Introduction to Programming Basics
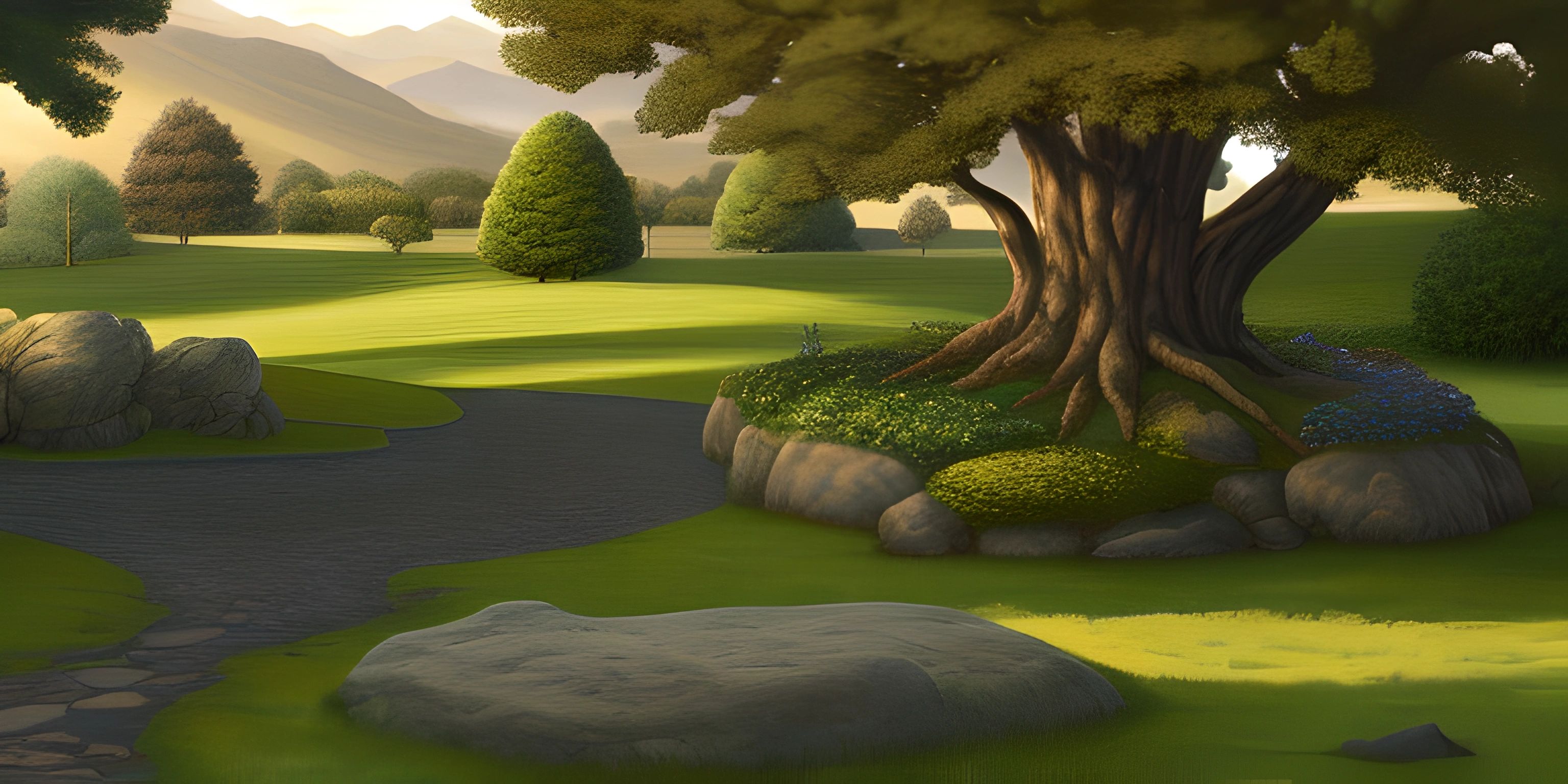
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Programming is the process of creating a set of instructions that tell a computer how to perform a task. It involves writing code in a programming language, which the computer can then interpret and execute. In this article, we will introduce you to some fundamental programming concepts and terminology.
Variables
Variables are used to store data in a program. They have a name and a value, which can be changed during the execution of the program. Variables can hold different types of data, such as numbers, text, or more complex data structures.
var name = "Alice"; var age = 30;
Data Types
Data types are the different types of data that can be stored in variables. Common data types include:
- Numbers: Integers and floating-point numbers (decimals).
- Strings: Text, represented by characters enclosed in double quotes.
- Booleans: True or false values.
- Arrays: Ordered collections of elements.
- Objects: Collections of key-value pairs.
var number = 42; var string = "Hello, world!"; var boolean = true; var array = [1, 2, 3, 4, 5]; var object = { "key": "value" };
Functions
Functions are blocks of code that can be defined and called by name. They can accept input (arguments) and return a value. Functions are used to organize and reuse code.
function greet(name) { return "Hello, " + name + "!"; } var message = greet("Alice"); console.log(message); // Output: Hello, Alice!
Control Structures
Control structures are used to control the flow of execution in a program. They include:
- Conditional statements: Execute a block of code if a certain condition is true (e.g.,
if
,else if
,else
). - Loops: Execute a block of code repeatedly (e.g.,
for
,while
,do-while
).
if (age >= 18) { console.log("You are an adult."); } else { console.log("You are a minor."); } for (var i = 0; i < 5; i++) { console.log("Iteration number " + i); }
Comments
Comments are lines of text in the code that are not executed by the computer. They are used to explain the code and make it easier to understand.
// This is a single-line comment /* This is a multi-line comment */
These are just a few of the basic concepts and terms you'll encounter in programming. As you continue to learn and explore different languages, you'll build on these fundamentals and develop a deeper understanding of programming.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Basic Concepts (psst, it's free!).
FAQ
What are the core programming concepts covered in this article?
This article covers the following key programming concepts:
- Variables and data types
- Functions and methods
- Control structures (loops and conditionals)
- Arrays and lists
How can I start learning programming basics?
The article "Introduction to Programming Basics" is an excellent starting point for beginners. Alongside reading the article, you should also practice the concepts shared through hands-on coding exercises and real-world examples. Experiment with different programming languages, such as Python, JavaScript, or Java, to find which one suits you best.
Are there any prerequisites for understanding programming basics?
There are no strict prerequisites for learning programming basics, but having a basic understanding of mathematical concepts and logical reasoning will be helpful. It's essential to have a curious mindset, willingness to learn, and patience to solve problems through trial and error.
Can I learn programming basics without any prior coding experience?
Absolutely! The "Introduction to Programming Basics" article is designed to help beginners with no prior coding experience to understand the fundamental concepts and terminology used in programming.
How important is it to learn multiple programming languages?
Learning multiple programming languages can be beneficial, as each language has its strengths, use-cases, and syntax. However, it's more important to have a solid understanding of the core programming concepts and problem-solving skills. Once you're proficient in these basics, you'll be able to quickly adapt to other languages and expand your skill set.