JUnit Introduction
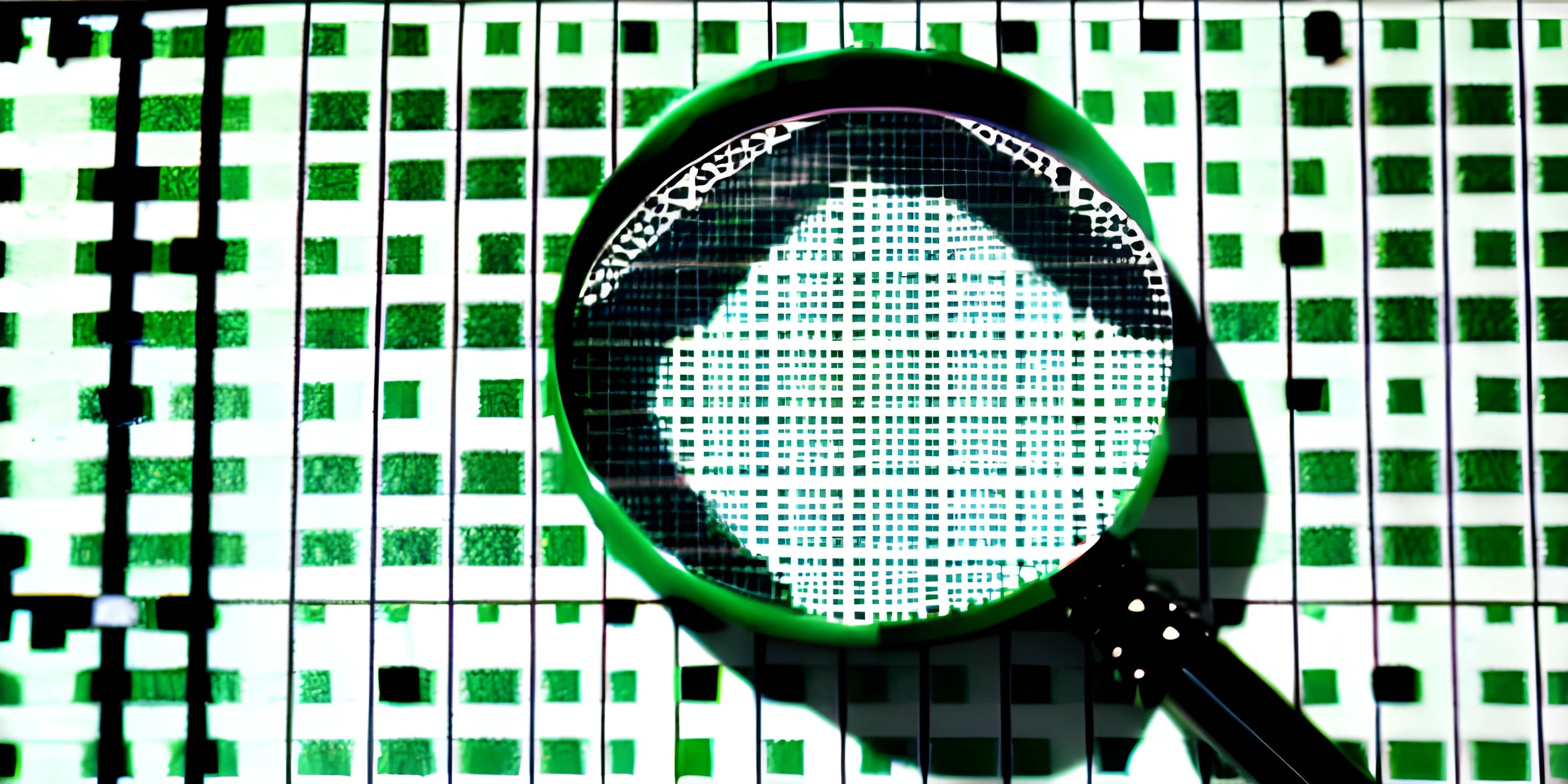
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Testing is a crucial part of software development processes, ensuring that your code is working as intended and catching bugs early on. One of the most popular testing frameworks for Java is JUnit, which simplifies the process of writing and running tests.
What is JUnit?
JUnit is an open-source testing framework for Java applications. It provides a set of annotations and assertions that allow you to write and run tests for your code easily. JUnit has become a standard in the industry for unit testing Java applications, thanks to its simplicity, wide adoption, and integration with various development tools.
Why Use JUnit?
JUnit offers several advantages that make it an attractive choice for testing Java applications:
-
Simplicity: JUnit is easy to learn and use, even for beginners. Its straightforward syntax and clear documentation make writing tests a breeze.
-
Extensibility: JUnit is designed to be extensible, allowing you to create custom test runners, reporters, and more.
-
Integration: JUnit is well-integrated with popular Java IDEs and build tools, such as Eclipse, IntelliJ IDEA, and Maven. This makes it easy to include JUnit tests in your development workflow.
-
Community Support: JUnit has a large and active community that provides support, plugins, and additional resources.
Getting Started with JUnit
To use JUnit, you'll need to add it as a dependency in your project. If you're using a build tool like Maven or Gradle, you can simply include the JUnit dependency in your build file. For example, in a Maven pom.xml
file:
<dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.13.2</version> <scope>test</scope> </dependency> </dependencies>
Once you have JUnit set up, you can start writing tests. JUnit tests are simple Java classes that use annotations and assertions to define test cases. Here's a basic example:
import org.junit.Test; import static org.junit.Assert.assertEquals; public class MyTests { @Test public void testAddition() { int a = 2; int b = 3; int expected = 5; assertEquals("2 + 3 should equal 5", expected, a + b); } }
In this example, we import the necessary JUnit classes, create a test class (MyTests), and define a test method (testAddition) using the @Test
annotation. Inside the test method, we use the assertEquals
assertion to verify that the sum of a
and b
equals expected
.
To run your JUnit tests, you can use your IDE's built-in test runner, or you can run them from the command line using a build tool like Maven or Gradle.
Conclusion
JUnit is a powerful and widely-used testing framework for Java applications. Its simplicity and integration with development tools make it an excellent choice for Java developers looking to improve their code quality through testing. By incorporating JUnit into your workflow, you can catch bugs early, reduce development time, and ensure that your code is robust and reliable.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: AI Test Playground (psst, it's free!).
FAQ
What is JUnit?
JUnit is an open-source testing framework for Java applications. It provides a set of annotations and assertions to write and run tests easily, and has become the industry standard for unit testing Java applications.
Why should I use JUnit for my Java projects?
JUnit offers simplicity, extensibility, integration with popular Java development tools, and a large community for support. These features make it an attractive choice for testing Java applications.
How do I get started with JUnit?
To get started, add JUnit as a dependency in your project using a build tool like Maven or Gradle. Then, create test classes that use JUnit annotations and assertions to define and run test cases.
How do I run JUnit tests?
You can run JUnit tests using your IDE's built-in test runner or from the command line using a build tool like Maven or Gradle.