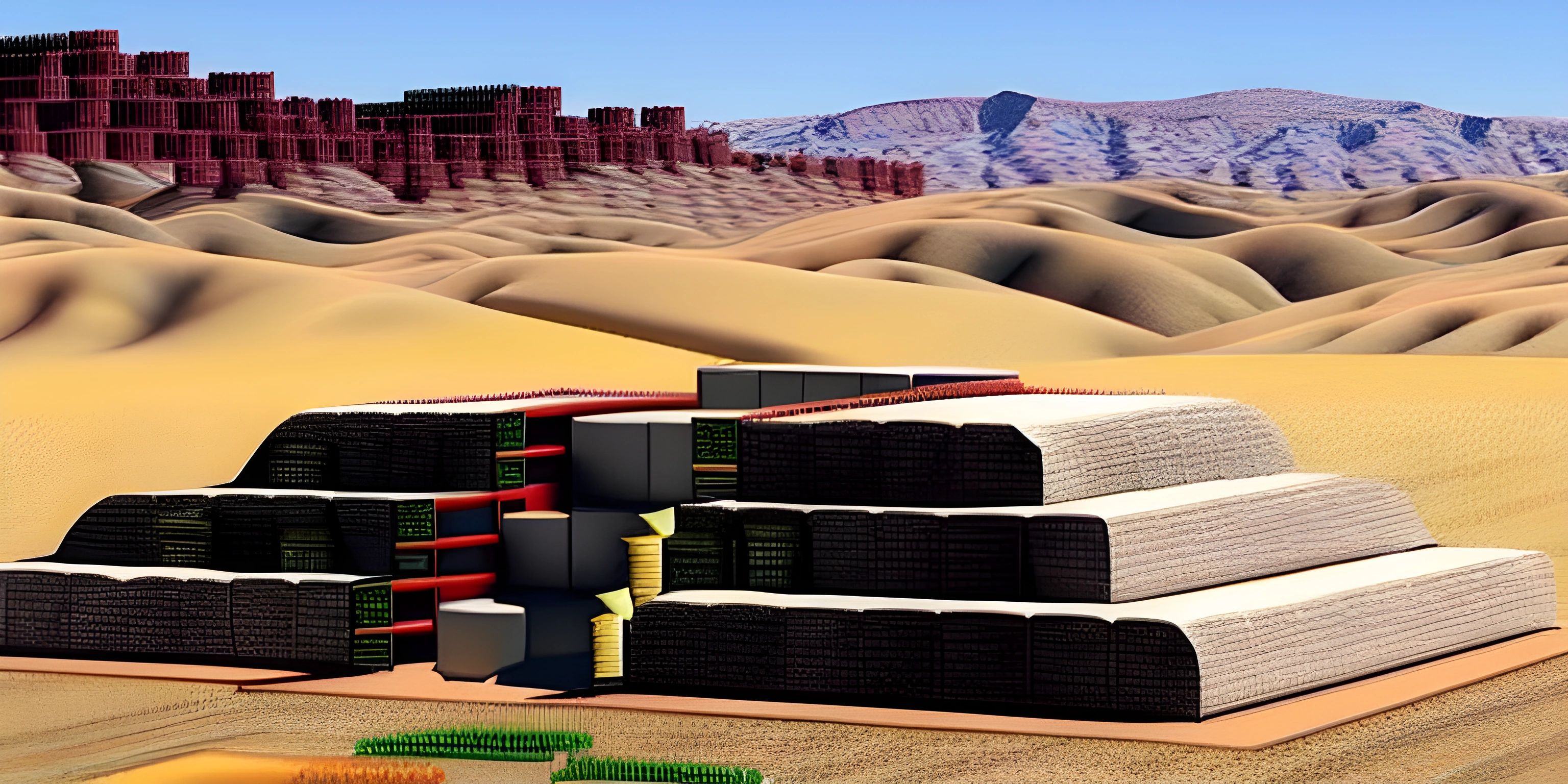
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Quicksort is like the superhero of sorting algorithms. Imagine you have a huge pile of unsorted comic books, and you want to arrange them in alphabetical order. Quicksort swoops in with its cape and lightning-fast speed to get the job done. It’s not just any ordinary sorting algorithm; it’s one of the most efficient ones out there, and it does it all with style and grace.
The Magic Behind Quicksort
Quicksort uses a divide-and-conquer strategy to sort elements. The basic idea is to pick a 'pivot' element from the array and partition the other elements into two sub-arrays according to whether they are less than or greater than the pivot. The sub-arrays are then sorted recursively. This might sound confusing, but let’s break it down step by step.
Choosing the Pivot
The choice of the pivot element is crucial for the efficiency of the algorithm. A poorly chosen pivot can degrade Quicksort to O(n^2) complexity, while a well-chosen pivot generally yields O(n log n) complexity. Common strategies for choosing the pivot include:
- Picking the first element.
- Picking the last element.
- Picking a random element.
- Picking the median element.
Partitioning the Array
Once the pivot is chosen, the next step is partitioning the array. This involves rearranging the elements so that all elements less than the pivot come before it, and all elements greater than the pivot come after it. Here’s a quick example:
def partition(arr, low, high): i = low - 1 pivot = arr[high] for j in range(low, high): if arr[j] <= pivot: i += 1 arr[i], arr[j] = arr[j], arr[i] arr[i + 1], arr[high] = arr[high], arr[i + 1] return i + 1
In this implementation, we choose the last element as the pivot and reorder the array based on that pivot.
Recursively Sorting Sub-arrays
The final step is to recursively apply the same process to the sub-arrays formed by partitioning. Here's the complete Quicksort function:
def quicksort(arr, low, high): if low < high: pi = partition(arr, low, high) quicksort(arr, low, pi - 1) quicksort(arr, pi + 1, high) # Example usage arr = [10, 7, 8, 9, 1, 5] n = len(arr) quicksort(arr, 0, n - 1) print("Sorted array is:", arr)
This function will sort the array in-place, meaning it doesn’t require additional memory to hold copies of the array, making Quicksort memory-efficient as well.
Applications of Quicksort
Quicksort is widely used in various applications due to its efficiency and simplicity. Here are some common uses:
Database Systems
In databases, sorting is a fundamental operation. Quicksort’s speed makes it the algorithm of choice for sorting records.
Search Algorithms
Quicksort is often used in conjunction with search algorithms. For instance, sorting data before performing binary searches can significantly speed up the search process.
Data Analysis
In data analysis, sorting data is a frequent task. Quicksort’s ability to handle large datasets efficiently makes it an ideal choice for data scientists.
Networking
In networking, Quicksort can be used to sort packets of data, ensuring that they are processed in the correct order.
Libraries and Frameworks
Many programming libraries and frameworks use Quicksort as their default sorting algorithm because of its performance and reliability.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).
FAQ
How does Quicksort compare to other sorting algorithms?
Quicksort is generally faster than other O(n log n) algorithms like Merge Sort, especially for large datasets. However, its worst-case performance is O(n^2), which can happen with poorly chosen pivots. In practice, this is rare, and optimizations like randomized pivots can mitigate this risk.
Can Quicksort be used for all types of data?
Quicksort works well for numerical and alphabetical data. However, for data with complex structures, the pivot selection and partitioning logic might need adjustments to handle the specific characteristics of the data.
Is Quicksort stable?
Quicksort is not a stable sort, meaning that equal elements might not retain their original order. If stability is essential, other algorithms like Merge Sort might be preferable.
Why is Quicksort not always the default sorting algorithm?
While Quicksort is very efficient, its O(n^2) worst-case performance is a drawback in some scenarios. Algorithms like Timsort, which combines Merge Sort and Insertion Sort, can offer more consistent performance and are used as default sorting algorithms in languages like Python.
How can I optimize Quicksort?
Optimizations for Quicksort include choosing a good pivot, using a hybrid approach with Insertion Sort for small sub-arrays, and implementing tail call optimization to reduce the stack depth in recursive calls.