Java Concurrency API
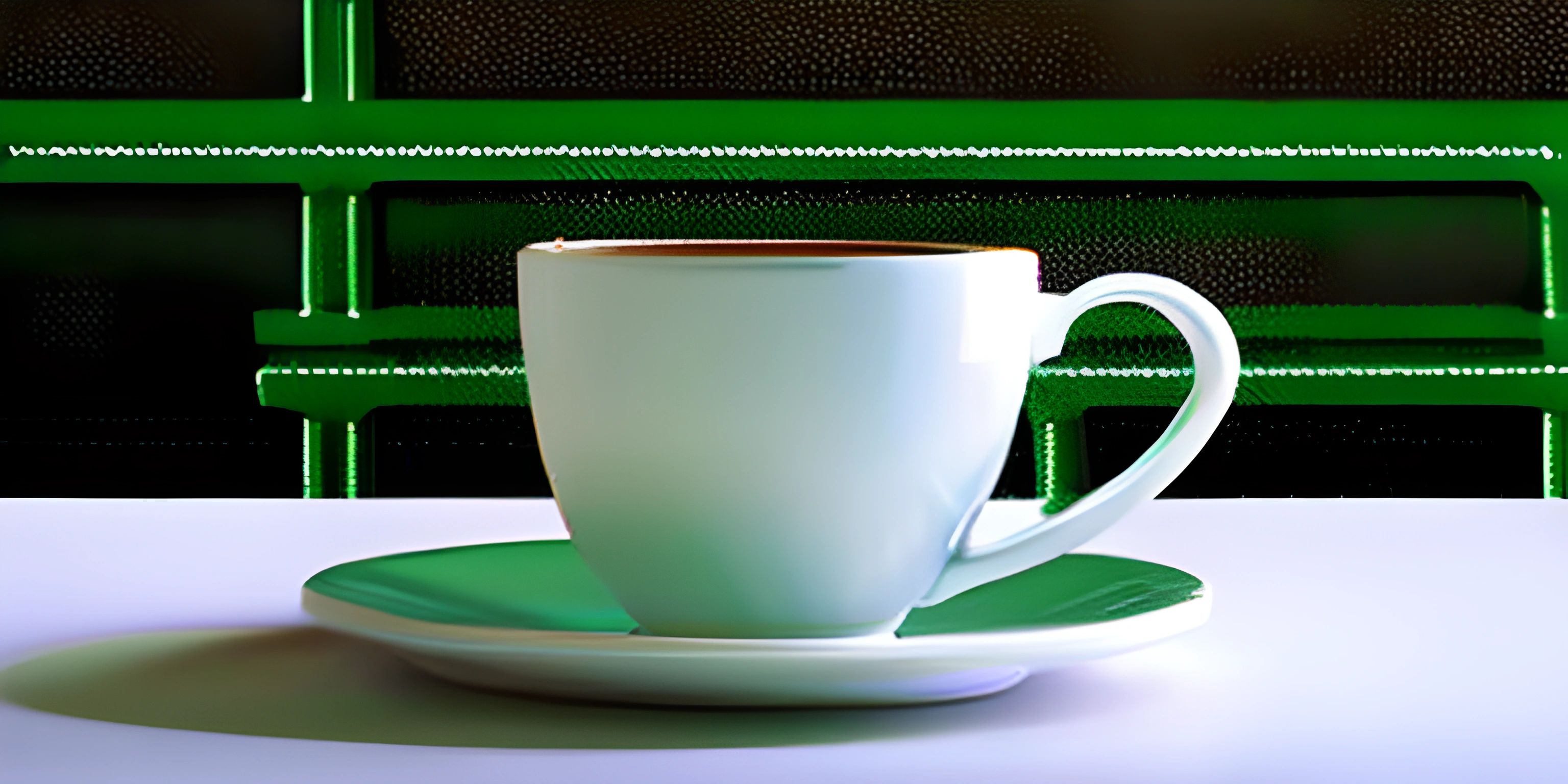
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Concurrency is the ability of a program to execute multiple tasks simultaneously. In the world of Java, efficiently managing concurrent tasks is crucial for creating high-performance applications. That's where the Java Concurrency API comes into play.
Java Concurrency API Overview
The Java Concurrency API provides a set of high-level tools and utilities for managing concurrent tasks. It includes a variety of components like:
- Executors: Manage thread creation and execution.
- ThreadPool: Manage a pool of reusable threads.
- Synchronization constructs: Synchronize access to shared resources.
- Atomic variables: Perform atomic operations on variables.
- Concurrent collections: Thread-safe versions of regular data structures.
Let's dive into each of these components and explore their capabilities.
Executors
Executors, also known as the Executor framework, are a powerful abstraction over the traditional Thread class. They provide a cleaner and more efficient way to manage thread creation and execution.
The Executor
interface is the foundation of this framework and contains a single method: execute(Runnable)
. Implementations of this interface manage the execution of tasks, represented by the Runnable
objects, without the need to create and manage threads directly.
Here's a simple example:
Executor executor = Executors.newSingleThreadExecutor(); executor.execute(() -> System.out.println("Hello from a thread managed by an Executor"));
ThreadPool
A ThreadPool
is a pool of threads that can be reused to execute tasks. It manages thread creation, recycling, and termination, which helps reduce the overhead associated with thread management.
Java provides several built-in thread pool implementations through the Executors
class, such as:
newFixedThreadPool(int n)
: Creates a fixed-size thread pool withn
threads.newCachedThreadPool()
: Creates a thread pool that creates new threads as needed but reuses previously created threads if they are available.newScheduledThreadPool(int n)
: Creates a thread pool that can schedule tasks to run after a given delay or periodically.
Here's an example using a fixed thread pool:
ExecutorService executorService = Executors.newFixedThreadPool(4); executorService.submit(() -> System.out.println("Task 1")); executorService.submit(() -> System.out.println("Task 2")); executorService.shutdown();
Synchronization Constructs
Java provides several synchronization constructs to coordinate access to shared resources among multiple threads. Some of the most commonly used constructs include:
synchronized
: A keyword that enforces mutual exclusion on a block of code or method.Lock
: An interface that provides more flexible locking mechanisms than thesynchronized
keyword.ReadWriteLock
: An interface for managing separate locks for read and write access.Semaphore
: A counting semaphore to control access to a shared resource.CountDownLatch
: A synchronization aid that allows one or more threads to wait until a set of operations complete.
For example, using a ReentrantLock
for mutual exclusion:
Lock lock = new ReentrantLock(); lock.lock(); try { // Critical section } finally { lock.unlock(); }
Atomic Variables
Atomic variables, provided by the java.util.concurrent.atomic
package, are used to perform atomic operations on variables, ensuring that multiple threads can access and modify them safely without the need for synchronization.
Some common atomic variables include:
AtomicInteger
AtomicLong
AtomicReference
An example using an AtomicInteger
:
AtomicInteger counter = new AtomicInteger(0); counter.incrementAndGet(); // Atomically increments the value
Concurrent Collections
Java provides thread-safe versions of regular collections called concurrent collections. They minimize synchronization overhead and offer better performance than their synchronized counterparts.
Some common concurrent collections include:
ConcurrentHashMap
CopyOnWriteArrayList
CopyOnWriteArraySet
ConcurrentSkipListSet
A ConcurrentHashMap
example:
ConcurrentMap<String, Integer> concurrentMap = new ConcurrentHashMap<>(); concurrentMap.put("Key", 42);
In conclusion, the Java Concurrency API provides a powerful and flexible set of tools to manage concurrent tasks. By leveraging its features, you can write efficient and scalable applications that can handle the complexities of concurrency with ease.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is the Java Concurrency API?
The Java Concurrency API is a set of classes and interfaces provided by the Java platform that allows developers to manage and control concurrent tasks seamlessly. It provides powerful tools to perform complex, multi-threaded operations with ease, such as creating and managing multiple threads, handling synchronization, and dealing with deadlocks.
How does the Java Concurrency API help in managing concurrent tasks?
The Java Concurrency API simplifies the management of concurrent tasks by providing higher-level abstractions for dealing with threads and their synchronization. Some of its key features include:
- Executors: These are used to manage thread pools and simplify the process of creating and assigning tasks to threads.
- Callables and Futures: They help in returning results from concurrent tasks and managing their completion.
- Locks and synchronization utilities: These help in controlling access to shared resources and avoiding race conditions.
What are Executors in the Java Concurrency API?
Executors are part of the Java Concurrency API that provide a higher-level replacement for working directly with threads. They make it easier to manage thread pools, create and assign tasks to threads, and manage their lifecycle. Executors offer a more flexible way of handling concurrency, enabling developers to focus on the logic of their tasks instead of dealing with low-level threading details.
Can you provide an example of using an Executor in Java?
Sure! Here's a simple example of using an Executor to run a task in a separate thread:
import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class ExecutorExample { public static void main(String[] args) { ExecutorService executor = Executors.newSingleThreadExecutor(); Runnable task = () -> { System.out.println("Hello, I'm running in a separate thread!"); }; executor.execute(task); executor.shutdown(); } }
In this example, an ExecutorService
is created with a single thread, and the Runnable
task is executed using that thread. Finally, we call shutdown()
to gracefully terminate the executor after the task is completed.
What are Callables and Futures in the Java Concurrency API?
Callables and Futures are part of the Java Concurrency API that allows you to work with tasks that return results and need to be managed upon completion. Callable is an interface representing a task that returns a value and can throw an exception. Future is an interface representing the result of a computation that might not have completed yet. When you submit a Callable task to an Executor, you get a Future object, which can be used to check the status of the task, retrieve the result when it's available, or even cancel the task if necessary. This approach provides a more flexible way to handle the results of concurrent tasks.