Introduction to Assembly Programming on Intel x86
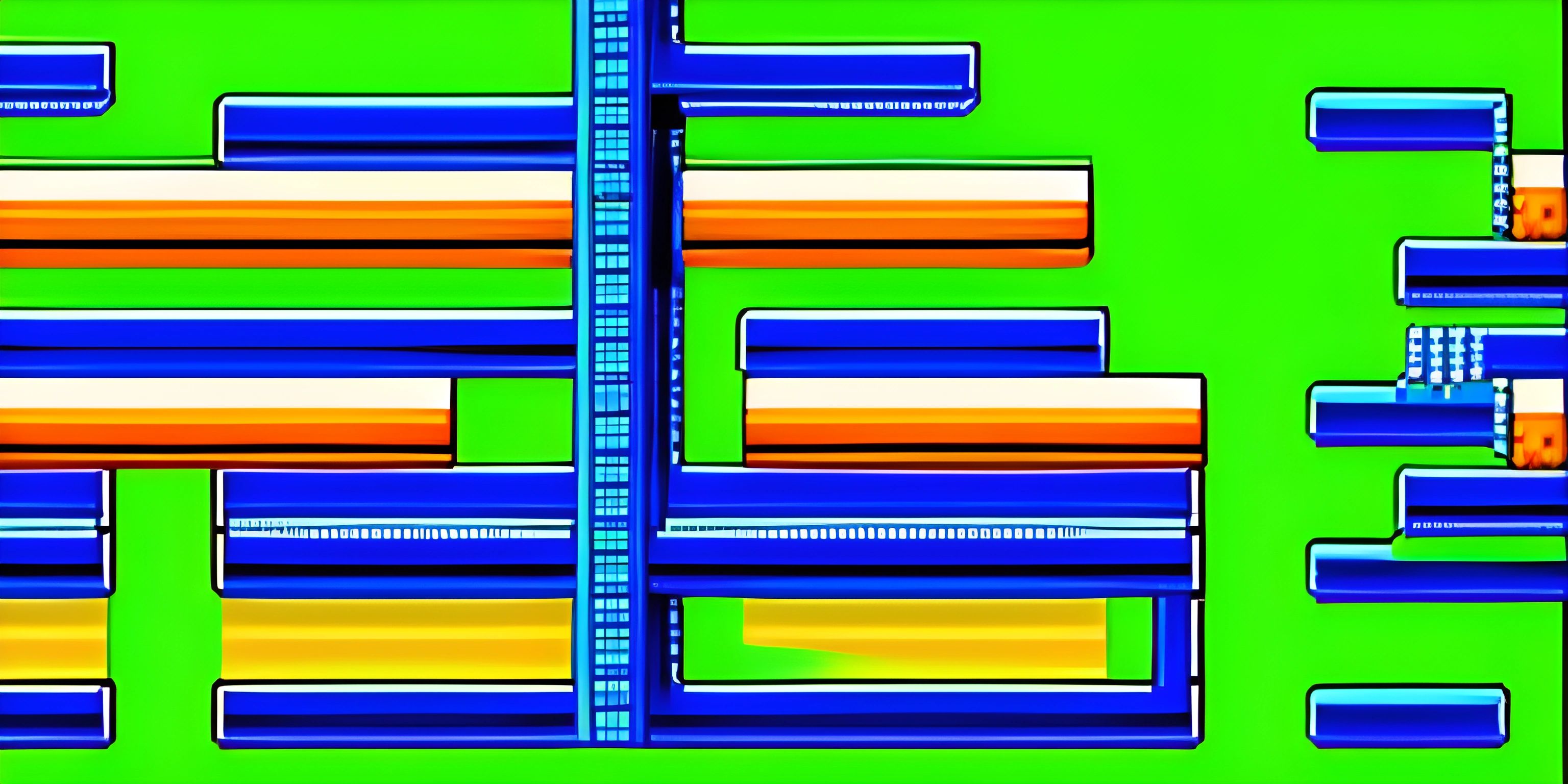
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Welcome to the world of assembly programming on the Intel x86 architecture! If you've ever wondered what it feels like to speak directly to your computer, assembly language is as close as you can get without getting electrocuted. It's like communicating with a friend using only winks and eyebrow raises—minimal, precise, and often confusing to outsiders.
What is Assembly Language?
Assembly language is a low-level programming language that is closely related to a computer's machine code. Unlike high-level languages like Python or JavaScript, which are more abstract and user-friendly, assembly requires you to manage every tiny detail of the hardware. Think of it as the difference between giving someone a fully baked cake (high-level) and handing them a recipe with raw ingredients (assembly).
The Intel x86 Architecture
The Intel x86 architecture is one of the most widely used instruction set architectures (ISAs) in the world. It's the backbone of many personal computers and servers. Understanding x86 assembly can give you a deeper insight into how computers operate at a fundamental level, which can be incredibly powerful for optimization and debugging.
Registers and Memory
In assembly, you'll often work directly with the CPU's registers. Think of registers as the CPU's tiny, super-fast scratchpads. Intel x86 has several general-purpose registers, including:
- EAX: Accumulator for operands and results data
- EBX: Base pointer for memory access
- ECX: Counter for loops
- EDX: Data pointer
Here's a simple example of moving data between registers:
; Move the value 10 into EAX MOV EAX, 10 ; Move the value in EAX to EBX MOV EBX, EAX ; Now both EAX and EBX contain the value 10
Basic Instructions
The Intel x86 instruction set includes a variety of commands for arithmetic, logic, control flow, and data movement. Some basic instructions include:
- MOV: Transfer data between registers
- ADD: Add two values
- SUB: Subtract one value from another
- CMP: Compare two values
- JMP: Jump to a different part of the program
Consider this simple arithmetic example:
; Initialize registers MOV EAX, 5 MOV EBX, 3 ; Perform addition (EAX = EAX + EBX) ADD EAX, EBX ; Now EAX contains 8
Control Flow
Control flow in assembly is managed through jumps and conditional jumps. These instructions allow your program to make decisions and iterate, much like loops and conditionals in high-level languages.
; Initialize register and counter MOV ECX, 5 start_loop: ; Decrement counter DEC ECX ; If counter is not zero, jump back to start_loop JNZ start_loop ; When ECX is zero, the loop exits
In this example, DEC
decrements ECX
by 1, and JNZ
(Jump if Not Zero) causes the program to jump back to start_loop
as long as ECX
is not zero.
Assembling and Running Your Code
To run your assembly code, you'll need an assembler (like NASM) and a linker. Here's a brief overview of the process:
- Write your assembly code in a
.asm
file. - Use NASM to assemble the code into an object file:
nasm -f elf32 yourfile.asm
- Link the object file to create an executable:
ld -m elf_i386 -o yourfile yourfile.o
- Run the executable:
./yourfile
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is assembly language used for?
Assembly language is used for tasks that require direct hardware manipulation, performance optimization, and real-time processing. It's often utilized in system programming, embedded systems, and situations where you need precise control over the CPU.
How does assembly language differ from high-level languages?
Assembly language is much lower-level and closer to machine code than high-level languages. It requires more detailed management of hardware resources, which can make it more complex and less portable. However, it offers greater control and efficiency.
What are some common registers in Intel x86?
Common general-purpose registers in Intel x86 include EAX, EBX, ECX, and EDX. These registers are used for various operations, such as arithmetic calculations, memory access, and loop counting.
Why is learning assembly language important?
Learning assembly language can help you understand how computers work at a fundamental level. It can improve your debugging skills, enable you to optimize performance-critical code, and make you a more versatile programmer.
What tools do I need to write and run assembly code?
You'll need an assembler (like NASM) to convert your assembly code into machine code, and a linker to create an executable file. Additionally, you'll need a text editor to write your code and a terminal to run the commands.