Understanding Java Variables and Basic Syntax
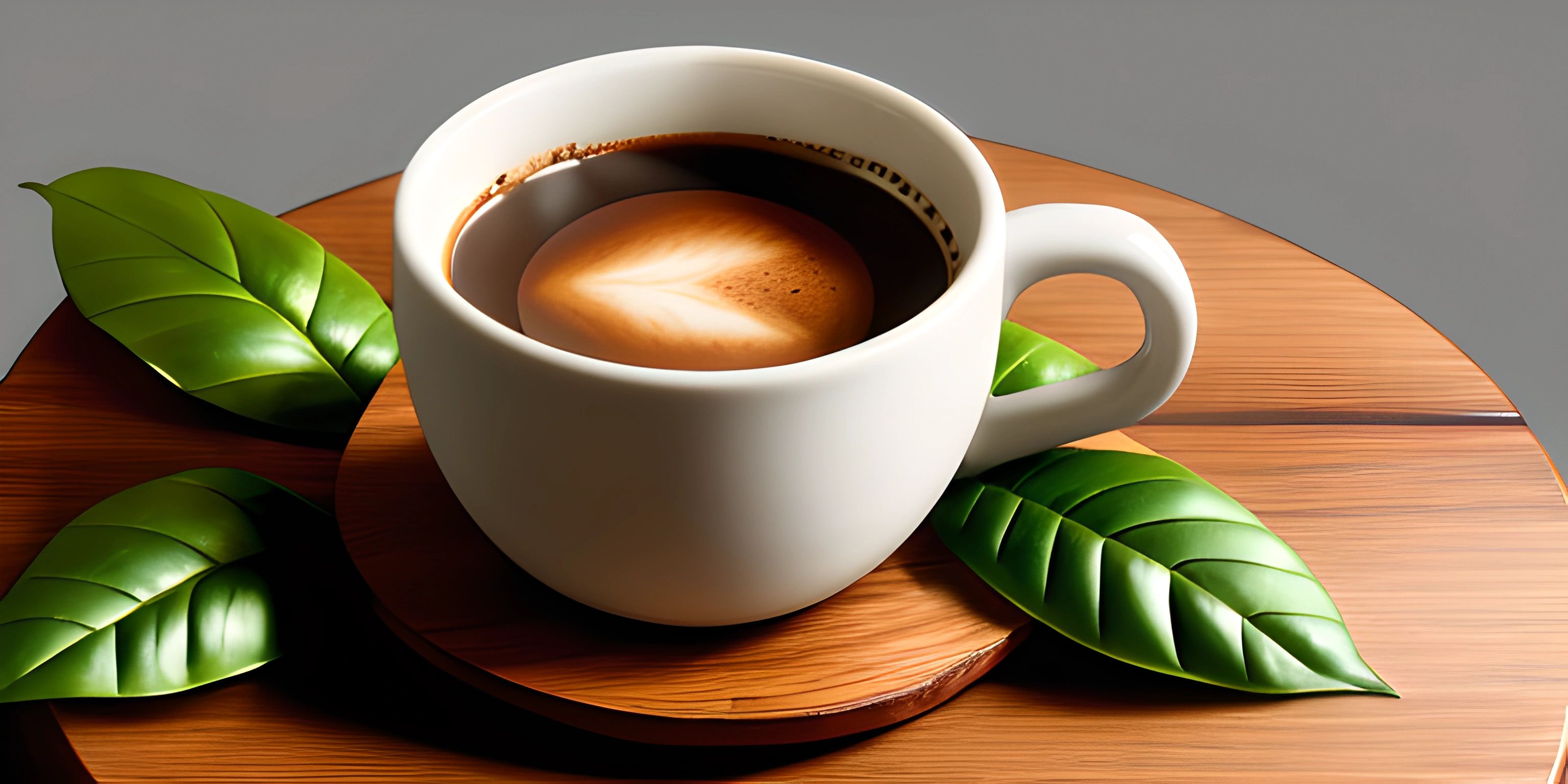
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Diving into the world of Java programming can be exhilarating, but to make the most of it, we need to grasp the basics. In this case, that means understanding Java variables and the language's basic syntax. So let's jump in and explore the foundation of Java!
Java Variables
In Java, variables are the cornerstone for holding and managing data. Consider variables as labeled containers that store different types of information, which can be retrieved, updated, or manipulated later in the program.
Data Types
Java is a strongly-typed language, which means you must specify the type of data a variable can hold. These data types can be broadly categorized into two groups: primitive data types and reference data types.
Primitive data types are the most basic data types available, and include:
byte
: small integers (-128 to 127)short
: medium-sized integers (-32,768 to 32,767)int
: regular-sized integers (-2^31 to 2^31 - 1)long
: large integers (-2^63 to 2^63 - 1)float
: single-precision floating-point numbersdouble
: double-precision floating-point numbersboolean
: true or false valueschar
: single Unicode characters
Reference data types store the memory address of an object or a collection of data, such as arrays, classes, and interfaces.
Variable Declaration
To create a variable in Java, you need to declare it first. Variable declaration follows this pattern:
data_type variable_name;
For example:
int numberOfApples;
Now we have a variable named numberOfApples
that can store integer values.
Variable Assignment
Once a variable is declared, you can assign a value to it using the assignment operator =
:
variable_name = value;
Following our previous example:
numberOfApples = 10;
You can also declare and assign a value to a variable at the same time:
int numberOfApples = 10;
Basic Java Syntax
Now that you know how to create and use variables, let's explore some basic Java syntax rules.
Keywords and Identifiers
Java has a set of reserved words, called keywords, that have special meanings in the language. Examples include int
, class
, public
, and if
. You cannot use these words as variable or method names.
Identifiers are the names you give to Java elements, such as variables, methods, and classes. Identifiers must start with a letter, a dollar sign $
, or an underscore _
, and can contain any combination of letters, numbers, dollar signs, or underscores thereafter.
Statements and Semicolons
In Java, a single line of code that performs an action is called a statement. Statements must end with a semicolon ;
. For example:
int age = 25; age = age + 1; System.out.println("Hello, world!");
Each line is a separate statement, and each ends with a semicolon.
Whitespace and Indentation
Java ignores extra spaces, tabs, and newlines. However, proper use of whitespace and indentation makes your code more readable and organized. The common practice is to use four spaces for each level of indentation:
public class MyClass { public static void main(String[] args) { int x = 5; System.out.println(x); } }
In this example, the contents of the class
and the main
method are indented using four spaces.
Now that you've dipped your toes into Java variables and basic syntax, you're well on your way to mastering the language. Keep practicing, and soon you'll be writing advanced programs with ease!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).