Java Variables
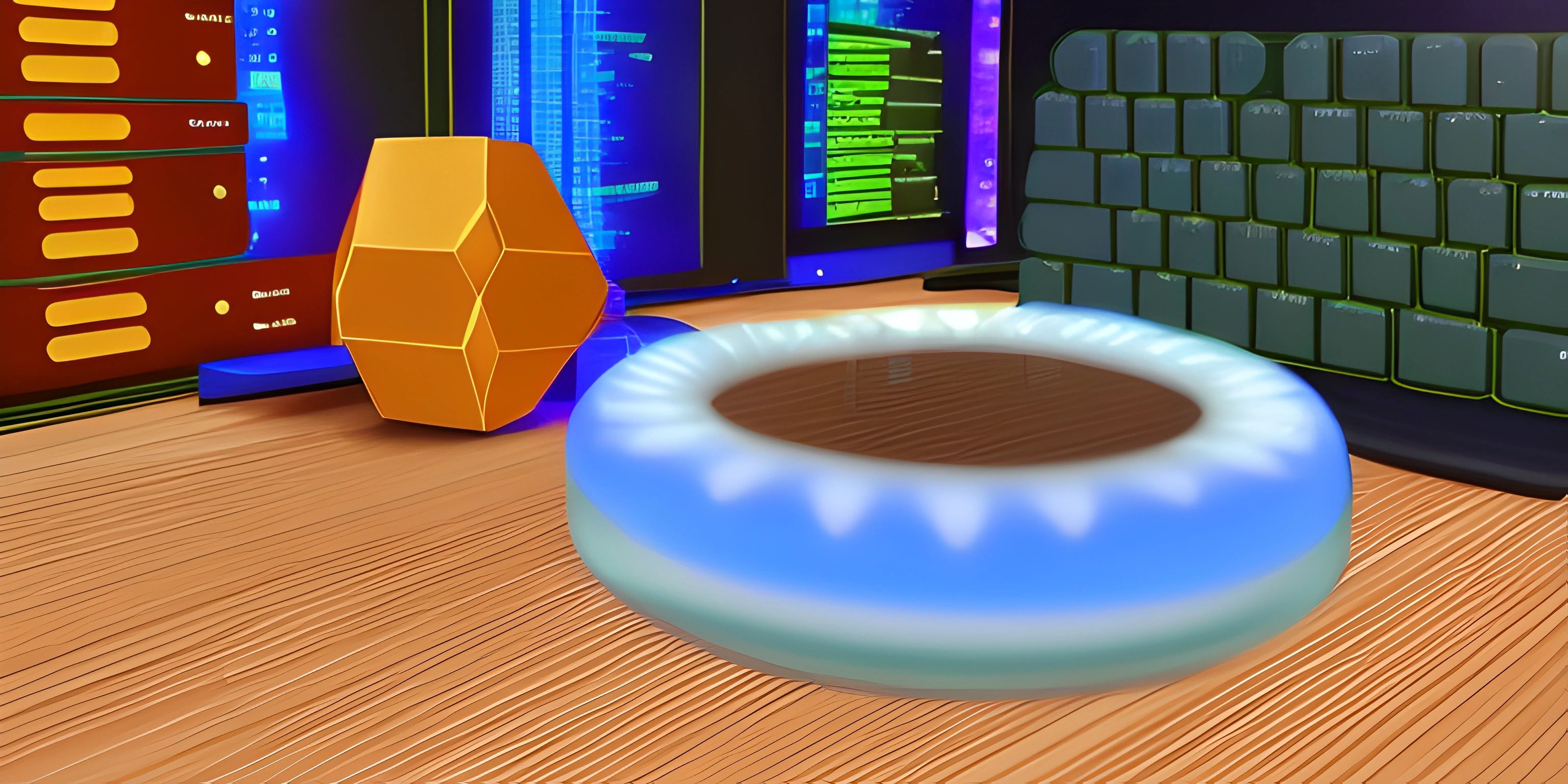
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In Java, variables are the cornerstone of coding – they're like tiny containers that keep track of information. Variables can store all kinds of data, from numbers and letters to complex objects. In this article, we'll explore how to declare and use variables in Java.
Variable Declaration
Before you can use a variable in Java, you need to declare it. Declaring a variable is like introducing it to the code – it tells Java what kind of data the variable can store and what to call it. To declare a variable, you need to specify its data type and name. Here's the general syntax:
dataType variableName;
For example, if you want to create a variable that holds an integer, you would write:
int myNumber;
Java has several built-in data types for storing different kinds of information. Some common ones are int
(integer), double
(floating-point number), and String
(a sequence of characters). You can also create custom data types using classes.
Variable Assignment
After declaring a variable, you can assign a value to it. Assignment is the process of giving a variable a value. You can use the =
operator to do this. The syntax is as follows:
variableName = value;
Let's assign a value to the myNumber
variable we declared earlier:
myNumber = 42;
Now, myNumber
contains the value 42
. You can also declare and assign a variable in a single line:
int myNumber = 42;
Using Variables
Once you've declared and assigned a variable, you can use it in your code. For example, you can use variables in mathematical expressions, print their values, or pass them as arguments to functions. Here's a simple example that adds two numbers together:
int num1 = 5; int num2 = 10; int sum = num1 + num2; System.out.println("The sum is: " + sum);
This code will output: The sum is: 15
.
Variable Scope
In Java, variables have a scope, which determines where they can be accessed within your code. The scope of a variable is determined by where it's declared. Variables declared inside a method or block of code are called local variables, and their scope is limited to that method or block. Variables declared outside any method, but inside a class, are called instance variables, and their scope is the whole class.
Final Thoughts
Variables are essential building blocks in Java programming, and understanding how to declare, assign, and use them will help you write more efficient and organized code. As you become more comfortable with Java, you'll find that variables play a crucial role in many programming tasks, from simple calculations to complex object manipulation. So practice working with variables and watch your Java skills grow!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
What are the basic types of variables in Java?
Java has two main types of variables: primitive data types and reference data types. Primitive data types include:
byte
short
int
long
float
double
char
boolean
Reference data types include objects, arrays, and strings, which store references to the actual data.
How do you declare a variable in Java?
To declare a variable in Java, you need to specify its type, followed by its name. For example:
int myNumber; String greeting;
In this example, myNumber
is an integer variable and greeting
is a String variable.
How do you assign a value to a variable in Java?
To assign a value to a variable in Java, use the assignment operator =
followed by the value you want to assign. For example:
int myNumber = 42; String greeting = "Hello, World!";
In this example, myNumber
is assigned the value 42 and greeting
is assigned the value "Hello, World!".
How do you change the value of a variable in Java?
To change the value of a variable in Java, simply use the assignment operator =
again with the new value. For example:
myNumber = 13; greeting = "Goodbye, World!";
In this example, the value of myNumber
is changed to 13 and the value of greeting
is changed to "Goodbye, World!".
Can you declare and assign a variable at the same time in Java?
Yes, you can declare and assign a variable at the same time in Java. Just include the assignment operator =
and the value you want to assign immediately after declaring the variable. For example:
int myNumber = 42; String greeting = "Hello, World!";
In this example, myNumber
is declared as an integer variable and assigned the value 42, and greeting
is declared as a String variable and assigned the value "Hello, World!".