Rust Threading
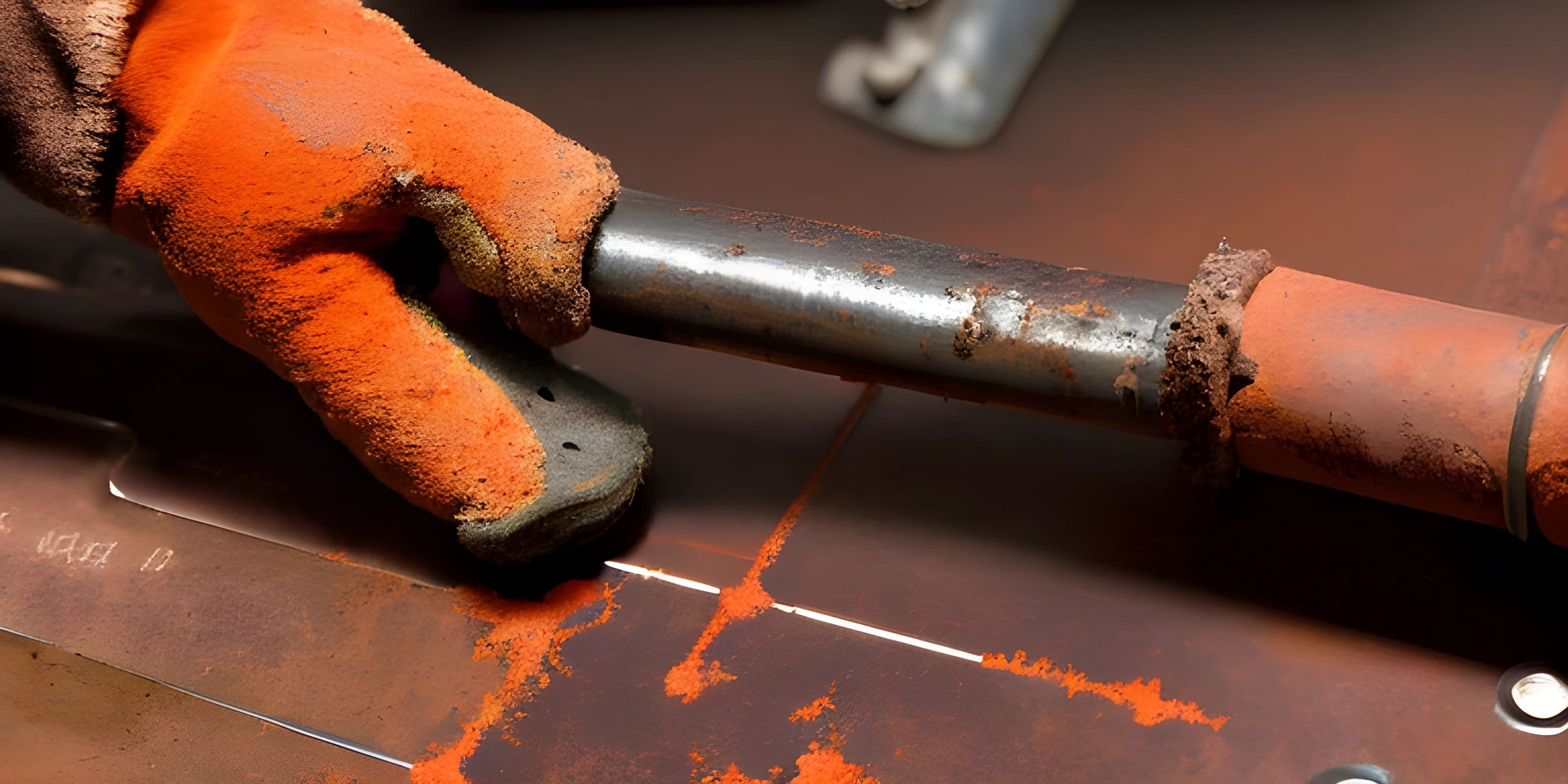
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Before diving into the world of Rust threading, it's important to understand what threads are, and how they help in managing concurrent and parallel execution. Just like a loom weaves multiple threads together to create a beautiful tapestry, the Rust programming language uses threads to elegantly manage multiple tasks running at the same time.
Threads in Rust
In Rust, threads are created using the std::thread
module. This module provides a way to spawn new threads and manage their execution. The main thread is where the program starts by default, but you can easily create additional threads to run tasks concurrently.
Here's a simple example to demonstrate spawning a new thread:
use std::thread; use std::time::Duration; fn main() { thread::spawn(|| { for i in 1..10 { println!("Hello from spawned thread #{}", i); thread::sleep(Duration::from_millis(1)); } }); for i in 1..5 { println!("Hello from main thread #{}", i); thread::sleep(Duration::from_millis(1)); } }
In this example, we create a new thread using thread::spawn
and pass it a closure containing the code we want to run. The spawned thread will execute the closure while the main thread continues to run its own code. Both threads will print messages and sleep for a short duration.
Notice that the output may vary each time you run the program, as the order in which the threads execute is not guaranteed.
Join Handles
When you spawn a thread, it returns a JoinHandle
. A JoinHandle
can be used to wait for the thread to finish before proceeding. This is useful when you need to ensure that a certain task is completed before moving forward. Here's an example:
use std::thread; fn main() { let handle = thread::spawn(|| { for i in 1..10 { println!("Hello from spawned thread #{}", i); } }); handle.join().unwrap(); // Wait for the spawned thread to finish for i in 1..5 { println!("Hello from main thread #{}", i); } }
In this example, we use the join()
method on the JoinHandle
to wait for the spawned thread to complete before the main thread continues its execution. This ensures that all messages from the spawned thread are printed before the main thread's messages.
Sharing Data Between Threads
Sharing data between threads can be a bit tricky due to Rust's strict safety rules. To share data, you can use Arc
(Atomic Reference Counting) and Mutex
(Mutual Exclusion) from the std::sync
module. These tools ensure that data is accessed safely and prevent race conditions.
Here's an example of sharing data between two threads:
use std::sync::{Arc, Mutex}; use std::thread; fn main() { let counter = Arc::new(Mutex::new(0)); let mut handles = vec![]; for _ in 0..10 { let counter = Arc::clone(&counter); let handle = thread::spawn(move || { let mut num = counter.lock().unwrap(); *num += 1; }); handles.push(handle); } for handle in handles { handle.join().unwrap(); } println!("Final counter value: {}", *counter.lock().unwrap()); }
In this example, we create a counter wrapped in an Arc<Mutex<_>>
. We then spawn 10 threads that each increment the counter. By using Arc
and Mutex
, we can safely share and mutate the data across multiple threads.
Now you have a solid foundation in Rust threading! You can use these concepts to create efficient, concurrent, and parallel programs that make the best use of your system's resources. Happy weaving!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is threading in Rust?
Threading in Rust refers to the process of running multiple threads within a program, allowing for concurrent and parallel execution. Rust provides a rich API for creating, managing, and synchronizing threads, which helps developers make better use of system resources and improve overall performance.
How do I create a new thread in Rust?
To create a new thread in Rust, you can use the spawn
function from the std::thread
module. This function takes a closure that is executed in a separate thread. Here's an example:
use std::thread; fn main() { let handle = thread::spawn(|| { println!("Hello from the new thread!"); }); handle.join().unwrap(); println!("Hello from the main thread!"); }
How can I share data between threads in Rust?
Rust offers several ways to safely share data between threads, one of the most common being Arc
(Atomic Reference Counting) and Mutex
(Mutual Exclusion). You can wrap your shared data in an Arc<Mutex<T>>
where T is the type of your data, to ensure thread-safety and prevent data races. Here's an example:
use std::sync::{Arc, Mutex}; use std::thread; fn main() { let counter = Arc::new(Mutex::new(0)); let mut handles = vec![]; for _ in 0..10 { let counter = Arc::clone(&counter); let handle = thread::spawn(move || { let mut num = counter.lock().unwrap(); *num += 1; }); handles.push(handle); } for handle in handles { handle.join().unwrap(); } println!("Result: {}", *counter.lock().unwrap()); }
What is the difference between concurrency and parallelism?
Concurrency refers to the ability of a program to manage multiple tasks at the same time, even if they don't run simultaneously. Parallelism, on the other hand, is the execution of multiple tasks simultaneously, usually achieved by distributing tasks across multiple CPU cores or processors. Threading in Rust enables both concurrent and parallel execution, depending on the system resources and the way developers structure their programs.
How do I control the maximum number of threads in a Rust program?
In Rust, you can control the maximum number of threads by using a thread pool. The rayon
crate provides a simple and efficient thread pool implementation that automatically manages the number of threads based on your system's resources. To use rayon
, add it to your Cargo.toml
file and then use the par_iter
or par_iter_mut
methods provided by the rayon::prelude::*
for parallel iterations. Here's an example:
[dependencies] rayon = "1.5.1"
use rayon::prelude::*; fn main() { let mut data = vec![1, 2, 3, 4, 5]; data.par_iter_mut().for_each(|num| { *num *= 2; }); println!("{:?}", data); }