Exploring Java Concurrency and Multithreading
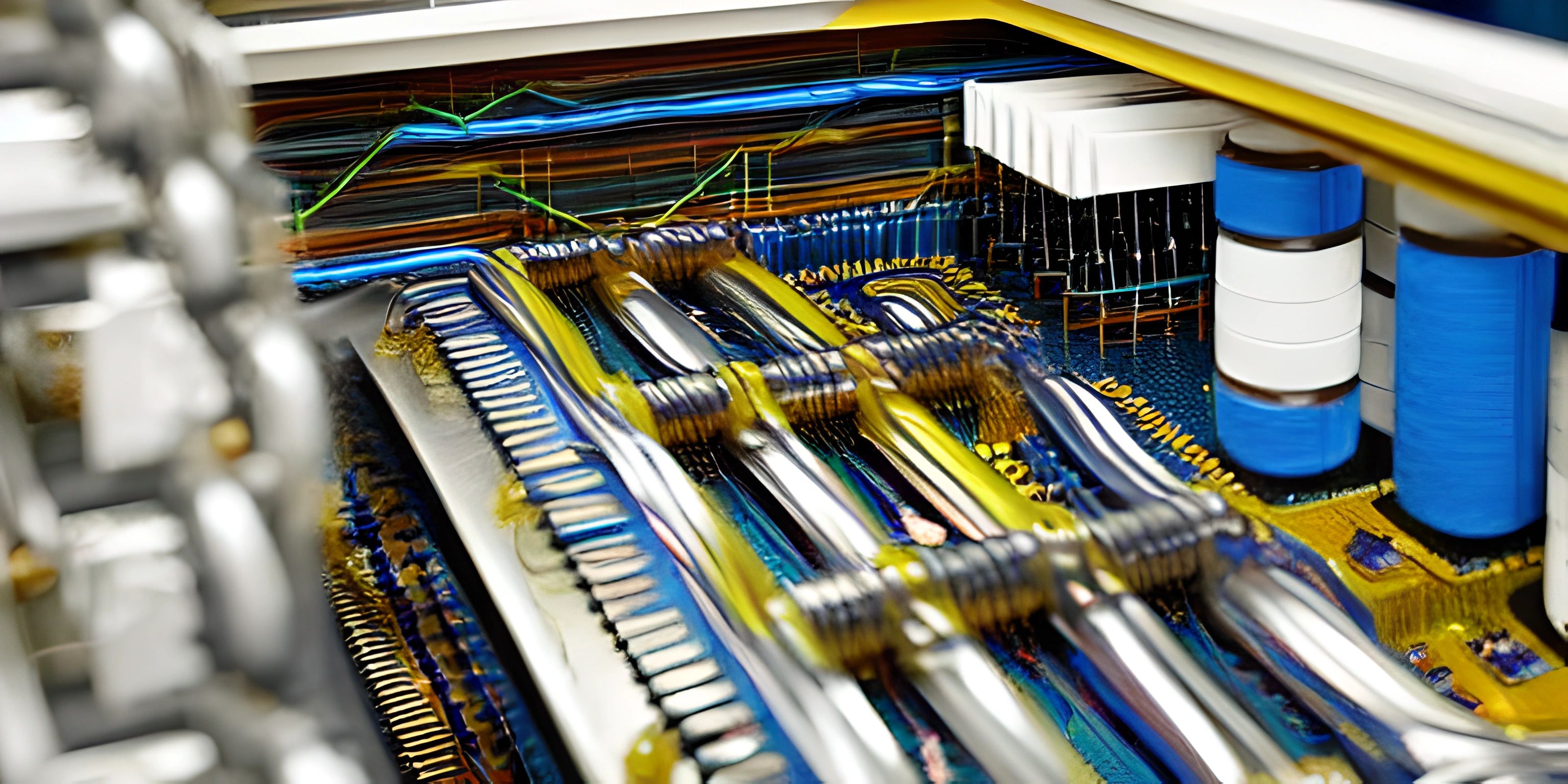
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the realm of Java, one of the key features that sets it apart from other programming languages is its robust support for concurrency and multithreading. These concepts allow you to harness the full power of modern multi-core processors, executing multiple tasks at the same time, and making your programs more efficient and responsive.
Concurrency and Multithreading
Concurrency refers to the ability of a program to manage multiple tasks at the same time. In Java, this is achieved using threads. A thread is a lightweight, independent unit of execution within a program, and a single process can have multiple threads running concurrently. This is known as multithreading.
Creating Threads in Java
There are two main ways to create threads in Java:
- Extending the
Thread
class: You can create a new class that extends theThread
class and override itsrun()
method. Therun()
method contains the code that will be executed when the thread starts.
class MyThread extends Thread { public void run() { System.out.println("Hello from MyThread!"); } }
To start the thread, create an instance of your class and call the start()
method:
MyThread myThread = new MyThread(); myThread.start();
- Implementing the
Runnable
interface: You can create a new class that implements theRunnable
interface and implement itsrun()
method. Then, pass an instance of your class to aThread
object and start the thread.
class MyRunnable implements Runnable { public void run() { System.out.println("Hello from MyRunnable!"); } } Thread thread = new Thread(new MyRunnable()); thread.start();
Both approaches are common, but implementing the Runnable
interface is generally preferred, as it allows your class to extend other classes if needed.
Synchronization and Thread Safety
When multiple threads access shared resources, problems can arise if the resource is not accessed in an orderly fashion. This can lead to unpredictable behavior and hard-to-find bugs, known as race conditions. To avoid these issues, Java provides mechanisms for synchronization and ensuring thread safety.
The synchronized
Keyword
The synchronized
keyword can be used to ensure that only one thread can access a specific method or block of code at a time. When a thread enters a synchronized method or block, it acquires a lock on the object. Other threads that attempt to enter the same method or block will be blocked until the lock is released.
public synchronized void myMethod() { // Code that needs to be synchronized }
Alternatively, you can use a synchronized block:
public void myMethod() { synchronized (this) { // Code that needs to be synchronized } }
The volatile
Keyword
The volatile
keyword is used to indicate that a variable's value may be changed by multiple threads. It ensures that the value of the variable is always read from and written to the main memory, rather than a cached version in a thread's local memory. This can help prevent inconsistencies in the variable's value between different threads.
private volatile int myCounter;
Executors and Thread Pools
Java provides a high-level framework for managing multiple threads called the Executor framework. One of the main components of this framework is the ExecutorService, which allows you to manage a pool of threads and submit tasks to be executed by them.
import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class Main { public static void main(String[] args) { ExecutorService executor = Executors.newFixedThreadPool(4); for (int i = 0; i < 10; i++) { executor.submit(() -> { System.out.println("Hello from thread " + Thread.currentThread().getName()); }); } executor.shutdown(); } }
In this example, we create a fixed-size thread pool with four threads and submit ten tasks. The tasks are distributed among the available threads, and the executor takes care of managing their execution.
These are just the fundamentals of Java concurrency and multithreading. There's a lot more to explore, such as the java.util.concurrent
package, which offers advanced synchronization constructs, atomic variables, and other powerful tools to help you master concurrent programming in Java.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).