Array Basics
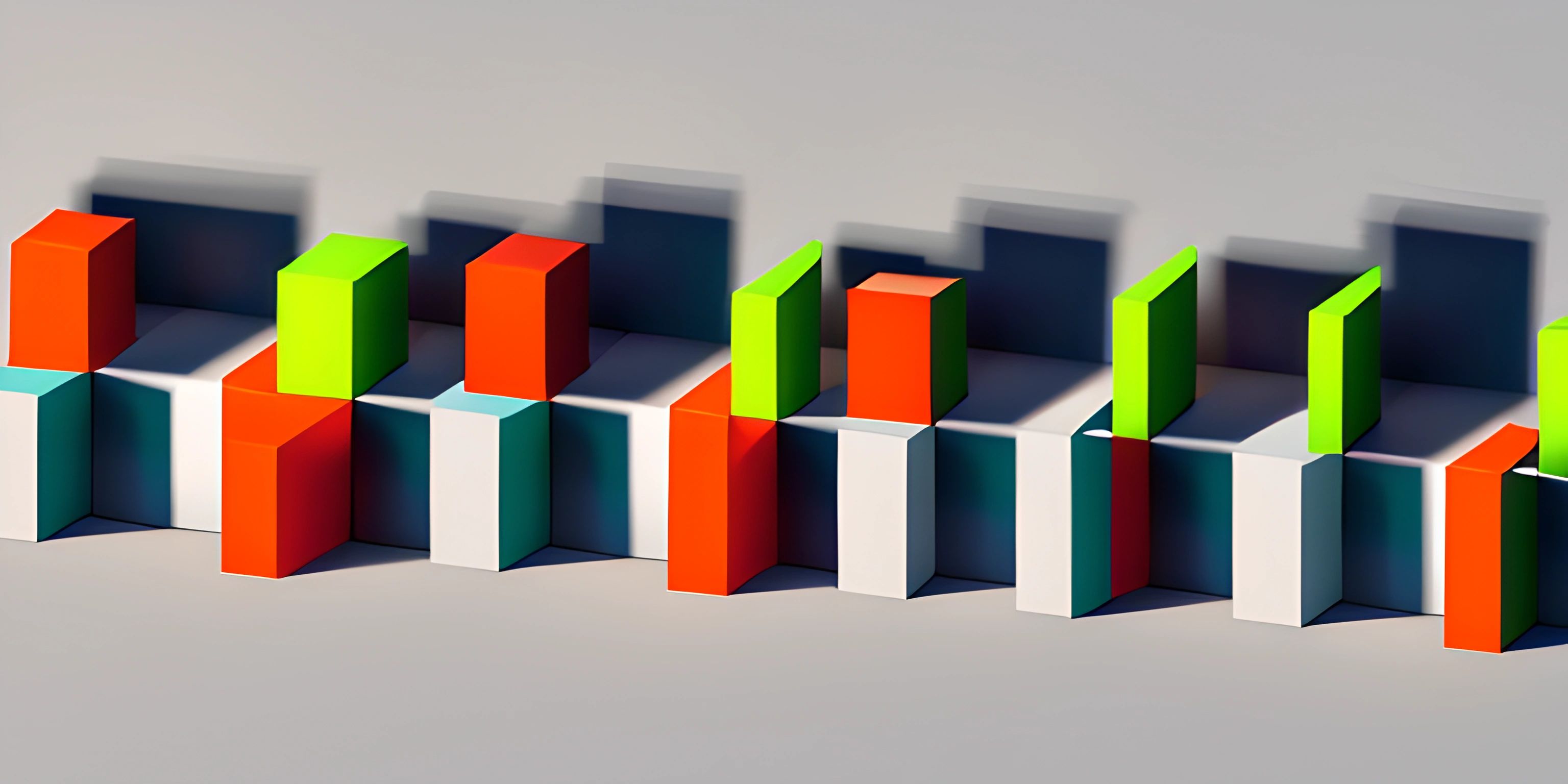
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine you're a chef in a restaurant and you've got a lot of ingredients to keep track of. You could keep them all scattered around the kitchen, but you'd probably lose track of some of them. Instead, you organize them on shelves, with each shelf holding a specific type of ingredient. This makes your life much easier, right?
Well, arrays in programming are like those shelves. They help you keep track of and organize multiple pieces of data in an orderly and accessible manner. Just like you can add, remove, or rearrange ingredients on the shelves, you can do the same with data in arrays.
What is an Array?
An array is a data structure used in programming languages to store and manage multiple values (called elements) in a single variable. These elements are stored in contiguous memory locations, and each element can be accessed using a unique integer index, starting from 0.
Think of an array as a row of boxes, each containing a piece of data. The boxes are numbered (indexed) from left to right, with the first box having index 0, second box index 1, and so on.
Creating an Array
To create an array, you'll need to specify its type, name, and size. The syntax varies depending on the programming language you're using.
Here's an example in Python:
my_array = [1, 2, 3, 4, 5]
In this example, we created an array called my_array
containing five integer elements.
Accessing Array Elements
To access the elements of an array, you'll use their index. Remember, array indices start from 0. So, to access the first element of my_array
, you would use:
first_element = my_array[0]
Similarly, to access the third element, you would use:
third_element = my_array[2]
Modifying Array Elements
Once you have an array, you can easily modify its elements. To do so, simply use the index and assign a new value:
my_array[1] = 42
This code assigns the value 42 to the second element of my_array
.
Array Operations
Arrays support various operations, such as adding new elements, removing elements, or finding the length of the array. The specific operations available and their syntax depend on the programming language you're using.
For example, in Python, you can find the length of an array using the built-in len()
function:
array_length = len(my_array)
Now that you have a basic understanding of arrays and how to use them, remember to always keep your data organized, just like a chef with their ingredients. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Word Splitter (psst, it's free!).
FAQ
What is an array and why is it important in programming?
An array is a collection of elements, typically of the same data type, stored in a contiguous block of memory. It is important in programming because it allows you to store and manage multiple values efficiently, making it easier to organize and process data.
How do I declare and initialize an array in a programming language?
The syntax to declare and initialize an array varies between programming languages. Here's an example in JavaScript:
let numbers = [1, 2, 3, 4, 5];
In this example, we declared an array called numbers
and initialized it with five integer values.
Can I store different data types in an array?
While arrays typically store elements of the same data type, some programming languages, like JavaScript, allow you to store different data types within the same array. However, it's generally recommended to keep arrays consistent by storing elements of the same data type, as it makes the code more predictable and easier to maintain.
How do I access and modify elements in an array?
Array elements can be accessed and modified using their index, which starts from 0. Here's an example in Python:
colors = ["red", "blue", "green"] print(colors[1]) # Output: blue colors[1] = "yellow" print(colors) # Output: ["red", "yellow", "green"]
How can I loop through an array and perform operations on its elements?
You can use a loop, like a for
loop, to iterate through the elements of an array. Here's an example in Java:
int[] numbers = {1, 2, 3, 4, 5}; for (int i = 0; i < numbers.length; i++) { numbers[i] *= 2; } // The array now contains [2, 4, 6, 8, 10]