Understanding Data Races in Concurrent Programming
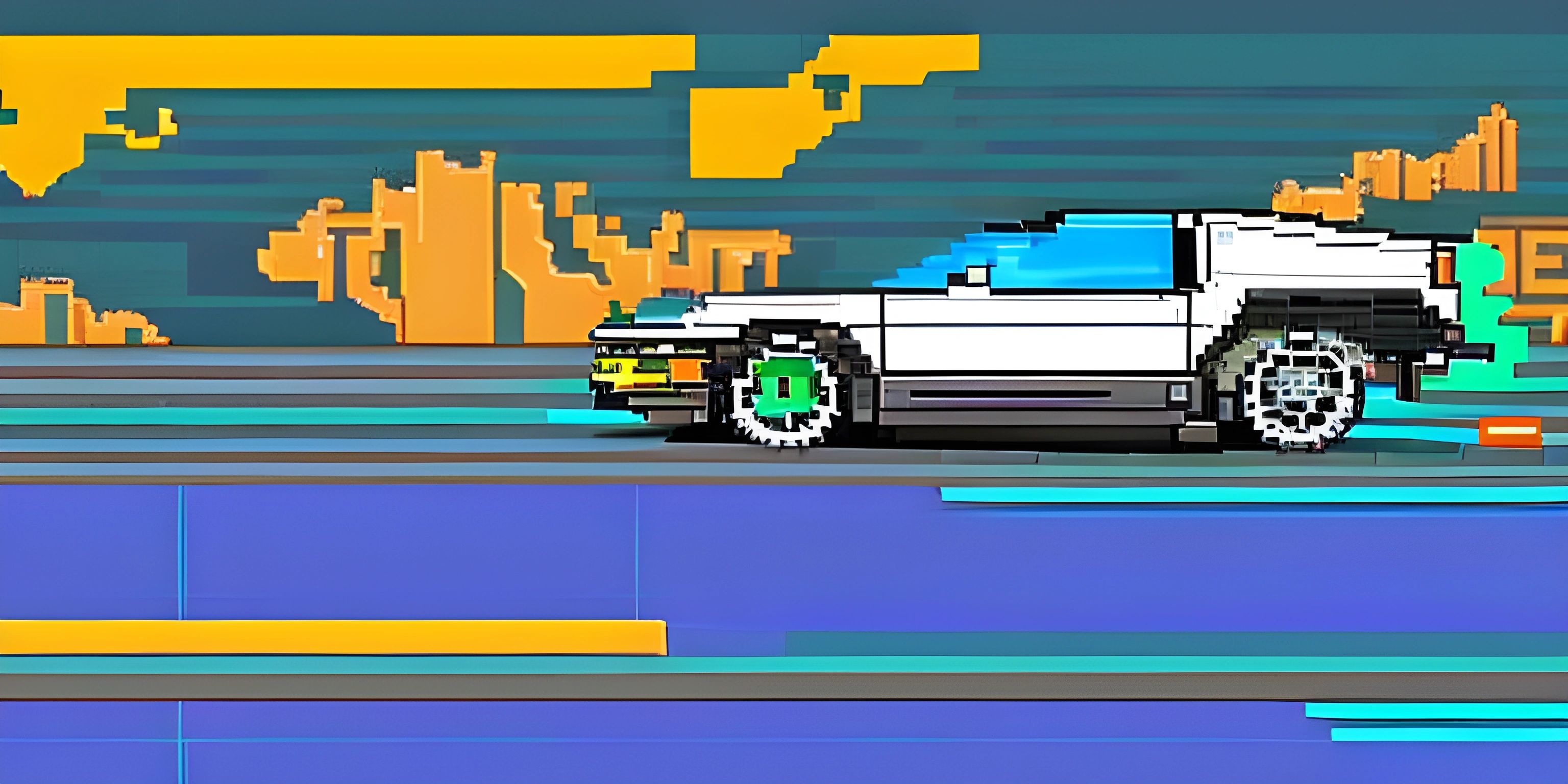
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Picture this: You're at a bustling bakery, with two bakers trying to decorate the same cake simultaneously. One is adding frosting while the other is applying sprinkles. Suddenly, chaos ensues. The bakers' actions conflict, leading to a cake that looks more like a culinary battlefield than a dessert. That's essentially what a data race is in the world of concurrent programming: multiple threads (or bakers) trying to access and modify shared data (the cake) simultaneously, causing unpredictable results.
What is a Data Race?
A data race occurs when two or more threads in a program access shared data at the same time, and at least one of the accesses is a write. This can lead to inconsistent or corrupted data, as the order of operations is not guaranteed. Imagine trying to read a book while someone else is randomly flipping the pages—confusion is inevitable.
Data races are notorious in multi-threaded applications, where threads operate independently and can execute out of order. This is common in languages like Rust and Go, which support concurrent programming models.
Real-World Example
To understand data races better, here's a simple example in Go that demonstrates how a data race can occur:
package main import ( "fmt" "sync" ) func main() { var wg sync.WaitGroup var counter int // Increment function increment := func() { for i := 0; i < 1000; i++ { counter++ } wg.Done() } wg.Add(2) go increment() go increment() wg.Wait() fmt.Println("Final counter:", counter) }
In this example, two goroutines are incrementing a shared variable counter
. Since both goroutines can access counter
at the same time, a data race occurs. The final value of counter
is unpredictable, and running the program multiple times will likely yield different results.
Detecting Data Races
Detecting data races can be tricky, as they may not always manifest themselves in obvious ways. Thankfully, modern languages and tools provide mechanisms to help. For instance, Go's -race
flag can be used to detect data races:
go run -race main.go
Similarly, Rust has built-in features to prevent data races through its ownership system and the borrow checker. Rust ensures that data is either accessed immutably by multiple threads or mutably by a single thread at a time, thus avoiding data races.
Preventing Data Races
Avoiding data races often involves using synchronization mechanisms, such as mutexes, locks, or atomic operations, to control access to shared data.
Using Mutexes
A mutex (short for mutual exclusion) is a synchronization primitive that ensures only one thread can access the critical section of code at a time. Let's rewrite the previous example using a mutex in Go:
package main import ( "fmt" "sync" ) func main() { var wg sync.WaitGroup var counter int var mu sync.Mutex // Increment function with mutex lock increment := func() { for i := 0; i < 1000; i++ { mu.Lock() counter++ mu.Unlock() } wg.Done() } wg.Add(2) go increment() go increment() wg.Wait() fmt.Println("Final counter:", counter) }
By adding a mutex, we ensure that only one goroutine can increment counter
at a time, eliminating the data race.
Using Atomic Operations
Atomic operations are indivisible operations that complete without any interference from other threads. They are useful for simple operations like counters. In Go, we can use the sync/atomic
package:
package main import ( "fmt" "sync" "sync/atomic" ) func main() { var wg sync.WaitGroup var counter int64 // Increment function using atomic operation increment := func() { for i := 0; i < 1000; i++ { atomic.AddInt64(&counter, 1) } wg.Done() } wg.Add(2) go increment() go increment() wg.Wait() fmt.Println("Final counter:", counter) }
Impact of Data Races
Data races can lead to various issues, including:
- Corrupted Data: Inconsistent or unexpected values in your data.
- Crashes: Unexpected crashes or segmentation faults due to invalid memory access.
- Security Vulnerabilities: Exploitable race conditions that can be used to bypass security checks.
Conclusion
Understanding and preventing data races is crucial for writing robust concurrent programs. Using synchronization mechanisms like mutexes, locks, and atomic operations can help ensure that your programs run smoothly without unexpected behavior.
For further reading on concurrency and data races, check out our articles on Rust threading and Go concurrency. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is a data race?
A data race occurs when two or more threads access shared data simultaneously and at least one of the accesses is a write, leading to unpredictable results.
How can I detect data races in my Go program?
You can use the -race
flag when running your Go program to detect data races. For example, go run -race main.go
.
What are some common ways to prevent data races?
Common methods include using mutexes, locks, and atomic operations to control access to shared data and ensure that only one thread can modify the data at a time.
Why are data races problematic?
Data races can lead to corrupted data, program crashes, and even security vulnerabilities, making your program unreliable and potentially exploitable.
How does Rust help prevent data races?
Rust uses its ownership system and borrow checker to ensure that data is either accessed immutably by multiple threads or mutably by a single thread at a time, preventing data races.