Variables in Java
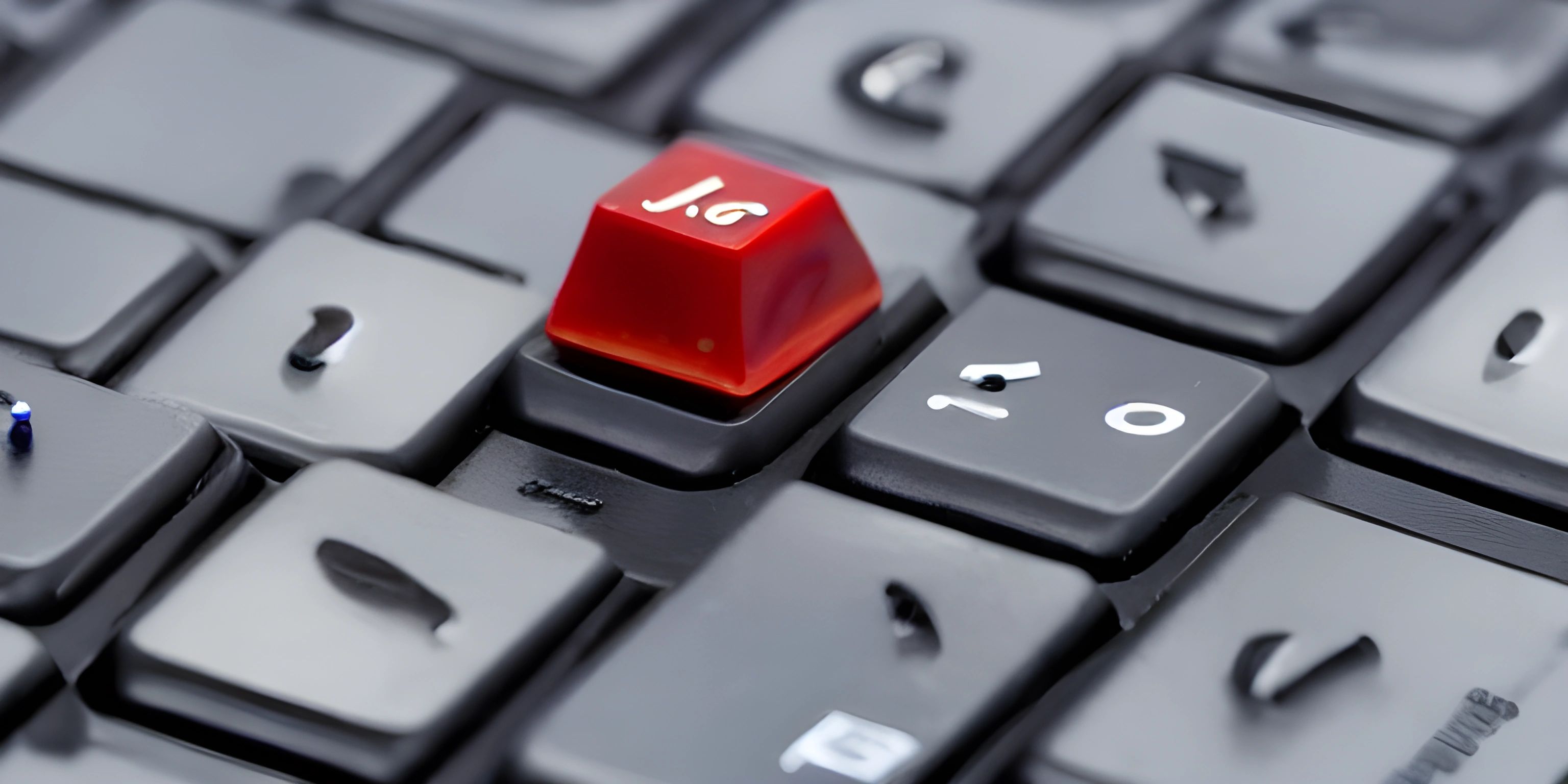
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When working with Java, one of the fundamental building blocks you'll encounter are variables. In this article, we'll delve into understanding how variables work in Java, including declaring, initializing, and using them throughout your code.
What are Variables?
Variables in Java are like containers for storing data. They have a name, a type, and a value. The type of a variable determines the kind of data it can store, such as integers, floating-point numbers, characters, or objects.
Data Types
Java has two categories of data types:
- Primitive data types: These are the basic built-in data types, such as
int
,double
,boolean
,char
, etc. - Reference data types: These are data types that store references to objects, such as
String
,Integer
, arrays, and custom classes.
Here are some common data types in Java:
int // for integer values double // for floating-point numbers boolean // for true/false values char // for single characters String // for text (a sequence of characters)
Declaring Variables
To declare a variable in Java, you must first specify its data type, followed by its name. Here's an example:
int age; double weight; boolean isActive; char firstLetter; String name;
Initializing Variables
After declaring a variable, you can assign a value to it using the assignment operator (=
). This process is called initialization:
int age = 25; double weight = 70.5; boolean isActive = true; char firstLetter = 'A'; String name = "Alice";
You can also declare and initialize a variable in the same line:
int age = 25;
Variable Scope
The scope of a variable refers to the part of the code where it can be accessed. In Java, variables can have different scopes, such as:
-
Local scope: A variable declared within a method or block of code has local scope. It can only be accessed within that method or block.
-
Instance scope: A variable declared within a class but outside any method has instance scope. It can be accessed by any method within that class.
-
Static (class) scope: A variable declared with the
static
keyword has class scope. It can be accessed by any method in the class without creating an instance of that class.
Naming Conventions
In Java, it's important to follow naming conventions for variables to keep your code readable and consistent:
- Variable names should be in camelCase (lowercase first word, uppercase subsequent words).
- They should start with a letter or underscore, followed by letters, digits, or underscores.
- Do not use Java keywords as variable names.
Here are some examples of valid variable names:
int myVar; double _interestRate; String firstName;
Now that you have a solid understanding of variables in Java, you can start using them in your programs to store and manipulate data with ease.
FAQ
What are the two categories of data types in Java?
The two categories of data types in Java are primitive data types and reference data types. Primitive data types are basic built-in data types like int, double, boolean, and char. Reference data types store references to objects, such as String, Integer, arrays, and custom classes.
How do you declare and initialize a variable in Java?
To declare a variable in Java, you specify its data type followed by its name, for example, int age;
. To initialize a variable, you assign a value to it using the assignment operator (=
), for example, age = 25;
. You can also declare and initialize a variable in the same line, like this: int age = 25;
.
What is the scope of a variable in Java?
The scope of a variable in Java refers to the part of the code where it can be accessed. Variables can have different scopes, such as local scope (within a method or block of code), instance scope (within a class but outside any method), and static (class) scope (declared with the static
keyword and accessible by any method in the class without creating an instance of that class).