Stunning Text Effects with p5.js
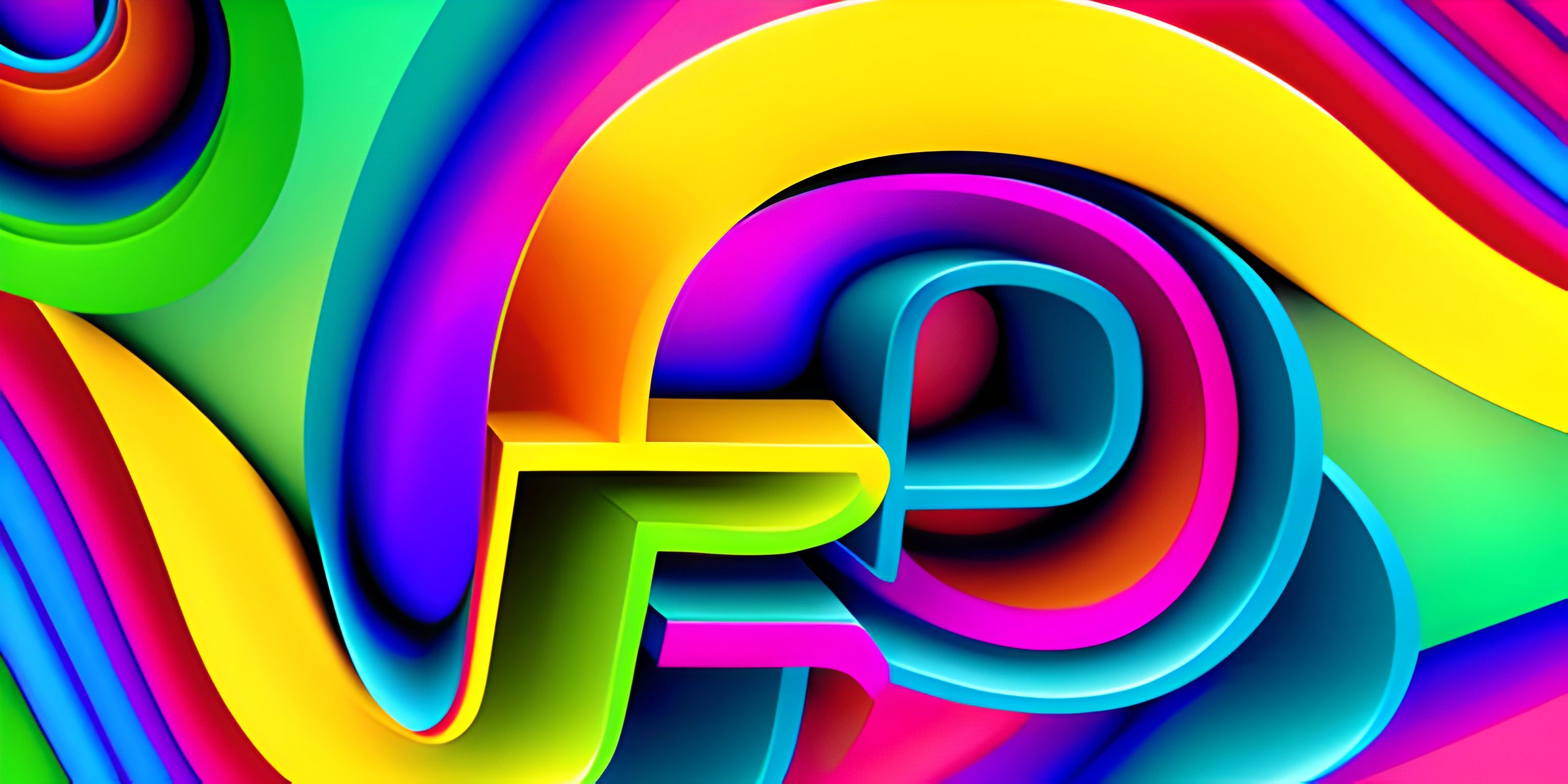
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Feast your eyes on the magic of typography with p5.js! This JavaScript library makes it incredibly simple to add delightful text effects to your creative projects. In this article, we'll explore some of the ways you can use p5.js to make your text stand out and captivate your audience.
Setting Up p5.js
Before we dive into creating text effects, let's quickly set up a p5.js project. First, head over to the p5.js website and download the latest version of the library. Next, create an HTML file and link the p5.js library in the head section:
<!DOCTYPE html> <html> <head> <script src="p5.js"></script> <script src="sketch.js"></script> </head> <body> </body> </html>
Now, create a sketch.js
file where we'll write our p5.js code. In this file, we'll define the setup
and draw
functions that p5.js will call automatically:
function setup() { createCanvas(windowWidth, windowHeight); } function draw() { // Our text effects will go here }
With our p5.js project set up, let's move on to creating some stunning text effects!
Basic Text Rendering
To render text with p5.js, we use the text
function. It takes three arguments: the text string, the x-coordinate, and the y-coordinate. For example:
function draw() { background(255); text("Hello, world!", 50, 50); }
However, this plain text doesn't look very exciting. Let's add some style!
Styling Text
To change the appearance of our text, we can use various p5.js functions such as textSize
, textFont
, fill
, and stroke
. Here's an example of a more stylish "Hello, world!" message:
function draw() { background(255); textSize(36); textFont("Arial"); fill(200, 0, 0); noStroke(); text("Hello, world!", 50, 50); }
Now we have a larger, red "Hello, world!" message in the Arial font. But we can do more! Let's move on to some animated text effects.
Animated Text Effects
Wavy Text
One way to make your text stand out is by giving it a wavy animation. To achieve this, we can use the sin
function and adjust the y-coordinate of each character:
function draw() { background(255); textSize(36); textFont("Arial"); fill(200, 0, 0); noStroke(); let message = "Wavy text!"; let spacing = 36; for (let i = 0; i < message.length; i++) { let x = 50 + i * spacing; let y = 50 + sin(frameCount * 0.1 + i) * 20; text(message.charAt(i), x, y); } }
In this example, we loop through each character of the "Wavy text!" message, and adjust the y-coordinate using the sin
function based on the current frame count and character index.
Rainbow Text
Another eye-catching effect is changing the color of each character in the text. We can use the color
function and the lerpColor
function to create a gradient of colors across the text:
function draw() { background(255); textSize(36); textFont("Arial"); noStroke(); let message = "Rainbow text!"; let spacing = 36; let startColor = color(255, 0, 0); let endColor = color(0, 255, 255); for (let i = 0; i < message.length; i++) { let x = 50 + i * spacing; let y = 50; let t = i / (message.length - 1); let currentColor = lerpColor(startColor, endColor, t); fill(currentColor); text(message.charAt(i), x, y); } }
In this code, we loop through each character of the "Rainbow text!" message and interpolate between two colors using the lerpColor
function, creating a gradient effect.
By combining and tweaking these techniques, you can create countless fascinating text effects in your p5.js projects. The only limit is your imagination! So go ahead, experiment with different styles, and make your text shine!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making Websites (psst, it's free!).