Java Inheritance Basics
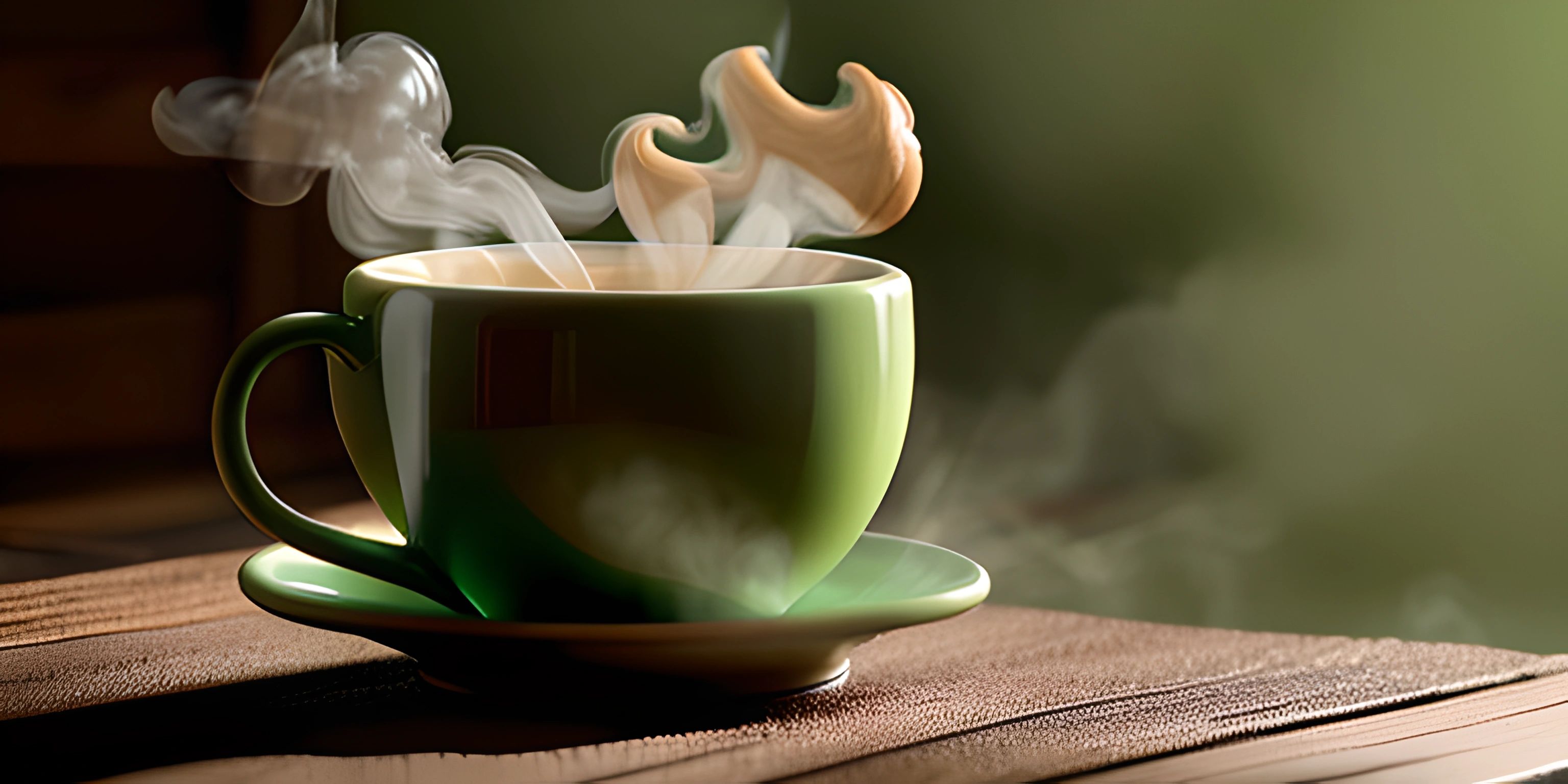
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Inheritance is a cornerstone of object-oriented programming in Java. It allows us to build complex systems by reusing, extending, and modifying existing code. So, strap in and let's explore this fantastic superpower of Java!
What is Inheritance
In a nutshell, inheritance is a mechanism in Java that allows one class to inherit or adopt properties and methods from another class. Think of inheritance like a family tree, where a child can inherit traits from their parent, like eye color or height.
In Java, the class that gets inherited is called the superclass or parent class, while the class that inherits is called the subclass or child class. The subclass can access and use the properties and methods of the superclass, and even override or extend them.
The extends
Keyword
In Java, if you want a class to inherit from another class, you use the extends
keyword followed by the name of the superclass:
class Superclass { // properties and methods } class Subclass extends Superclass { // additional properties and methods }
Now that we know the basics of what inheritance is, let's dive deeper into some examples.
Inheritance in Action
Let's say we're building a zoo management system, and we need to represent different animals. All animals share some common traits, like a name and an age. We can create a superclass Animal
with these shared properties:
class Animal { String name; int age; void makeSound() { System.out.println("The animal makes a sound"); } }
Now, let's create a Dog
subclass that inherits from Animal
:
class Dog extends Animal { void bark() { System.out.println("The dog barks"); } }
In this example, Dog
is the subclass, and Animal
is the superclass. Since the Dog
class extends Animal
, it inherits the properties and methods of Animal
.
We can now create a new Dog
object and access its properties and methods, including those inherited from Animal
:
class Main { public static void main(String[] args) { Dog myDog = new Dog(); myDog.name = "Buddy"; myDog.age = 3; myDog.makeSound(); // Output: The animal makes a sound myDog.bark(); // Output: The dog barks } }
Method Overriding
Sometimes, the inherited method from the superclass might not be suitable for the subclass. In such cases, you can override the method in the subclass by defining a new method with the same name, return type, and parameters. The subclass's version of the method will be executed instead of the superclass's version.
Let's override the makeSound()
method in our Dog
class:
class Dog extends Animal { @Override void makeSound() { System.out.println("The dog barks"); } void bark() { System.out.println("The dog barks"); } }
Now, when we call makeSound()
on a Dog
object, the output will be "The dog barks" instead of "The animal makes a sound":
class Main { public static void main(String[] args) { Dog myDog = new Dog(); myDog.name = "Buddy"; myDog.age = 3; myDog.makeSound(); // Output: The dog barks myDog.bark(); // Output: The dog barks } }
The super
Keyword
The super
keyword in Java is used to refer to the superclass of a subclass. This can be helpful in several situations, such as when you want to call a superclass's method or access its properties.
Calling a Superclass's Method
In some cases, you might want to invoke the superclass's version of an overridden method within the subclass. You can do this by using super.methodName()
:
class Dog extends Animal { @Override void makeSound() { super.makeSound(); // Call the superclass's makeSound() method System.out.println("The dog barks"); } }
Now, when we call makeSound()
on a Dog
object, both the superclass's and subclass's outputs will be displayed:
class Main { public static void main(String[] args) { Dog myDog = new Dog(); myDog.name = "Buddy"; myDog.age = 3; myDog.makeSound(); // Output: The animal makes a sound // The dog barks } }
Accessing Superclass's Properties
Similarly, you can access a superclass's properties using super.propertyName
. This can be useful when you want to modify the value of a property in the subclass without affecting the superclass's property value.
In Conclusion
Inheritance is a powerful feature in Java that promotes code reuse, modularity, and organization. By understanding how inheritance works, you can build more efficient and maintainable Java applications. So keep practicing, and remember: with great power comes great responsibility!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).