If Statements
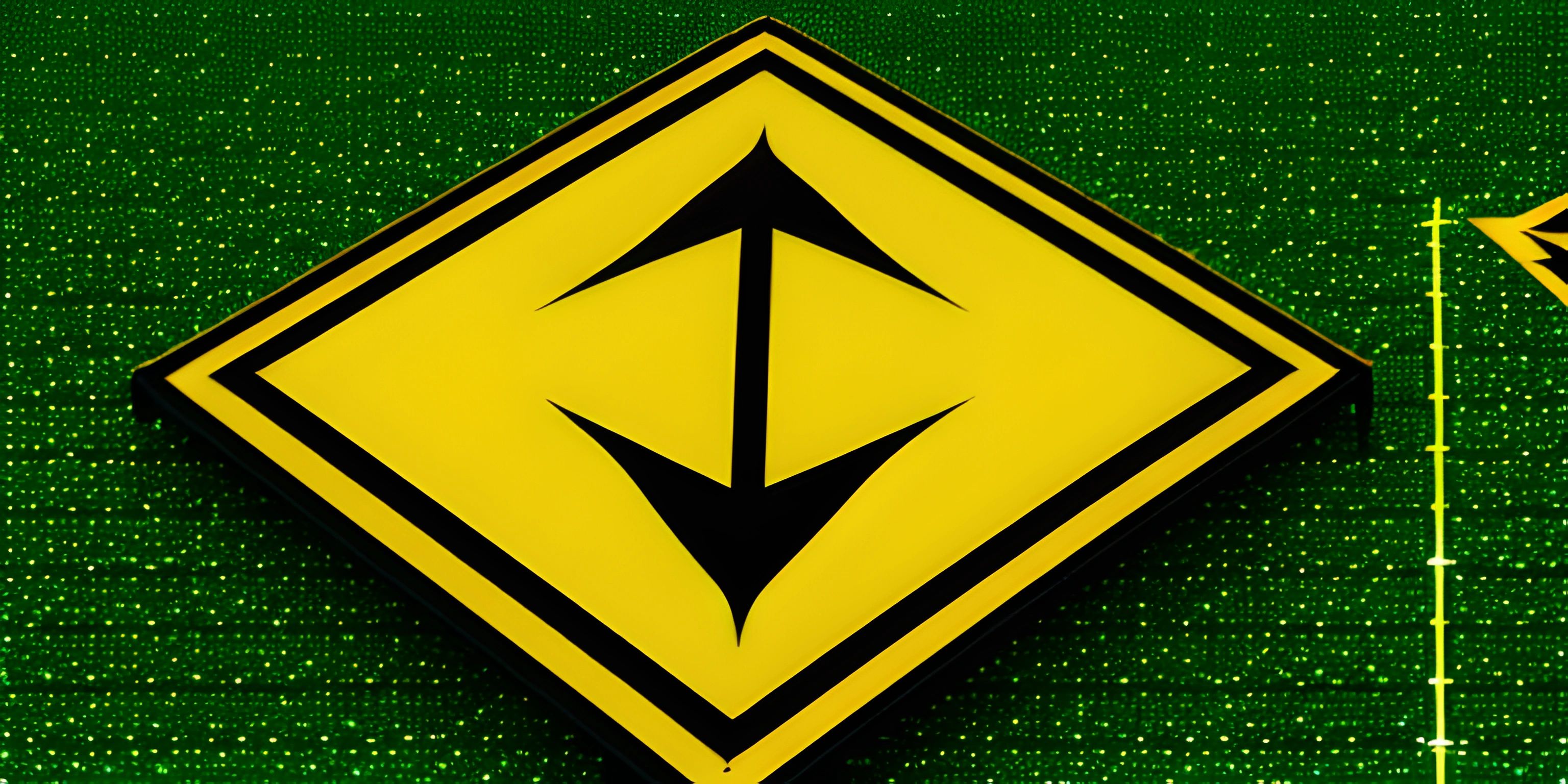
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine you're a robot chef and your job is to make peanut butter sandwiches. But what if someone is allergic to peanuts? You'd want to make sure you only make the sandwich if it's safe. In programming, we use if statements to help us make these kinds of decisions.
If Statements: The Basics
An if statement is a way to tell your program to perform certain actions only if a certain condition is met. If the condition is true, the code inside the if statement is executed. If the condition is false, the code is skipped.
Here's a simple example using Python:
temperature = 75 if temperature > 70: print("It's warm outside!")
In this example, the program checks if the temperature is greater than 70. If it is, it prints "It's warm outside!" If the temperature is less than or equal to 70, nothing happens.
Adding an Else
Sometimes, we want to do something different if the condition is not met. We can use an else statement to achieve this. Continuing with our temperature example:
if temperature > 70: print("It's warm outside!") else: print("It's not so warm outside.")
Now, if the temperature is less than or equal to 70, the program will print "It's not so warm outside."
Else If: Testing Multiple Conditions
Let's say we want to add more temperature ranges to our program. We can use an else if statement, which is written as elif
in Python. This allows us to check for multiple conditions. Here's what it might look like:
if temperature > 80: print("It's hot outside!") elif temperature > 70: print("It's warm outside!") else: print("It's not so warm outside.")
With this code, if the temperature is greater than 80, it will print "It's hot outside!" If it's between 71 and 80, it will print "It's warm outside!" And if it's 70 or below, it will print "It's not so warm outside."
Nested If Statements
Sometimes, we need to test conditions within conditions. In these cases, we can use nested if statements. Suppose we want to make sure our robot chef only makes a peanut butter sandwich for those who are not allergic to peanuts and who actually want one:
is_allergic_to_peanuts = False wants_peanut_butter = True if not is_allergic_to_peanuts: if wants_peanut_butter: print("Making a peanut butter sandwich!") else: print("Making a different sandwich.") else: print("Sorry, can't make a peanut butter sandwich.")
Our robot chef will only make a peanut butter sandwich if the person is not allergic to peanuts and if they actually want one. Otherwise, it will either make a different sandwich or inform them it's not possible to make a peanut butter sandwich.
That's the basics of if statements! They're an essential tool for controlling the flow of your code and making decisions based on conditions. Now go forth and make more informed, peanut butter-friendly programs!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making Things Stop (psst, it's free!).
FAQ
What is an if statement and why is it important in programming?
An if statement is a fundamental concept in programming that allows you to make decisions in your code based on whether a specific condition is true or false. It helps you create dynamic and responsive programs that can adapt to different situations, inputs, or data.
How do I write a basic if statement in Python and JavaScript?
Here's a simple example of an if statement in both Python and JavaScript: Python:
age = 18 if age >= 18: print("You are an adult.")
JavaScript:
let age = 18; if (age >= 18) { console.log("You are an adult."); }
In both examples, the if statement checks if the value of age
is greater than or equal to 18. If the condition is true, it executes the code inside the if block (printing the message "You are an adult.").
Can I use multiple conditions in an if statement?
Yes, you can use multiple conditions in an if statement by using logical operators such as AND (&&
in JavaScript, and
in Python) and OR (||
in JavaScript, or
in Python). Here's an example in both languages:
Python:
age = 25 country = "USA" if age >= 21 and country == "USA": print("You can legally drink alcohol.")
JavaScript:
let age = 25; let country = "USA"; if (age >= 21 && country === "USA") { console.log("You can legally drink alcohol."); }
In these examples, the if statement checks if the age
is greater than or equal to 21 and if the country
is "USA". If both conditions are true, it prints the message "You can legally drink alcohol."
How can I add an alternative action when an if statement's condition is false?
You can use an "else" block to specify code that should be executed when the if statement's condition is false. Here's an example in Python and JavaScript: Python:
temperature = 10 if temperature > 20: print("It's warm outside.") else: print("It's cold outside.")
JavaScript:
let temperature = 10; if (temperature > 20) { console.log("It's warm outside."); } else { console.log("It's cold outside."); }
In these examples, the if statement checks if the temperature
is greater than 20. If true, it prints "It's warm outside." If the condition is false, the else block executes, and it prints "It's cold outside."
How can I check multiple conditions in a single if statement using "else if"?
You can use "else if" (Python uses elif
) to check multiple conditions in a single if statement. Here's an example in Python and JavaScript:
Python:
score = 85 if score >= 90: print("You got an A.") elif score >= 80: print("You got a B.") else: print("You scored below a B.")
JavaScript:
let score = 85; if (score >= 90) { console.log("You got an A."); } else if (score >= 80) { console.log("You got a B."); } else { console.log("You scored below a B."); }
In these examples, the if statement checks if the score
is greater than or equal to 90. If true, it prints "You got an A." If the first condition is false, the else if block checks if the score is greater than or equal to 80. If this second condition is true, it prints "You got a B." If neither condition is true, the else block executes and prints "You scored below a B."