Python Array Manipulation
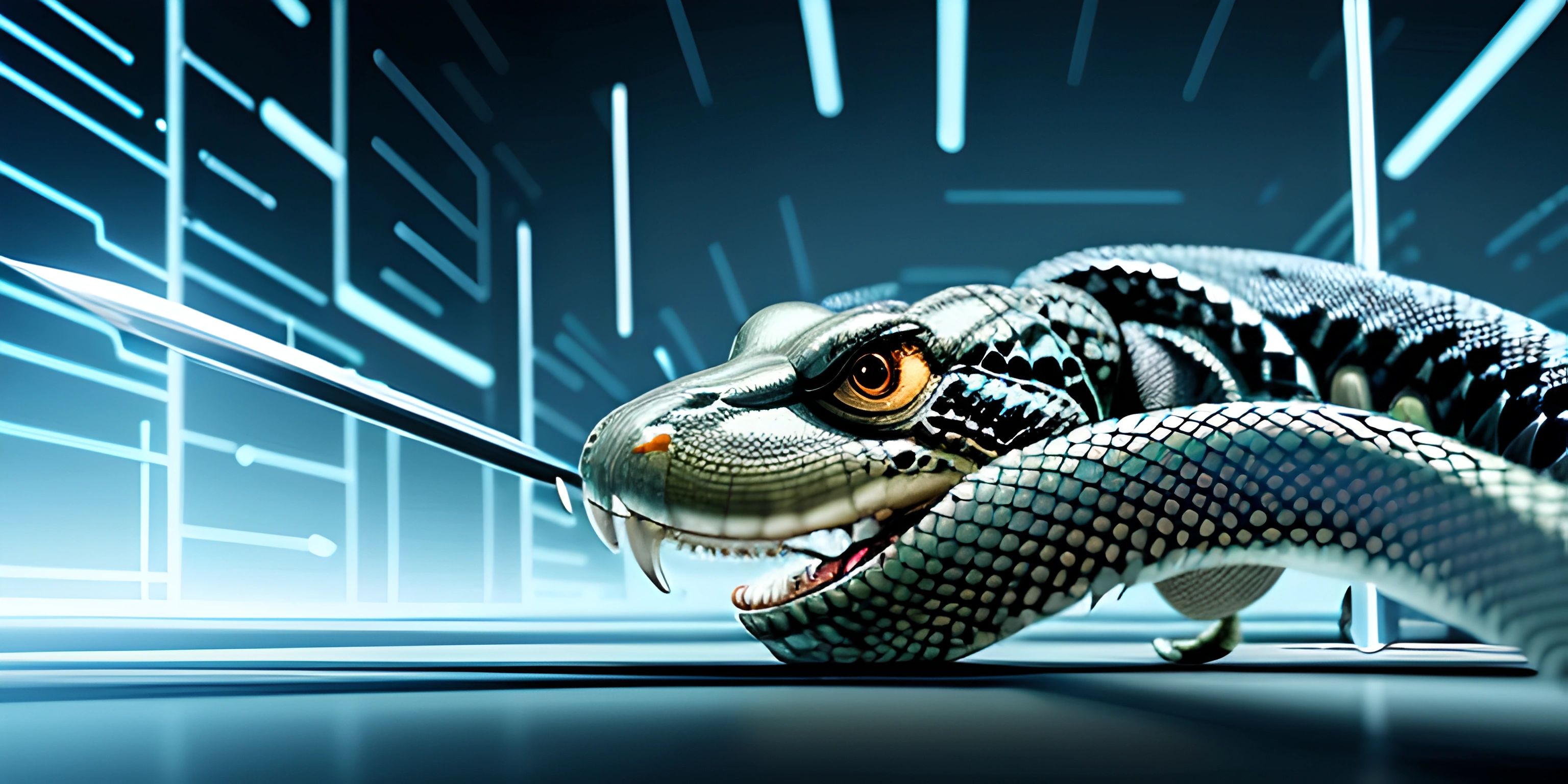
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Arrays are a fundamental data structure used in almost all programming languages. In Python, they are called lists. A list is like a container that stores multiple values, allowing you to access, modify, and manipulate them in various ways. Think of lists as a stack of dishes, where you can add, remove, or rearrange dishes as needed.
Creating a Python List
Before we start manipulating lists, let's create one. In Python, you can define a list using square brackets []
and separating elements with commas. Here's an example:
my_list = ["apple", "banana", "cherry"]
Now that we have a list, let's dive into some common manipulation techniques.
Accessing Elements
To access a specific element in a list, use its index (position) within square brackets []
. List indices in Python start at 0, not 1. So, to access the first element, use my_list[0]
. Here's an example:
print(my_list[1]) # Output: banana
Slicing
Slicing allows you to extract a portion of a list, creating a new list. To slice a list, use the colon :
operator within square brackets []
. Here's the syntax:
new_list = original_list[start:end]
start
: The index of the first element you want to include in the slice (inclusive).end
: The index of the last element you want to include in the slice (exclusive).
If you leave out start
, it defaults to 0. If you leave out end
, it defaults to the length of the list. Here's an example:
sliced_list = my_list[1:3] print(sliced_list) # Output: ['banana', 'cherry']
Appending Elements
To add an element to the end of a list, use the append()
method. Here's an example:
my_list.append("orange") print(my_list) # Output: ['apple', 'banana', 'cherry', 'orange']
Inserting Elements
To insert an element at a specific index, use the insert()
method. The syntax is as follows:
original_list.insert(index, element)
index
: The position at which you want to insert the element.element
: The element you want to insert.
Here's an example:
my_list.insert(1, "grape") print(my_list) # Output: ['apple', 'grape', 'banana', 'cherry', 'orange']
Deleting Elements
To remove an element from a list, use the del
keyword followed by the element's index. Here's an example:
del my_list[1] print(my_list) # Output: ['apple', 'banana', 'cherry', 'orange']
You can also delete a slice of elements using the same syntax:
del my_list[1:3] print(my_list) # Output: ['apple', 'orange']
Now that you've learned the basics of Python list manipulation, you can start applying these techniques in your own programs. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Word Splitter (psst, it's free!).
FAQ
What is slicing in Python arrays and how can I use it?
Slicing in Python arrays is a technique used to extract a portion of the array or modify specific parts of it. To use slicing, you need to provide a start index, an end index, and an optional step value. The syntax for slicing is array[start:end:step]
. For example, to extract elements from index 2 to 4, you would do array[2:5]
. Remember that the end index is exclusive.
How can I append an element to a Python array?
You can append an element to a Python array using the append()
method. Simply pass the element you want to add as an argument to the method. For example:
array = [1, 2, 3] array.append(4) print(array) # Output: [1, 2, 3, 4]
How do I delete an element from a Python array by index?
To delete an element from a Python array by index, you can use the pop()
method. Pass the index of the element you want to remove as an argument to the method. For example:
array = [1, 2, 3, 4] array.pop(1) print(array) # Output: [1, 3, 4]
Note that the pop()
method also returns the removed element.
Can I append multiple elements to a Python array at once?
Yes, you can append multiple elements to a Python array at once using the extend()
method. Pass the list of elements you want to add as an argument to the method. For example:
array = [1, 2, 3] array.extend([4, 5, 6]) print(array) # Output: [1, 2, 3, 4, 5, 6]
How can I delete an element from a Python array by value?
To delete an element from a Python array by value, you can use the remove()
method. Pass the value you want to remove as an argument to the method. For example:
array = [1, 2, 3, 4, 2] array.remove(2) print(array) # Output: [1, 3, 4, 2]
Note that the remove()
method only removes the first occurrence of the specified value.