Object-Oriented Programming Overview
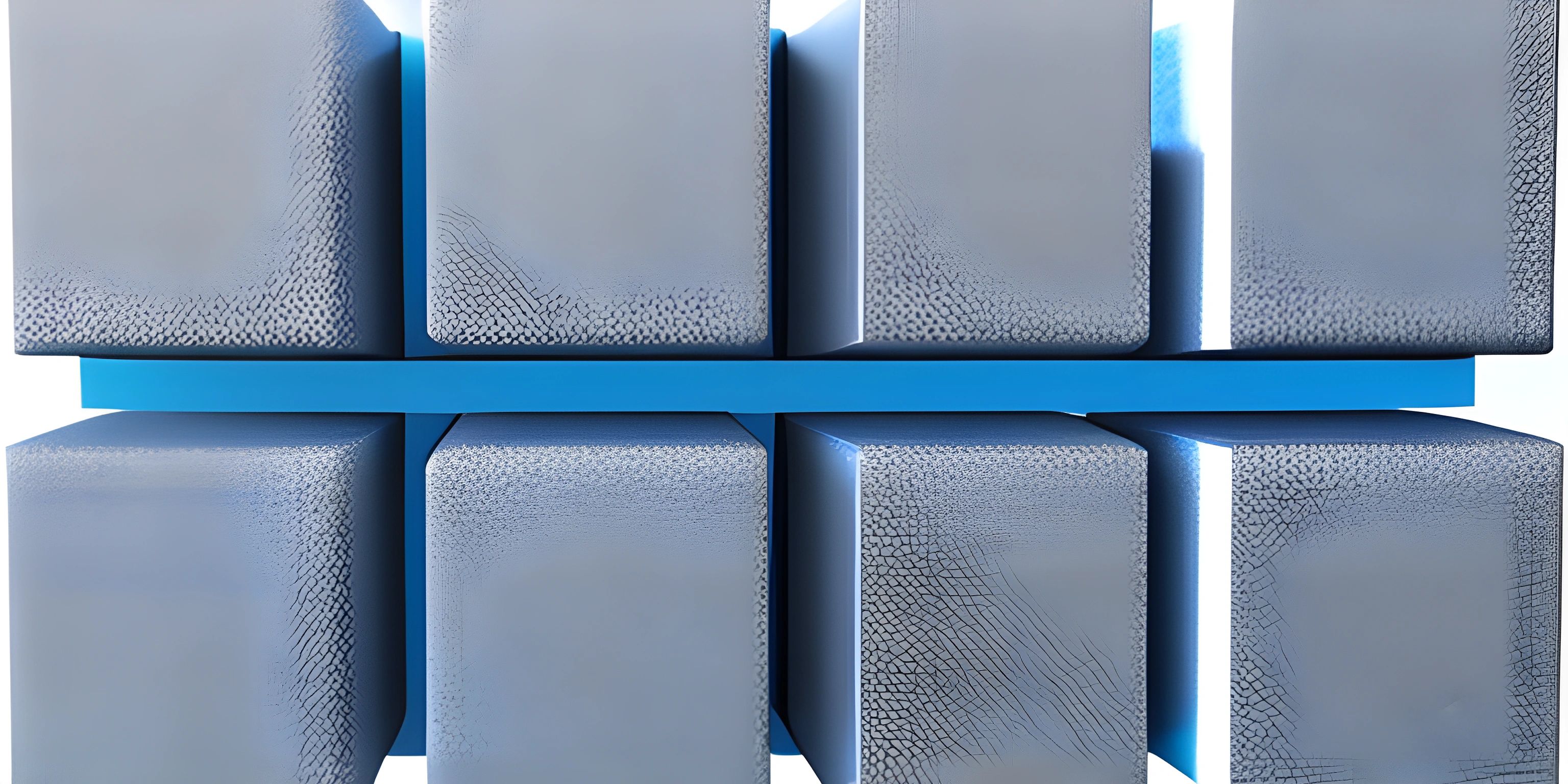
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Object-Oriented Programming (OOP) is a programming paradigm that models real-world entities and their interactions. It provides a powerful way to design, organize, and maintain code. Let's dive into the basics of OOP and explore its advantages.
Core Concepts
The foundation of OOP lies in three core concepts: encapsulation, inheritance, and polymorphism.
Encapsulation
Encapsulation is the process of bundling data and methods that operate on that data within a single unit, called an object. This object is an instance of a class, which acts as a blueprint for creating objects with similar characteristics.
Encapsulation provides a way to hide the internal workings of an object from the outside world, allowing access only through public interfaces called methods. This approach improves code maintainability and reduces the likelihood of unintended side effects.
Inheritance
Inheritance is the mechanism that allows a new class, called the subclass, to inherit properties and behaviors from an existing class, called the superclass. This concept promotes code reuse and modular design.
For example, imagine a superclass Animal
with the properties name
and age
. We can create subclasses like Dog
and Cat
that inherit these properties from Animal
, while also adding their own unique characteristics such as the Dog
class having a bark()
method and the Cat
class having a meow()
method.
Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common superclass. It enables a single interface to represent different types of objects, making it easier to add new classes without changing existing code.
For example, if we have a method make_sound(animal)
, it can accept both Dog
and Cat
objects because they both inherit from the Animal
superclass. The method can then call the appropriate sound-making method for each object (bark()
for a Dog
, and meow()
for a Cat
), without needing to know their specific class.
Advantages
OOP offers several advantages, including:
- Modularity: Encapsulation allows the separation of concerns, making it easier to maintain and modify code.
- Code Reuse: Inheritance promotes code reuse, reducing the need to rewrite common functionality.
- Extensibility: Polymorphism enables the addition of new classes and objects without changing existing code, making it more adaptable to changing requirements.
- Readability: OOP closely models the real world, making the code more intuitive and easier to understand.
Now that you have a basic understanding of Object-Oriented Programming, you can explore OOP in Python, OOP in Java, or OOP in your preferred programming language.
FAQ
What is Object-Oriented Programming?
Object-Oriented Programming (OOP) is a programming paradigm that focuses on modeling real-world entities and their interactions using objects, classes, and methods. It provides a powerful way to design, organize, and maintain code.
What are the core concepts of OOP?
The three core concepts of OOP are encapsulation, inheritance, and polymorphism. Encapsulation bundles data and methods within objects. Inheritance enables a class to inherit properties and behaviors from another class. Polymorphism allows objects of different classes to be treated as objects of a common superclass, simplifying code.
How does OOP improve code maintainability?
OOP improves code maintainability through encapsulation, which hides the internal workings of objects and exposes only their public interfaces. This separation of concerns makes it easier to manage and modify code without causing unintended side effects.