Exploring the Java Collections Framework
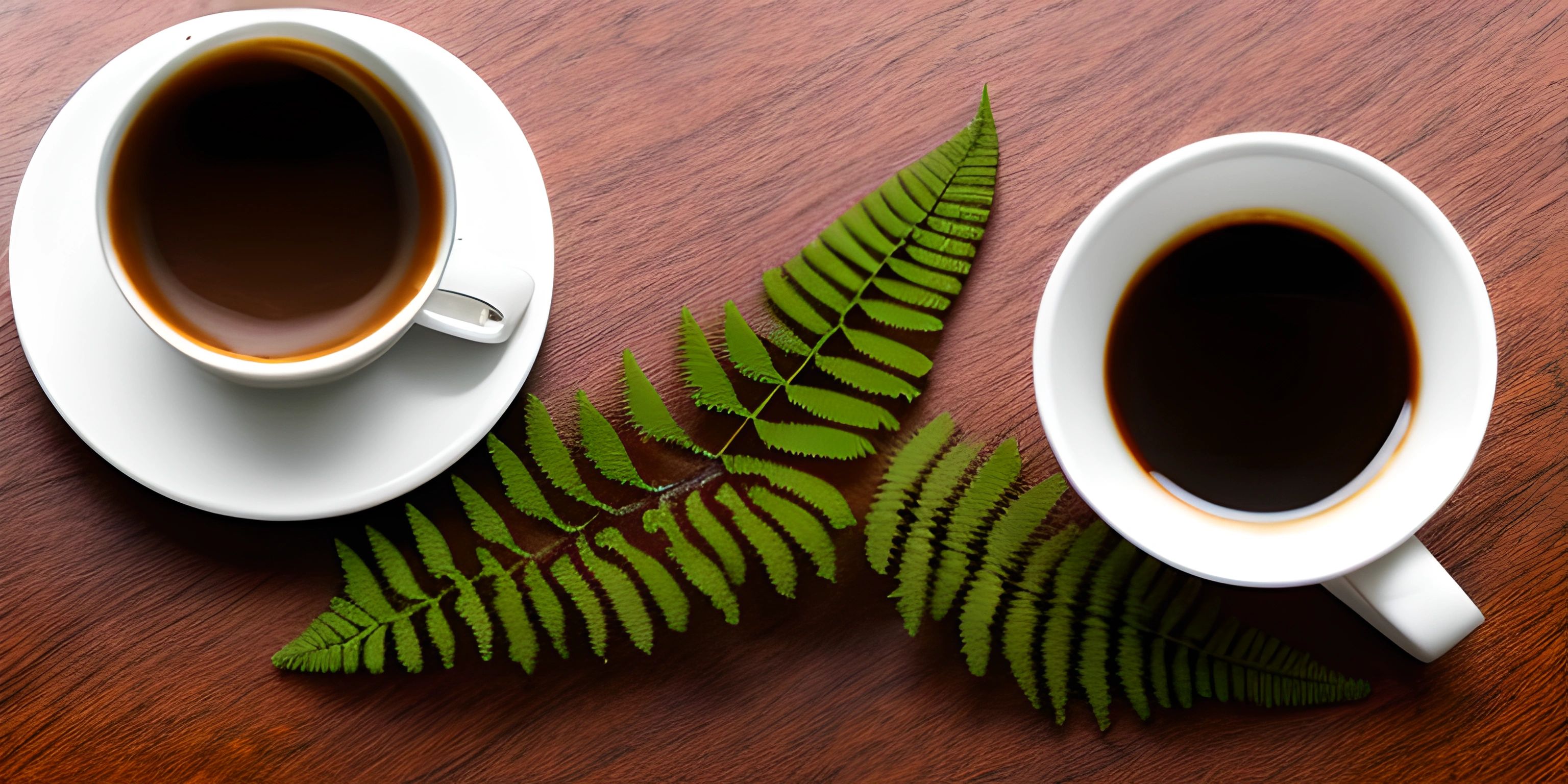
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
As a Java developer, you'll often find yourself working with data that needs to be stored, manipulated, and retrieved. The Java Collections Framework provides a powerful set of classes and interfaces to help you manage data efficiently and effectively.
Overview of the Java Collections Framework
The Java Collections Framework is a set of classes and interfaces that implement common data structures, such as lists, sets, maps, and queues. It provides a standardized way to handle collections of objects, making it easier to work with different types of data structures without having to implement them from scratch.
The framework is organized into three main parts:
- Interfaces: Define the abstract data types, such as
List
,Set
,Map
, andQueue
. - Implementations: Concrete classes that implement the interfaces, such as
ArrayList
,HashSet
,HashMap
, andLinkedList
. - Algorithms: Utility methods that perform operations on collections, such as searching, sorting, and reversing.
Let's dive into each part of the framework and see how they can be used in your Java programs.
Interfaces
The Java Collections Framework provides several interfaces to define the behavior of various data structures. Some of the most commonly used interfaces include:
List
: An ordered collection that allows duplicate elements. Elements can be accessed by their index.Set
: A collection that contains no duplicate elements. It doesn't maintain any order for its elements.Map
: A collection that maps keys to values, where each key is unique. It's also known as an associative array or dictionary.Queue
: A collection that represents a sequence of elements in a first-in, first-out (FIFO) order.
These interfaces form the foundation for the concrete classes that implement them.
Implementations
The Java Collections Framework provides several concrete classes that implement the various interfaces. Some of the most commonly used classes are:
ArrayList
: A resizable array that implements theList
interface. It's useful for situations where you need to access elements by their index quickly.HashSet
: A collection that implements theSet
interface and uses a hash table to store elements. It offers fast insertion, deletion, and search operations.HashMap
: A hash table-based implementation of theMap
interface. It maps keys to values and allows for fast key-based lookups.LinkedList
: A doubly-linked list that implements both theList
andQueue
interfaces. It's useful when you need to perform frequent additions or removals at the beginning or end of the list.
These implementations offer different performance characteristics, so it's important to choose the right one for your specific use case.
Algorithms
The Collections
utility class provides a set of algorithms that you can use to perform operations on collections, such as sorting, searching, and reversing. Some of the most commonly used methods include:
Collections.sort()
: Sorts a list of elements according to their natural order or using a provided comparator.Collections.binarySearch()
: Searches a sorted list for a specific element using the binary search algorithm.Collections.reverse()
: Reverses the order of elements in a list.
These utility methods can save you time and effort when working with collections.
Example Usage
Let's see an example of how to use the Java Collections Framework:
import java.util.ArrayList; import java.util.Collections; import java.util.List; public class JavaCollectionsExample { public static void main(String[] args) { List<String> names = new ArrayList<>(); names.add("Alice"); names.add("Bob"); names.add("Carol"); Collections.sort(names); System.out.println("Sorted names: " + names); int index = Collections.binarySearch(names, "Bob"); System.out.println("Index of Bob: " + index); Collections.reverse(names); System.out.println("Reversed names: " + names); } }
In this example, we create an ArrayList of names, sort them, find the index of a specific name, and finally reverse the order of the names.
Conclusion
The Java Collections Framework is an essential tool for managing data in your Java programs. By understanding the interfaces, implementations, and algorithms it provides, you can work with data structures more efficiently and effectively. Use the framework's classes and interfaces to choose the right data structure for your needs and leverage the utility methods to perform common operations with ease.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).