Java Multithreading
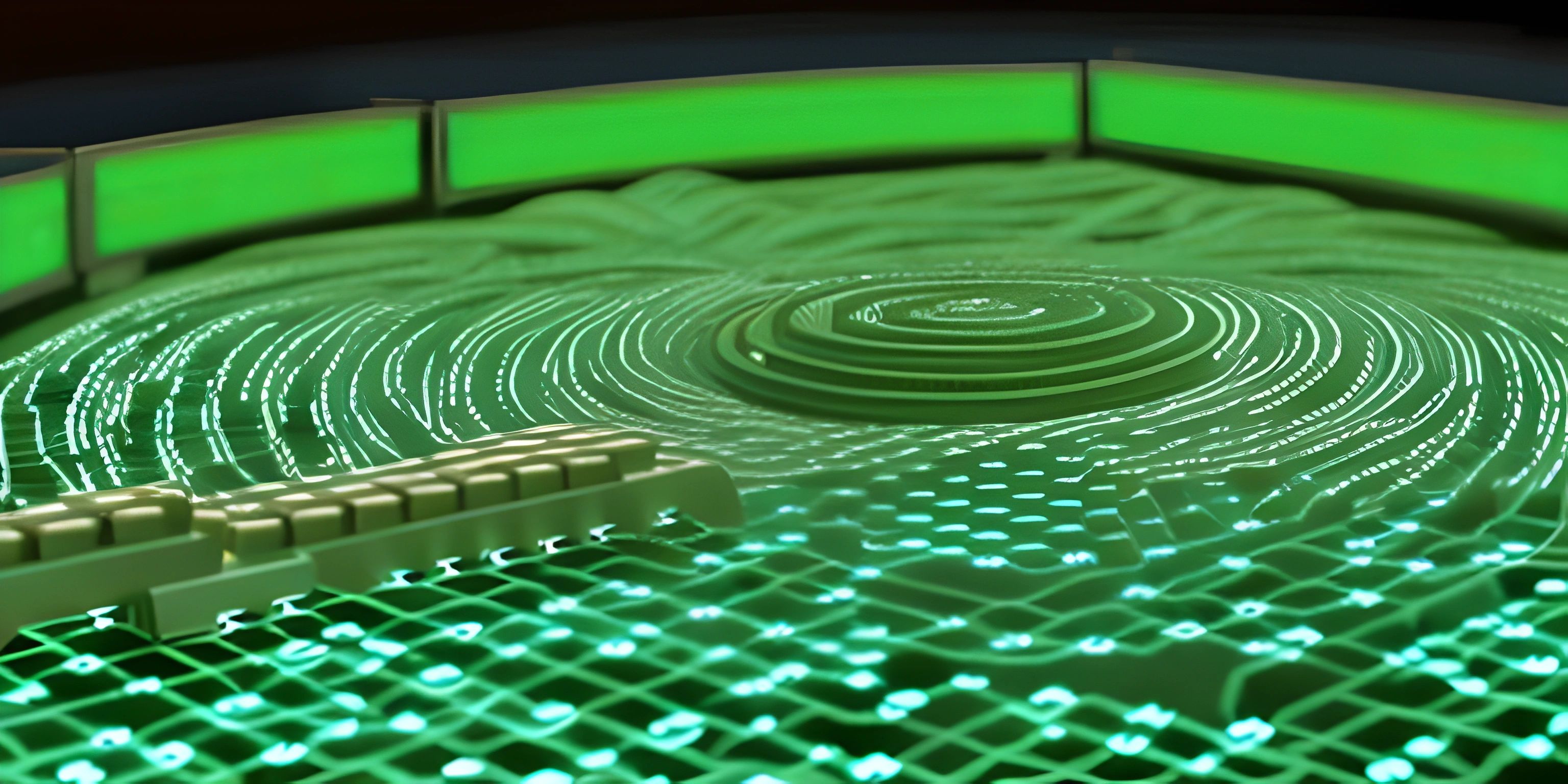
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Multithreading is an essential technique for creating more efficient and responsive programs. In Java, it's relatively simple to implement and can make a world of difference in your applications. Let's dive into the exciting world of Java multithreading!
What is Multithreading?
Multithreading is a form of parallelism in which several independent threads of execution can be run concurrently. This enables your program to perform multiple tasks simultaneously, making it faster and more responsive. In Java, multithreading is supported by the Thread class and the Runnable interface.
Creating Threads in Java
There are two primary ways to create a new thread in Java: extending the Thread
class or implementing the Runnable
interface. Let's take a look at both approaches.
Extending the Thread Class
To create a new thread by extending the Thread
class, you simply need to create a new class that extends Thread
and override the run()
method. The run()
method contains the logic that will be executed in the new thread. Here's an example:
class MyThread extends Thread { public void run() { // Your thread logic here System.out.println("Hello from MyThread!"); } } public class Main { public static void main(String[] args) { MyThread myThread = new MyThread(); myThread.start(); // Start the new thread } }
Implementing the Runnable Interface
Alternatively, you can create a new thread by implementing the Runnable
interface, which requires you to implement the run()
method. Then, you'll need to pass an instance of your Runnable
class to a new Thread
object. Here's an example:
class MyRunnable implements Runnable { public void run() { // Your thread logic here System.out.println("Hello from MyRunnable!"); } } public class Main { public static void main(String[] args) { MyRunnable myRunnable = new MyRunnable(); Thread thread = new Thread(myRunnable); thread.start(); // Start the new thread } }
Both approaches are valid, but implementing the Runnable
interface is generally preferred, as it allows your class to extend another class if needed since Java does not support multiple inheritance.
Synchronization and Thread Safety
When multiple threads access shared resources, there's a risk of data inconsistency or race conditions. To ensure data integrity, it's essential to use synchronization techniques. In Java, you can use the synchronized
keyword to achieve this:
class Counter { private int count = 0; public synchronized void increment() { count++; } public synchronized int getCount() { return count; } } class MyRunnable implements Runnable { private Counter counter; public MyRunnable(Counter counter) { this.counter = counter; } public void run() { counter.increment(); } } public class Main { public static void main(String[] args) throws InterruptedException { Counter counter = new Counter(); Thread[] threads = new Thread[10]; // Start multiple threads for (int i = 0; i < threads.length; i++) { threads[i] = new Thread(new MyRunnable(counter)); threads[i].start(); } // Wait for all threads to finish for (Thread thread : threads) { thread.join(); } System.out.println("Final count: " + counter.getCount()); } }
In this example, the synchronized
keyword ensures that only one thread can access the increment()
and getCount()
methods at a time, preventing race conditions and ensuring data consistency.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is multithreading?
Multithreading is a form of parallelism in which several independent threads of execution can be run concurrently. This enables your program to perform multiple tasks simultaneously, making it faster and more responsive.
How do you create threads in Java?
There are two primary ways to create a new thread in Java: extending the Thread
class or implementing the Runnable
interface. Both approaches require you to define a run()
method containing the logic that will be executed in the new thread.
What is synchronization and why is it important in multithreading?
Synchronization is a technique used to prevent data inconsistency and race conditions when multiple threads access shared resources. It ensures that only one thread can access the synchronized resource or method at a time, maintaining data integrity and consistency.