Java Object-Oriented Programming
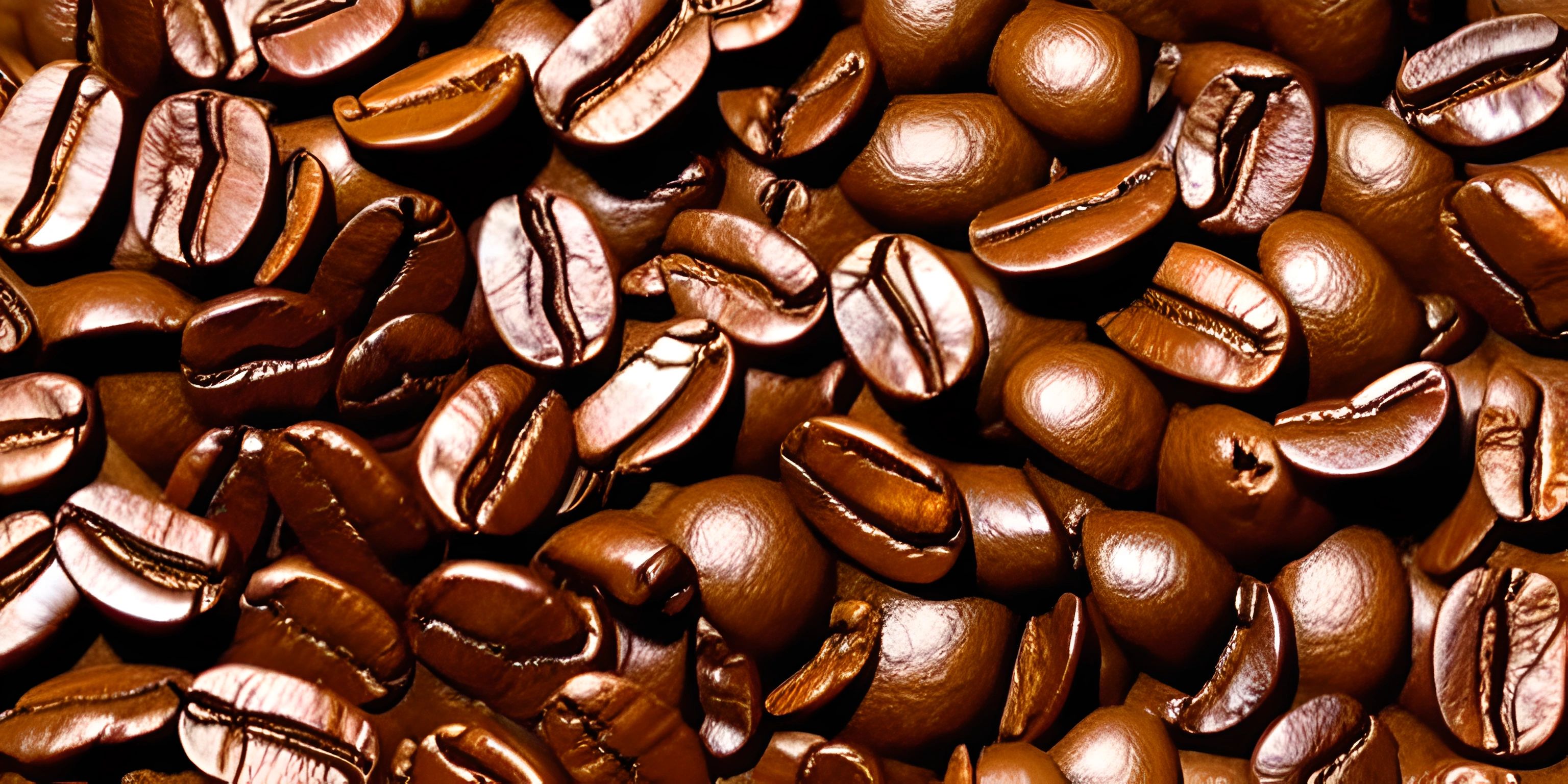
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Programming is like building with LEGO bricks - you can create an infinite variety of structures using a finite set of parts. In Java, one building block we use is called Object-Oriented Programming (OOP). OOP allows us to create reusable, modular code that represents real-world entities and their behavior. As a developer, it's crucial to understand OOP concepts in Java, like classes, objects, inheritance, encapsulation, and polymorphism. Let's dive into each of these ideas!
Classes and Objects
A class is a blueprint for creating objects (instances) with specific properties (attributes) and behaviors (methods). For example, let's say we want to represent cars in our program. We can create a class called Car
that has attributes like color
, make
, and model
, and methods like startEngine
, drive
, and stopEngine
.
class Car { String color; String make; String model; void startEngine() { // Code to start the engine } void drive() { // Code to drive the car } void stopEngine() { // Code to stop the engine } }
Now we can create objects (instances) of the Car
class, each with its own set of attributes:
Car myCar = new Car(); myCar.color = "red"; myCar.make = "Toyota"; myCar.model = "Corolla"; Car yourCar = new Car(); yourCar.color = "blue"; yourCar.make = "Honda"; yourCar.model = "Civic";
Inheritance
Inheritance allows classes to inherit properties and methods from a parent (superclass) class. This promotes code reusability and modularity. Let's say we want to represent electric cars in our program. Instead of creating an entirely new class, we can inherit from the Car
class and add electric car-specific attributes and methods. This is done using the extends
keyword.
class ElectricCar extends Car { int batteryCapacity; void chargeBattery() { // Code to charge the battery } }
Now we can create an object of the ElectricCar
class, and it will automatically have the properties and methods from the Car
class. We can also set the electric car-specific attributes:
ElectricCar myElectricCar = new ElectricCar(); myElectricCar.color = "green"; myElectricCar.make = "Tesla"; myElectricCar.model = "Model S"; myElectricCar.batteryCapacity = 100; myElectricCar.startEngine(); // Inherited from the Car class myElectricCar.chargeBattery(); // Specific to ElectricCar
Encapsulation
Encapsulation is the idea of bundling data (attributes) and methods that operate on that data within a single unit (class). It also involves restricting access to an object's data by making attributes private and providing public methods to access and modify that data. This is called data hiding and is achieved using access modifiers like private
and public
.
class Car { private String color; private String make; private String model; // Getter methods public String getColor() { return color; } public String getMake() { return make; } public String getModel() { return model; } // Setter methods public void setColor(String color) { this.color = color; } public void setMake(String make) { this.make = make; } public void setModel(String model) { this.model = model; } }
Now we can't directly access the color
, make
, and model
attributes. Instead, we use getters and setters:
Car myCar = new Car(); myCar.setColor("red"); myCar.setMake("Toyota"); myCar.setModel("Corolla"); String myCarColor = myCar.getColor(); // "red"
Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common superclass. This enables us to write more flexible and extensible code. Let's say we want to create an array of cars and electric cars. Without polymorphism, we'd need separate arrays for each type. But because ElectricCar
extends Car
, we can use an array of the Car
class to store both types:
Car[] myCars = new Car[3]; myCars[0] = new Car(); myCars[1] = new ElectricCar(); myCars[2] = new Car();
Now we can perform operations on this array, regardless of the specific car type:
for (Car car : myCars) { car.startEngine(); }
In conclusion, understanding and implementing object-oriented programming concepts in Java, such as classes, objects, inheritance, encapsulation, and polymorphism, is essential for creating efficient, reusable, and modular code. Like building with LEGO bricks, these concepts allow you to create intricate, interconnected structures from simple, reusable pieces.