Java Inheritance
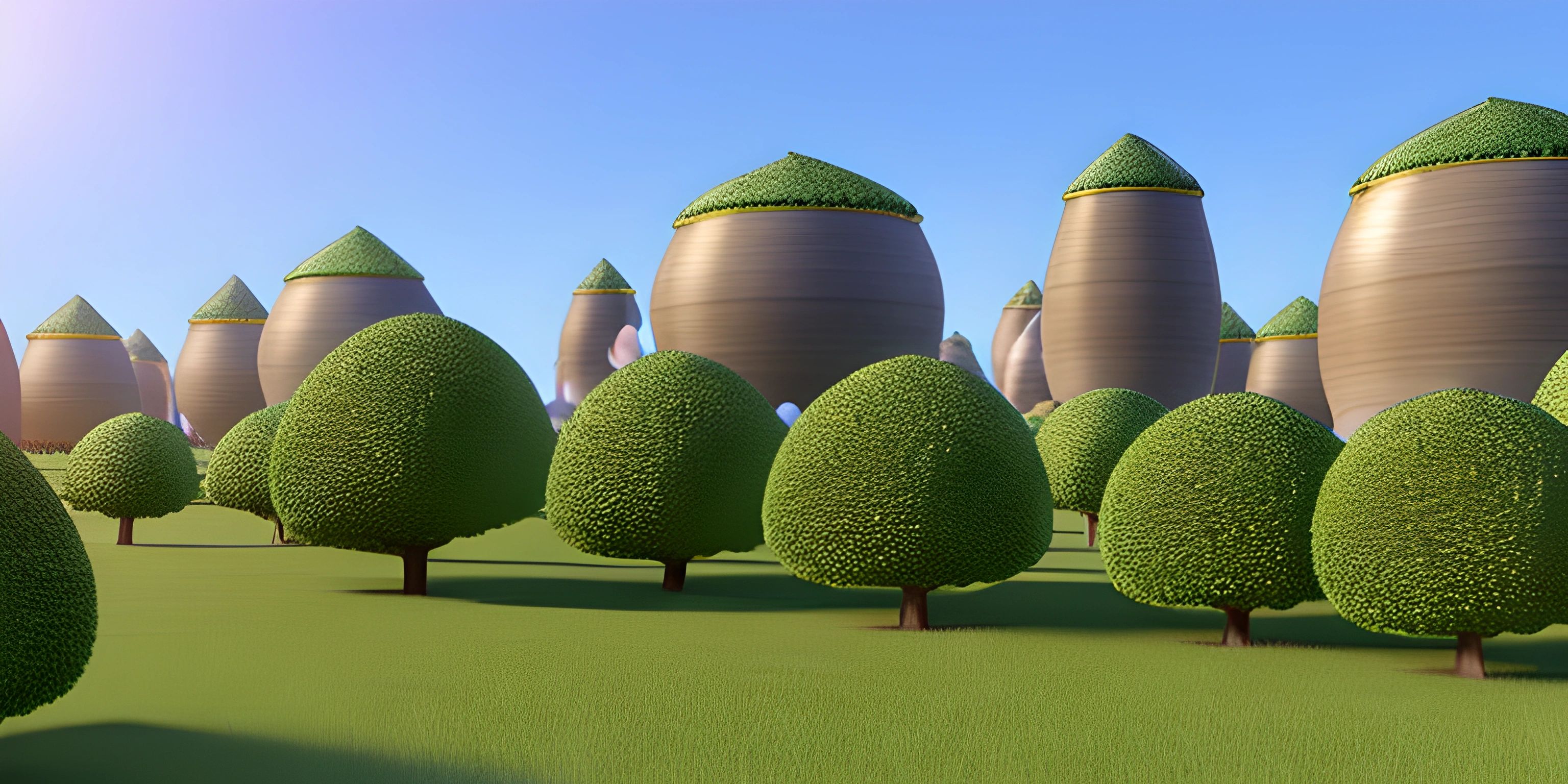
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Inheritance is a fundamental concept in object-oriented programming (OOP) languages like Java. It enables classes to inherit properties and methods from other classes, providing a way to organize and reuse code. In this journey, we'll dive into the world of inheritance in Java and discover its benefits.
Inheritance Basics
In Java, inheritance is a way for one class (called the subclass) to obtain the properties and methods of another class (called the superclass). The subclass can then extend or override the functionality provided by its superclass.
Imagine a scenario where you're designing an application to manage a zoo. You have various types of animals, such as lions, tigers, and bears. Instead of defining each animal as a separate class with duplicate code, you can create a common Animal
class (superclass) with shared properties and methods. Then, you can create subclasses for each animal type that inherits from the Animal
class.
Syntax
In Java, the extends
keyword is used to establish an inheritance relationship between classes. Here's a simplified example:
class Animal { // Properties and methods common to all animals } class Lion extends Animal { // Properties and methods specific to lions }
In this example, the Lion
class inherits the properties and methods from the Animal
class.
Benefits of Inheritance
Inheritance offers several advantages in Java programming:
Code Reusability
Inheritance allows you to reuse code from a superclass in one or more subclasses. This reduces code duplication and makes maintenance easier. If you need to make a change in the superclass, all subclasses automatically inherit the change.
Organization
Inheritance promotes a logical and hierarchical organization of your code, making it easier to understand and maintain. Classes can be grouped based on their relationships and shared characteristics.
Polymorphism
Polymorphism is a powerful OOP concept that allows objects of different classes to be treated as objects of a common superclass. Inheritance enables polymorphism by allowing you to use a superclass reference for subclass objects, making it possible to write more flexible and extensible code.
Overriding Methods
When a subclass inherits from a superclass, it can override the superclass's methods to provide its own implementation. This is done using the @Override
annotation followed by the method signature, as shown in this example:
class Animal { void makeSound() { System.out.println("The animal makes a sound"); } } class Lion extends Animal { @Override void makeSound() { System.out.println("The lion roars"); } }
In this example, the Lion
subclass overrides the makeSound
method from the Animal
superclass to provide its own custom implementation.
The super
Keyword
The super
keyword in Java is used to refer to the immediate superclass of a subclass. It can be used to call the superclass's constructor or access its methods. For example:
class Animal { void makeSound() { System.out.println("The animal makes a sound"); } } class Lion extends Animal { @Override void makeSound() { super.makeSound(); // Call the superclass method System.out.println("The lion roars"); } }
In this example, the super.makeSound()
call in the Lion
subclass invokes the makeSound
method from the Animal
superclass.
In conclusion, inheritance in Java is a powerful way to organize and reuse code, facilitating code maintenance and promoting polymorphism. By understanding and implementing inheritance in your Java projects, you'll be harnessing one of the core concepts of object-oriented programming.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).