Inheritance in Programming
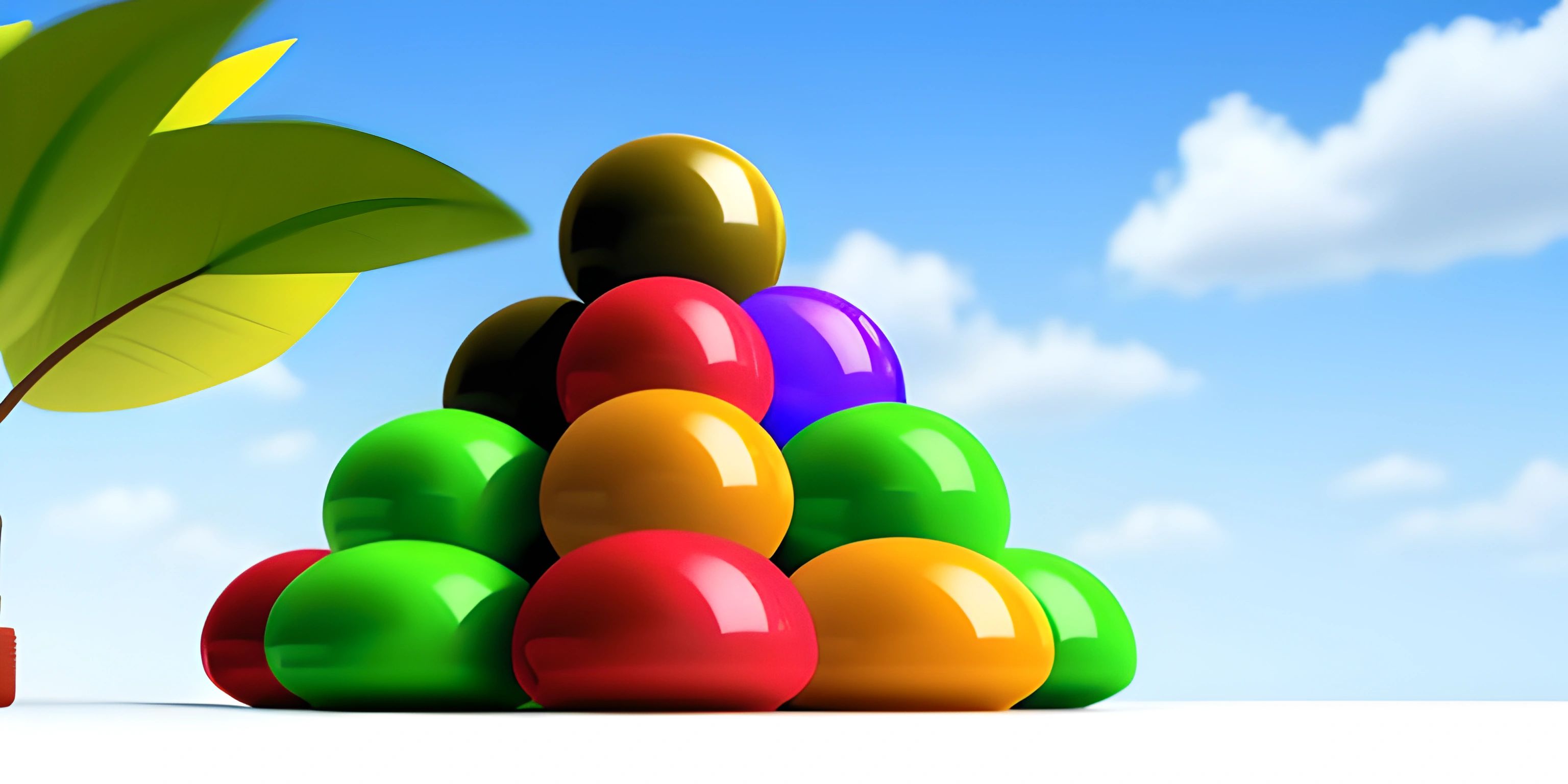
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Inheritance is a powerful concept in object-oriented programming (OOP) that allows one class to inherit the properties and methods of another. It's like a family tree in programming, where a derived class (the child) inherits traits from a base class (the parent). Ready to dive into this OOP genealogy? Let's go!
Base Class and Derived Class
Inheritance revolves around two main components: the base class (also known as the superclass or parent class) and the derived class (also known as subclass or child class). The base class is the one being inherited from, while the derived class is the one doing the inheriting.
Let's visualize this with a simple example. Imagine we're designing a game and we have a generic Character
class to start with. This class would have properties like name
, health
, and methods like attack()
and defend()
.
class Character: def __init__(self, name, health): self.name = name self.health = health def attack(self, target): # Attack logic goes here def defend(self): # Defend logic goes here
Now, suppose we want to create more specific character types like a Warrior
and a Wizard
. Instead of writing separate classes with duplicate code, we can use inheritance to create derived classes from the base Character
class.
class Warrior(Character): pass class Wizard(Character): pass
By using inheritance, both Warrior
and Wizard
classes have access to the name
, health
, attack()
, and defend()
properties and methods from the Character
class.
Overriding and Extending Methods
Inheritance doesn't mean the derived class is stuck with the exact same properties and methods as the base class. We can override or extend methods in the derived class to give them unique behaviors.
For instance, let's say we want our Warrior
class to have a unique attack method:
class Warrior(Character): def attack(self, target): # Warrior-specific attack logic goes here
We've overridden the attack()
method from the Character
class in the Warrior
class. Now, when we call attack()
on a Warrior
object, the new Warrior-specific logic will be executed.
Similarly, we can extend methods in derived classes, adding additional functionality to the base class's methods:
class Wizard(Character): def cast_spell(self, spell, target): # Spell casting logic goes here def attack(self, target): self.cast_spell("Fireball", target) super().attack(target)
In the Wizard
class, we've extended the attack()
method to include casting a spell before performing the base class's attack.
Multiple Inheritance and Mixins
Some programming languages, like Python, also support multiple inheritance, allowing a derived class to inherit from more than one base class. This can be useful for creating flexible and modular code.
For example, we could create a Flying
mixin class and have our Wizard
class inherit from both Character
and Flying
:
class Flying: def fly(self): # Flying logic goes here class Wizard(Character, Flying): pass
Now, our Wizard
class inherits properties and methods from both Character
and Flying
classes.
Conclusion
Inheritance is a fundamental concept in object-oriented programming that promotes modularity, code reusability, and a clear hierarchy between classes. By understanding the concepts of base and derived classes, overriding and extending methods, and multiple inheritance, you'll be well-equipped to wield the power of inheritance in your own programming projects.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What is inheritance in object-oriented programming?
Inheritance is a fundamental concept in object-oriented programming that allows one class to inherit properties and methods from another class. It enables code reusability, making it easier to create and maintain applications. Inheritance involves a "base class" (also known as parent class) and a "derived class" (also known as child class). The derived class inherits the characteristics of the base class, which can be extended or overridden to create new functionality.
How do base and derived classes work in inheritance?
In inheritance, the base class is the parent class that provides a set of properties and methods that can be reused or extended by the derived class. The derived class, also known as the child class, inherits these properties and methods from the base class and can add or modify them to create new functionality. This relationship between base and derived classes creates a hierarchy, making it easier to organize and maintain code.
Can a derived class inherit from multiple base classes?
Some programming languages, like Python and C++, allow a derived class to inherit from multiple base classes, a concept known as "multiple inheritance." In this case, the derived class inherits properties and methods from all the base classes. However, multiple inheritance can sometimes lead to complexities and ambiguities in the code structure. Other languages like Java and C# do not support multiple inheritance directly, but they provide alternative mechanisms like interfaces and abstract classes to achieve similar functionality.
What is method overriding in inheritance?
Method overriding is a feature in object-oriented programming that allows a derived class to provide a new implementation for a method that is already defined in its base class. This new implementation "overrides" the original method in the base class, allowing the derived class to modify or extend the behavior of that method. When a derived class object calls the overridden method, the new implementation in the derived class is executed instead of the base class version.
How is inheritance implemented in different programming languages?
The implementation of inheritance varies between programming languages, but the core concept remains the same. Here are examples of how inheritance is implemented in three popular languages:
# Python class BaseClass: def base_method(self): pass class DerivedClass(BaseClass): def derived_method(self): pass
// Java class BaseClass { void baseMethod() { // ... } } class DerivedClass extends BaseClass { void derivedMethod() { // ... } }
// C# class BaseClass { public void BaseMethod() { // ... } } class DerivedClass : BaseClass { public void DerivedMethod() { // ... } }