Comprehensive Guide to Registers in Intel x86 Architecture
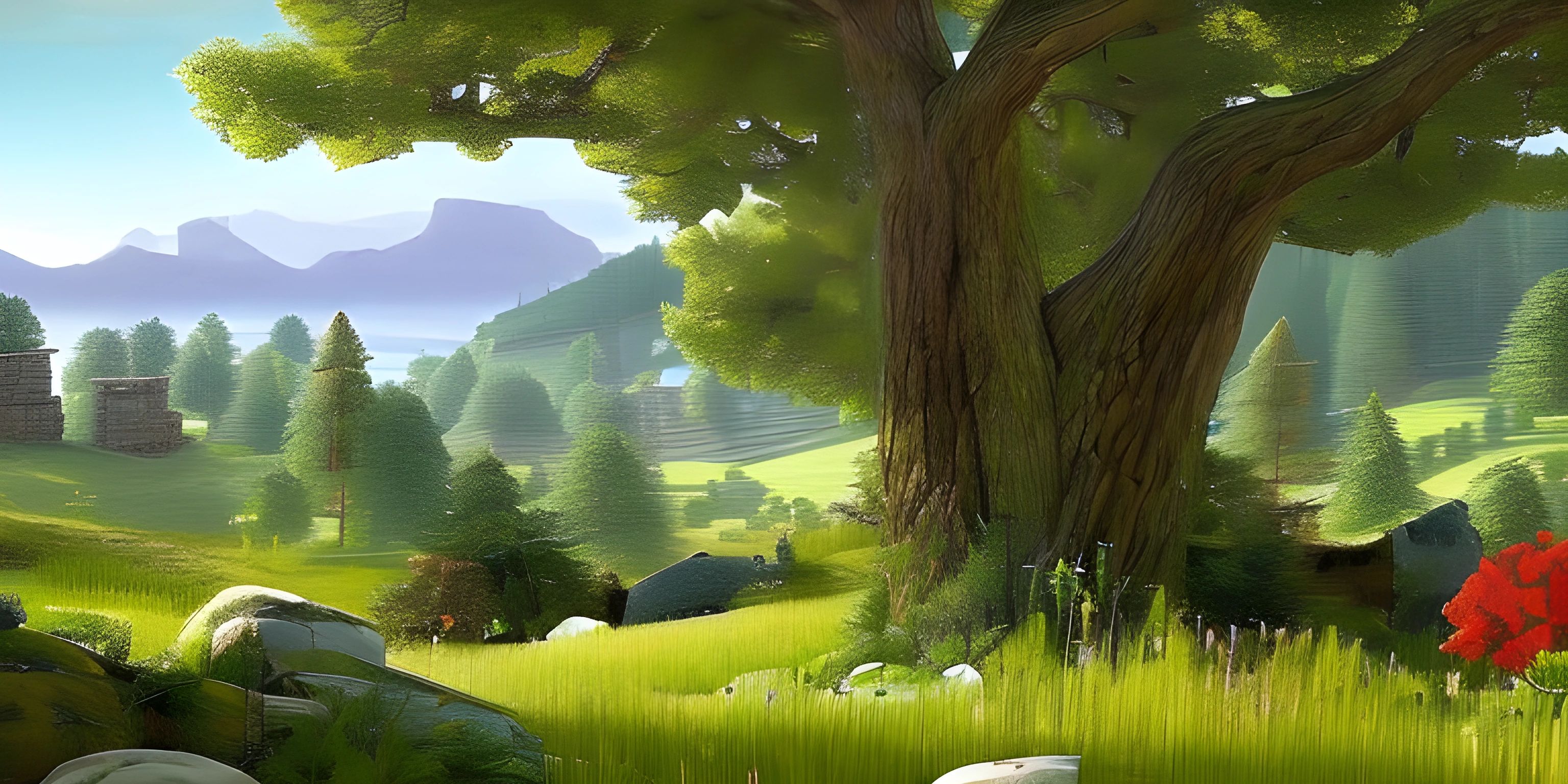
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Understanding how the Intel x86 architecture operates under the hood is crucial for anyone diving into low-level programming or trying to optimize their high-level code. At the core of this architecture lie the registers, which act like the CPU's tiny, super-fast storage units. Think of them as the VIP room in a nightclub—only the most critical data gets in!
General-Purpose Registers
First off, let's talk about the general-purpose registers, the workhorses of the x86 architecture. These registers are versatile and can be used for a variety of tasks, from arithmetic operations to data storage.
The Famous EAX, EBX, ECX, EDX
These four are the Kardashians of the register world—everyone knows them, and they are always in the spotlight.
- EAX: Known as the accumulator register, it's often used in arithmetic operations.
- EBX: The base register, frequently utilized for base pointers in memory accesses.
- ECX: The count register, typically used for loop counters.
- EDX: The data register, often involved in input/output operations.
Here's a simple example in x86 Assembly that shows these registers in action:
section .data num1 dd 10 num2 dd 20 section .text global _start _start: mov eax, [num1] ; Move the value of num1 into EAX add eax, [num2] ; Add the value of num2 to EAX ; Now EAX contains 30 ; Exit the program mov eax, 60 ; syscall: exit xor edi, edi ; status: 0 syscall
Extended Registers R8-R15
With the advent of 64-bit architecture, additional general-purpose registers R8 through R15 were introduced. These provide more flexibility and are denoted with "R" instead of "E" (for "extended").
Special Registers
Apart from the famous four, there are other general-purpose registers like ESP (Stack Pointer), EBP (Base Pointer), ESI (Source Index), and EDI (Destination Index), each serving specific roles, especially in function calling conventions.
Segment Registers
Segment registers are the bouncers of our nightclub. They manage the different "segments" of memory, ensuring that data doesn't overflow into the wrong places.
The Quintessential Segment Registers
- CS (Code Segment): Points to the segment containing the current program code.
- DS (Data Segment): Points to the segment containing data.
- SS (Stack Segment): Points to the segment containing the stack.
- ES, FS, GS: Additional segment registers for extra data segments.
Segment registers are often used in conjunction with general-purpose registers for memory access. Here's an example:
section .data message db 'Hello, World!', 0 section .text global _start _start: mov edx, len ; length of our message mov ecx, message ; pointer to message mov ebx, 1 ; file descriptor for stdout mov eax, 4 ; syscall number for sys_write int 0x80 ; call kernel ; Exit the program mov eax, 1 ; syscall: exit xor ebx, ebx ; status: 0 int 0x80 section .data len equ $ - message
Control Registers
Control registers are like the club's security cameras and alarm systems—they help monitor and control the CPU's operations.
CR0-CR4
- CR0: Contains flags that control the CPU's operating mode.
- CR2: Holds the address causing a page fault.
- CR3: Used to manage memory paging.
- CR4: Contains several control flags that enable extensions.
Here's a snippet showing how to access control registers:
mov eax, cr0 ; Move the value of CR0 into EAX or eax, 0x1 ; Set the first bit of EAX mov cr0, eax ; Move the modified value back into CR0
Debug Registers
Debug registers are the club's secret agents, working behind the scenes to keep everything running smoothly. They are used for setting breakpoints and monitoring specific memory locations.
DR0-DR7
- DR0-DR3: Hold linear addresses for breakpoints.
- DR6: Status register.
- DR7: Control register.
Floating Point and SIMD Registers
These registers are the club's special VIPs, reserved for high-precision and SIMD (Single Instruction, Multiple Data) operations.
x87 FPU Registers
The x87 FPU (Floating Point Unit) uses a stack of eight registers, st(0)
to st(7)
, for floating-point calculations.
MMX, SSE, and AVX
- MMX: Registers
mm0
tomm7
for multimedia tasks. - SSE: Registers
xmm0
toxmm15
for SIMD operations. - AVX: Registers
ymm0
toymm15
, extending SSE capabilities.
Here's an SSE example:
section .data arr1 dq 1.0, 2.0, 3.0, 4.0 arr2 dq 5.0, 6.0, 7.0, 8.0 result dq 0.0, 0.0, 0.0, 0.0 section .text global _start _start: movaps xmm0, [arr1] ; Load arr1 into xmm0 movaps xmm1, [arr2] ; Load arr2 into xmm1 addps xmm0, xmm1 ; Add packed single-precision floats movaps [result], xmm0 ; Store the result ; Exit the program mov eax, 60 ; syscall: exit xor edi, edi ; status: 0 syscall
Understanding these registers and their roles can significantly improve your ability to write efficient and effective assembly code. Registers are vital for manipulating data, controlling program flow, and optimizing performance.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).
FAQ
What are general-purpose registers in Intel x86 architecture?
General-purpose registers in Intel x86 architecture, such as EAX, EBX, ECX, and EDX, are versatile registers used for a variety of tasks including arithmetic operations, data storage, and loop counters.
What is the function of segment registers?
Segment registers manage different segments of memory in the Intel x86 architecture, ensuring that data does not overflow into the wrong areas. Key segment registers include CS (Code Segment), DS (Data Segment), and SS (Stack Segment).
How are control registers used in Intel x86 architecture?
Control registers in Intel x86 architecture, such as CR0 to CR4, are used to monitor and control the CPU's operations. They contain flags and addresses that manage memory paging and operating modes.
What are the debug registers in Intel x86 architecture?
Debug registers, like DR0 to DR7, are specialized registers used for setting breakpoints and monitoring specific memory locations during debugging processes.
Can you explain the use of floating point and SIMD registers?
Floating point and SIMD (Single Instruction, Multiple Data) registers, such as those in the x87 FPU, MMX, SSE, and AVX sets, are used for high-precision calculations and multimedia tasks, allowing for efficient parallel processing of data.