Rust Concurrency
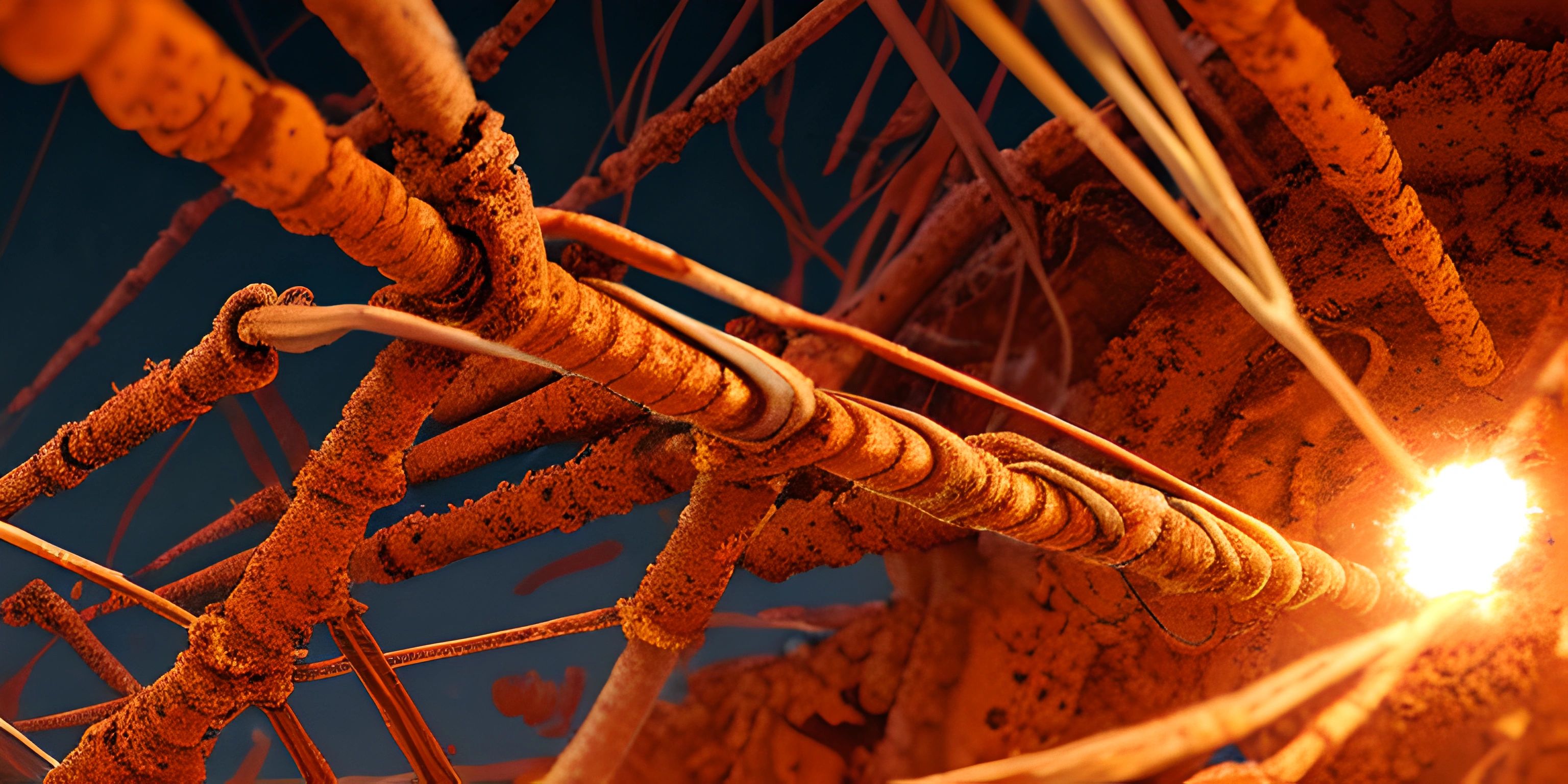
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Concurrency is a powerful concept that allows programs to run multiple tasks simultaneously. Rust offers an efficient and safe way to handle concurrency, minimizing the risk of race conditions and other bugs. This article will explore the concurrency features and patterns in Rust, such as threads, channels, and shared state.
Threads
In Rust, the primary method to achieve concurrency is through threads. Threads are lightweight, independent units of execution that can run in parallel. Rust's standard library provides the std::thread
module which allows you to create and manage threads.
Creating Threads
To create a new thread in Rust, you can use the thread::spawn
function. This function takes a closure as an argument and runs it in a new thread. Here's an example:
use std::thread; fn main() { let handle = thread::spawn(|| { println!("Hello from a new thread!"); }); handle.join().unwrap(); println!("Hello from the main thread!"); }
In this example, we create a new thread that prints "Hello from a new thread!" and then wait for it to finish using the join
method on the thread handle. After the new thread is done, the main thread prints "Hello from the main thread!".
Joining Threads
As seen above, the join
method can be used to wait for a thread to finish. This is useful when you need the main thread or another thread to wait for the spawned thread's result or completion. It's important to note that the join
method returns a Result
type, which can be used to handle any errors that occurred during the execution of the spawned thread.
Channels
While threads are useful for running tasks concurrently, they often need to communicate with each other. Rust provides channels to facilitate this communication in a safe and efficient manner. Channels in Rust are based on the multiple-producer, single-consumer (MPSC) model, which allows multiple senders to send messages to a single receiver.
Creating Channels
You can create a channel in Rust using the std::sync::mpsc::channel
function. This function returns a tuple containing a sender and a receiver. The sender can be cloned to create multiple producers, while the receiver remains unique. Here's an example of creating a channel and sending a message:
use std::sync::mpsc; use std::thread; fn main() { let (tx, rx) = mpsc::channel(); let thread_handle = thread::spawn(move || { let data = "Hello from the spawned thread!"; tx.send(data).unwrap(); }); let received = rx.recv().unwrap(); println!("Received: {}", received); thread_handle.join().unwrap(); }
In this example, we create a channel with a sender tx
and a receiver rx
. We then spawn a new thread that sends a message through the channel. In the main thread, we wait for the message using the recv
method on the receiver and print it.
Shared State
Another method of communication between threads is by sharing state. Rust provides several synchronization primitives for this purpose, such as Mutex
and RwLock
. These primitives ensure that only one thread can access the shared state at a time, preventing data races.
Mutex
A Mutex
(short for "mutual exclusion") is a synchronization primitive that ensures only one thread can access a piece of data at a time. You can create a Mutex
by wrapping your data in the std::sync::Mutex
type. Here's an example of using a Mutex
to protect shared state:
use std::sync::{Arc, Mutex}; use std::thread; fn main() { let counter = Arc::new(Mutex::new(0)); let mut handles = vec![]; for _ in 0..10 { let counter = Arc::clone(&counter); let handle = thread::spawn(move || { let mut num = counter.lock().unwrap(); *num += 1; }); handles.push(handle); } for handle in handles { handle.join().unwrap(); } println!("Counter: {}", *counter.lock().unwrap()); }
In this example, we create a shared counter protected by a Mutex
. We then spawn 10 threads, each incrementing the counter by 1. After all threads have finished, we print the final value of the counter.
By using Rust's concurrency features and patterns, such as threads, channels, and shared state, you can create powerful and efficient concurrent programs while minimizing the risk of race conditions and other bugs. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
How does Rust handle concurrency?
Rust offers an excellent concurrency model, focusing on three main concepts: threads, channels, and shared state. Rust's threads are similar to those in other languages, allowing developers to execute different parts of code concurrently. Channels facilitate communication between threads, while shared state deals with sharing data between threads using synchronization primitives like Mutex
and Arc
.
Can you provide an example of creating a thread in Rust?
Sure! Here's a simple example of how to create a new thread in Rust:
use std::thread; use std::time::Duration; fn main() { let handle = thread::spawn(|| { for i in 1..10 { println!("Hello from spawned thread, count: {}", i); thread::sleep(Duration::from_millis(1)); } }); for i in 1..5 { println!("Hello from main thread, count: {}", i); thread::sleep(Duration::from_millis(1)); } handle.join().unwrap(); }
In this example, we spawn a new thread using thread::spawn
and use a closure to define the code to be executed in the spawned thread. The main thread and the spawned thread both print messages and use thread::sleep
to simulate some work. The handle.join().unwrap()
call ensures that the main thread waits for the spawned thread to finish before exiting.
How do I share data between threads in Rust?
You can share data between threads in Rust using channels and shared state. Channels are used for message-passing between threads, while shared state involves sharing data through synchronization primitives like Mutex
and Arc
. Here's an example using a channel to share data between a producer and a consumer thread:
use std::sync::mpsc; use std::thread; fn main() { let (tx, rx) = mpsc::channel(); let producer = thread::spawn(move || { for i in 1..10 { tx.send(i).unwrap(); } }); let consumer = thread::spawn(move || { while let Ok(data) = rx.recv() { println!("Received data: {}", data); } }); producer.join().unwrap(); consumer.join().unwrap(); }
In this example, we create a channel using mpsc::channel()
, which returns a pair of sender (tx
) and receiver (rx
). The producer thread sends data to the channel, and the consumer thread receives and processes the data.
What is the role of Mutex and Arc in Rust concurrency?
Mutex
and Arc
are synchronization primitives provided by Rust's standard library that help with sharing data between threads safely.
Mutex
(short for "mutual exclusion") ensures that only one thread can access the data at a time. When a thread wants to access the data, it needs to acquire a lock on theMutex
. If another thread already holds the lock, the requesting thread will have to wait until the lock is released.Arc
(short for "atomic reference counting") is a shared ownership smart pointer that allows multiple threads to have read access to the same data, while ensuring that the data is deallocated when no longer needed. It is particularly useful when dealing with shared state across threads. UsingMutex
andArc
together allows you to share mutable data safely between multiple threads. For example:
use std::sync::{Arc, Mutex}; use std::thread; fn main() { let counter = Arc::new(Mutex::new(0)); let mut handles = vec![]; for _ in 0..10 { let counter = Arc::clone(&counter); let handle = thread::spawn(move || { let mut num = counter.lock().unwrap(); *num += 1; }); handles.push(handle); } for handle in handles { handle.join().unwrap(); } println!("Result: {}", *counter.lock().unwrap()); }
In this example, we use Mutex
to protect the shared counter
and wrap it in an Arc
to allow multiple threads to access it simultaneously. The threads increment the counter, and the final value is printed in the main thread after all spawned threads have finished their work.