A Beginner's Guide to Java Syntax
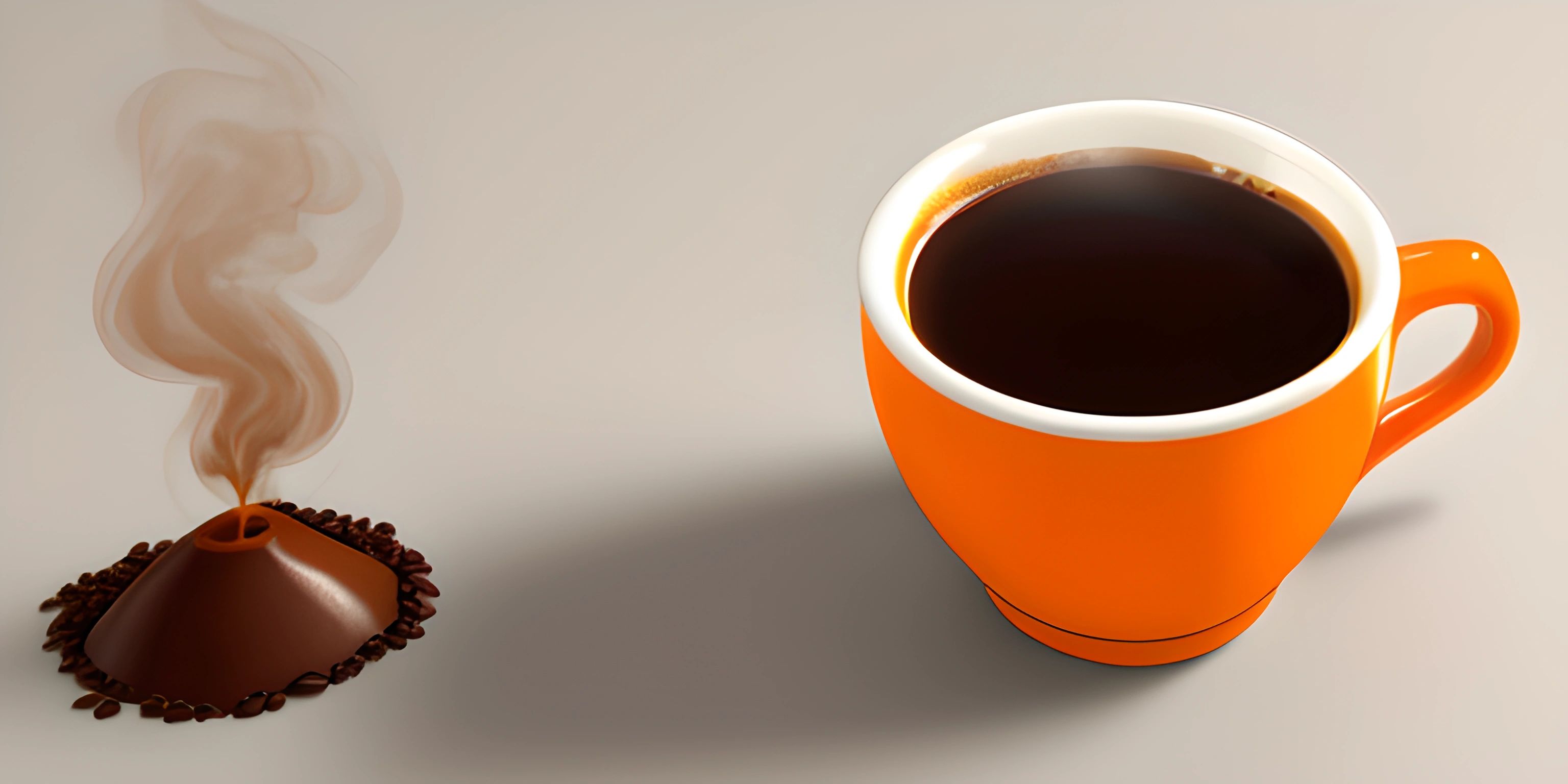
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Programming in Java is like learning to ride a bike. It might be a bit tricky at first, but once you get the hang of it, you'll find yourself zipping through the streets of code with ease. In this guide, we'll introduce you to some of the most essential Java syntax elements, so you can start your journey with confidence.
Comments
First things first, let's talk about comments. Comments are crucial for humans to understand what's going on in the code. In Java, there are two types of comments:
-
Single-line comments: These start with
//
and continue until the end of the line.// This is a single-line comment in Java
-
Multi-line comments: These begin with
/*
and end with*/
. Everything in between is considered a comment./* This is a multi-line comment in Java, which can span multiple lines of text. */
Variables and Data Types
In Java, a variable is like a little box that can store a piece of data. Each variable has a data type that defines what kind of information it can hold. Some common data types in Java are:
int
: Integer numbersfloat
: Floating-point numbers (decimal values)double
: Double-precision floating-point numberschar
: Single charactersboolean
: True or false values
To declare a variable, we use the following syntax:
<dataType> <variableName> = <value>;
For example:
int age = 25; float height = 1.75f; double weight = 70.5; char initial = 'A'; boolean isStudent = true;
Loops
Loops are used to execute a block of code multiple times. In Java, there are two main types of loops: for
and while
.
For loop
The for
loop has a specific structure:
for (<initialization>; <condition>; <increment>) { // Code to be executed }
Here's an example:
for (int i = 0; i < 5; i++) { System.out.println("Iteration: " + i); }
This loop will output the numbers 0 to 4, as it starts at 0 (int i = 0
), checks if i
is less than 5 (i < 5
), and increments i
by 1 each time (i++
).
While loop
A while
loop will keep running as long as the given condition is true:
while (<condition>) { // Code to be executed }
For example:
int counter = 0; while (counter < 5) { System.out.println("Counter: " + counter); counter++; }
This loop will produce the same output as the for
loop example.
Conditional Statements
Conditional statements help us make decisions in our code based on certain conditions. In Java, we have if
, else if
, and else
statements.
if (<condition>) { // Code to be executed if the condition is true } else if (<anotherCondition>) { // Code to be executed if the first condition is false and this one is true } else { // Code to be executed if none of the conditions are true }
Here's an example:
int grade = 85; if (grade >= 90) { System.out.println("Excellent!"); } else if (grade >= 80) { System.out.println("Great job!"); } else { System.out.println("Keep up the good work!"); }
In this case, since grade
is 85, the output will be "Great job!".
Now that you have a basic understanding of Java syntax, you're all set to dive deeper into the world of programming. Keep honing your skills, and soon you'll be tackling more complex projects and creating amazing applications. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).