Language Interoperability
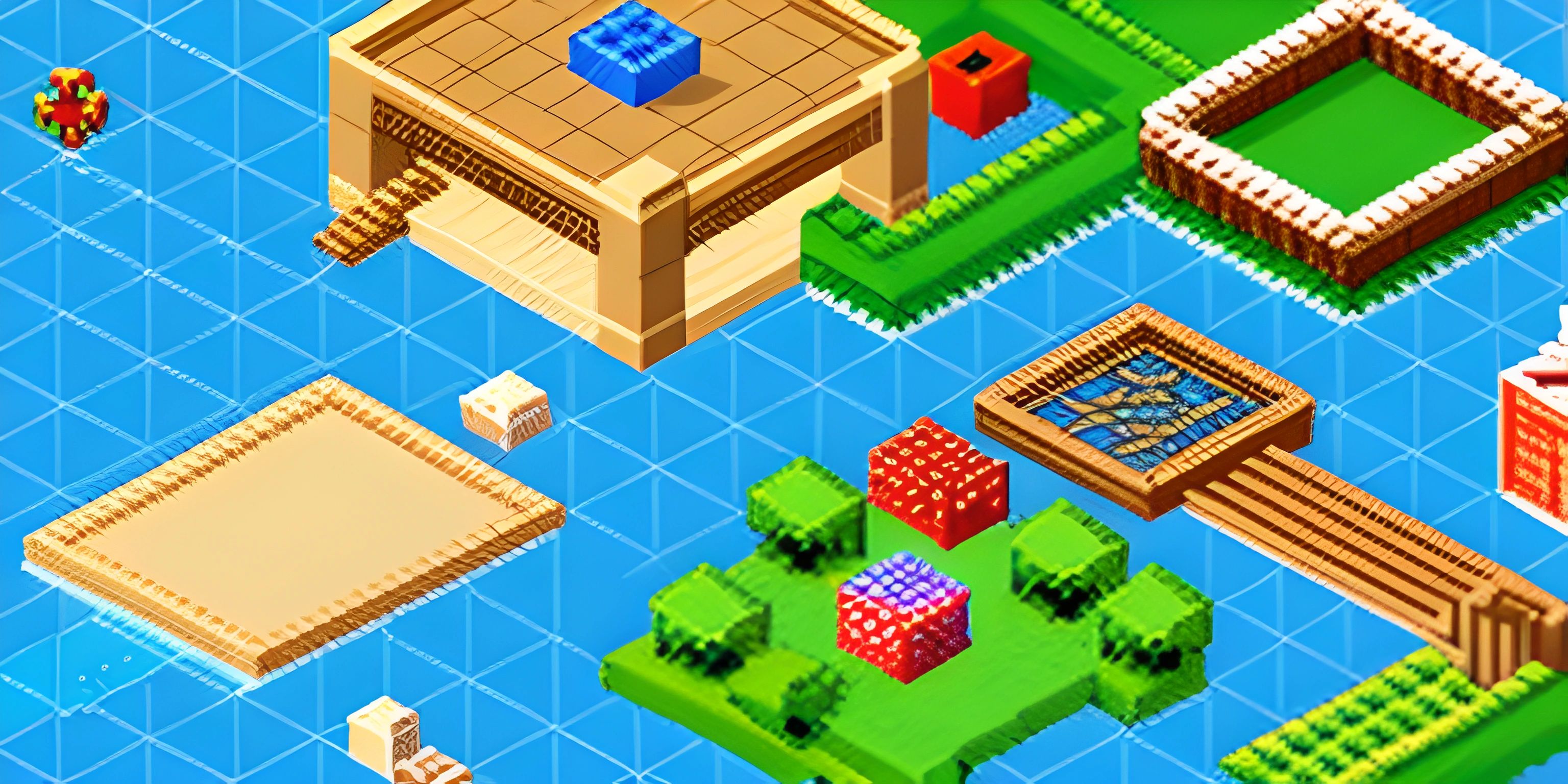
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the world of programming, there are many different languages, each with their unique strengths and weaknesses. Often, a single language may not have all the features or libraries needed to build an entire application. That's where language interoperability comes into play, allowing different languages to communicate and work together.
Foreign Function Interface (FFI)
One way for programming languages to cooperate is through the use of a Foreign Function Interface (FFI). An FFI allows a program to call functions written in another language directly. This can be useful when you want to take advantage of a library or feature that's only available in another language.
For example, imagine you're writing a Python program, but you want to use a library that's only available in C. You can use Python's built-in ctypes
library to interface with the C library, essentially enabling your Python program to "speak C" when needed.
Here's a simple example demonstrating FFI usage with Python and C:
// my_math.c int add(int a, int b) { return a + b; }
# ffi_example.py from ctypes import CDLL, c_int my_math = CDLL("my_math.so") add = my_math.add add.argtypes = [c_int, c_int] add.restype = c_int result = add(2, 3) print(result) # Output: 5
APIs and Microservices
Another approach to language interoperability is through the use of Application Programming Interfaces (APIs) and microservices. APIs are standardized ways of communicating between different software components, while microservices are small, independent applications that can be combined to build a larger system.
By designing your application as a collection of microservices, each implemented in the most suitable language, you can achieve language interoperability by having them communicate via APIs. Common API communication protocols include REST and gRPC.
For example, imagine you're building a web application that has a frontend written in JavaScript and a backend written in Go. The frontend and backend can communicate with each other using RESTful APIs or gRPC, allowing them to work together despite being written in different languages.
// main.go (Go backend) package main import ( "fmt" "net/http" ) func main() { http.HandleFunc("/api/greet", func(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello from the Go backend!") }) http.ListenAndServe(":8080", nil) }
// frontend.js (JavaScript frontend) fetch("http://localhost:8080/api/greet") .then(response => response.text()) .then(text => console.log(text)); // Output: Hello from the Go backend!
Polyglot Runtimes
A polyglot runtime is an environment that supports running multiple programming languages. This can be another way to achieve language interoperability. One example is the GraalVM, which is a high-performance runtime that supports running programs written in languages like JavaScript, Ruby, Python, and more.
By using a polyglot runtime, you can run different parts of your application in the languages best suited for each task, while still maintaining seamless communication between them.
In conclusion, language interoperability is a powerful concept that allows you to harness the strengths of multiple programming languages in a single application. Whether you choose to use FFIs, APIs and microservices, or polyglot runtimes, the key is to find the right combination that works best for your project.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is language interoperability?
Language interoperability is the ability of different programming languages to communicate and work together seamlessly. This concept allows developers to use multiple programming languages within a single project, enabling them to take advantage of each language's unique features and strengths.
Why is language interoperability important in software development?
Language interoperability is important because it allows developers to:
- Leverage the strengths of different programming languages
- Reuse existing code written in other languages
- Collaborate with other developers who have expertise in different languages
- Create more flexible and adaptable software systems
- Improve the overall efficiency and maintainability of a project
How can different programming languages communicate with each other?
Different programming languages can communicate with each other through various mechanisms, such as:
- APIs (Application Programming Interfaces)
- Foreign Function Interfaces (FFI)
- Language bindings
- Middleware software
- Inter-process communication (IPC)
Can you provide an example of using language interoperability in a project?
Sure! Let's say you're developing a web application that requires high-performance numerical computations. You could write the main application using a language like Python for its ease of use and extensive libraries, while leveraging the performance of C++ for the numerical computation part.
To achieve this, you can create a Python module that uses C++ through a Foreign Function Interface (FFI) like ctypes
or Cython
. This way, your Python application can call the high-performance C++ code, benefiting from the strengths of both languages.
What are some challenges associated with language interoperability?
While language interoperability has its advantages, it also comes with a few challenges, such as:
- Increased complexity in code management and debugging
- Potential performance overhead when using inter-language communication
- Ensuring compatibility between different language versions and libraries
- The need to learn and understand multiple programming languages
- Difficulty in finding developers with expertise in multiple languages