Threads in Java
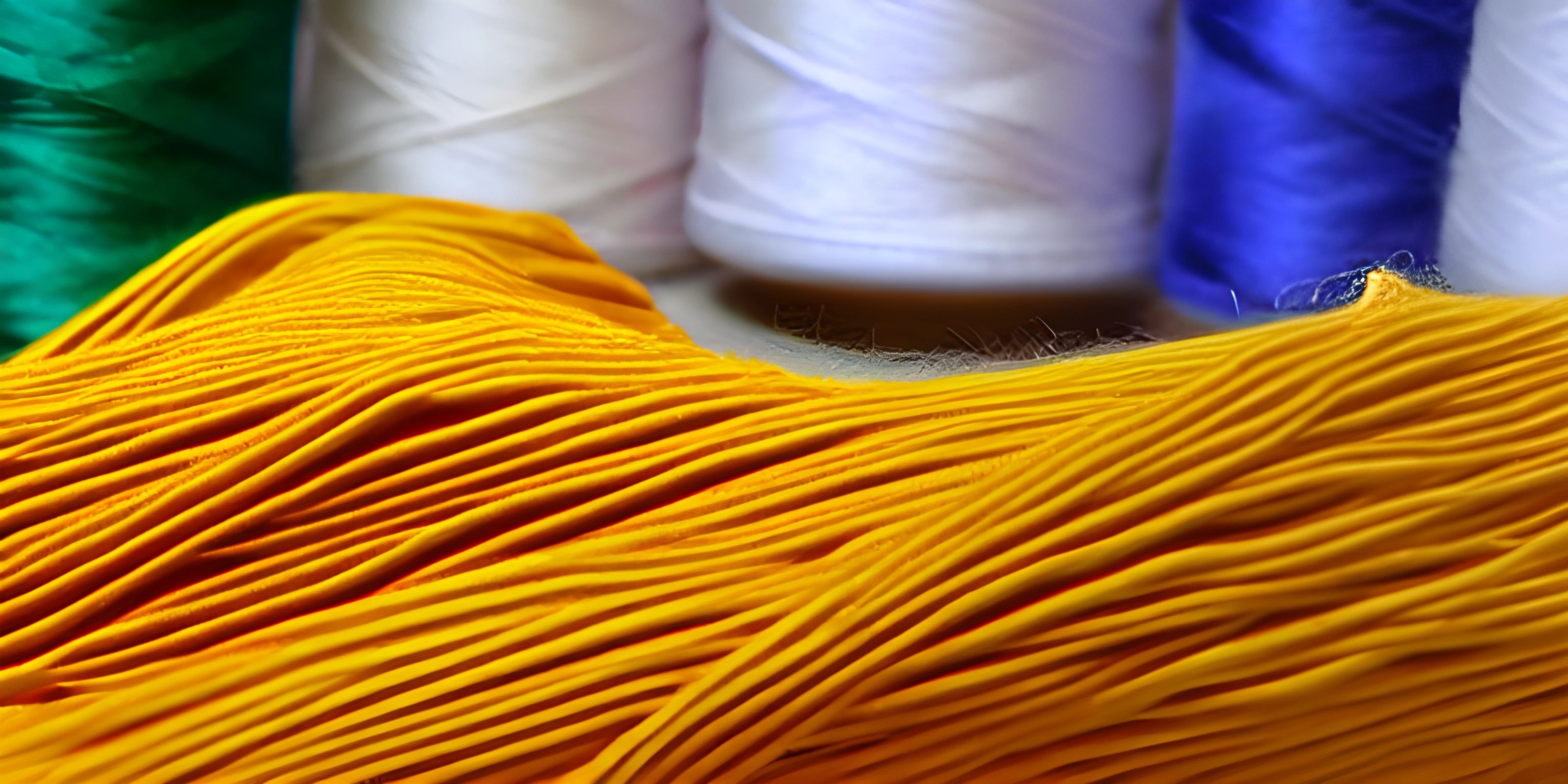
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Concurrency is a powerful tool in the world of programming, allowing multiple tasks to be executed simultaneously, improving the performance of your applications. In Java, threads play a crucial role in achieving concurrency, and they are essential for creating efficient and responsive applications.
What are Threads?
Threads are lightweight, independent units of execution within a process. They consist of a program counter, a stack, and a set of registers. In Java, threads are represented as objects of the Thread
class, which is part of the java.lang
package. Multiple threads can operate concurrently within a single process, sharing resources such as memory and file handles.
Main Thread
When you run a Java application, it starts with a single thread known as the main thread. This thread executes the main
method, which acts as the entry point for your application. The main thread is responsible for creating and managing other threads within the application.
Creating Threads in Java
There are two primary ways to create threads in Java:
-
Extending the
Thread
class: You can create a new class that extends theThread
class and override itsrun
method. Therun
method contains the code that will be executed when the thread starts.class MyThread extends Thread { public void run() { System.out.println("Hello from MyThread!"); } } public class Main { public static void main(String[] args) { MyThread myThread = new MyThread(); myThread.start(); } }
-
Implementing the
Runnable
interface: Alternatively, you can create a class that implements theRunnable
interface and pass an instance of this class to theThread
constructor.class MyRunnable implements Runnable { public void run() { System.out.println("Hello from MyRunnable!"); } } public class Main { public static void main(String[] args) { Thread myThread = new Thread(new MyRunnable()); myThread.start(); } }
Both approaches are valid, but implementing the Runnable
interface is generally preferred, as it allows your class to extend another class if needed (since Java does not support multiple inheritance).
Thread States and Lifecycle
A thread in Java can be in one of several states during its lifecycle:
- New: The thread has been created but has not yet started.
- Runnable: The thread is ready to run and is waiting for the scheduler to pick it up.
- Running: The thread is currently executing its
run
method. - Blocked: The thread is waiting for a resource or a monitor lock to become available.
- Waiting: The thread is waiting indefinitely for another thread to perform a particular action.
- Timed Waiting: The thread is waiting for a specified period of time.
- Terminated: The thread has completed its execution.
Understanding these states is essential for effectively managing threads in your applications.
Synchronization and Thread Safety
In a multithreaded environment, ensuring that shared resources are accessed safely is crucial. Java provides the synchronized
keyword to achieve thread safety through mutual exclusion. You can use the synchronized
keyword with methods or blocks to ensure that only one thread can execute the critical section at a time.
class Counter { private int count; public synchronized void increment() { count++; } public synchronized int getCount() { return count; } }
In this example, the increment
and getCount
methods are synchronized, ensuring that only one thread can access the shared count
variable at a time.
By understanding and effectively using threads in Java, you can create applications that perform multiple tasks concurrently, delivering improved performance and responsiveness.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What are threads in Java?
Threads are lightweight, independent units of execution within a process. In Java, they are represented as objects of the Thread
class. Threads allow multiple tasks to be executed concurrently, improving the performance and responsiveness of applications.
How can I create a thread in Java?
You can create a thread in Java either by extending the Thread
class and overriding its run
method, or by implementing the Runnable
interface and passing an instance of your class to the Thread
constructor. Implementing the Runnable
interface is generally preferred, as it allows your class to extend another class if needed.
What are the various states of a thread in Java?
A thread in Java can be in one of several states: New, Runnable, Running, Blocked, Waiting, Timed Waiting, and Terminated. These states are essential for effectively managing threads in your applications.
How can I ensure thread safety in Java?
You can ensure thread safety in Java by using the synchronized
keyword with methods or blocks. This ensures that only one thread can execute the critical section at a time, preventing concurrent access to shared resources.