Introduction to JavaScript Arrow Functions
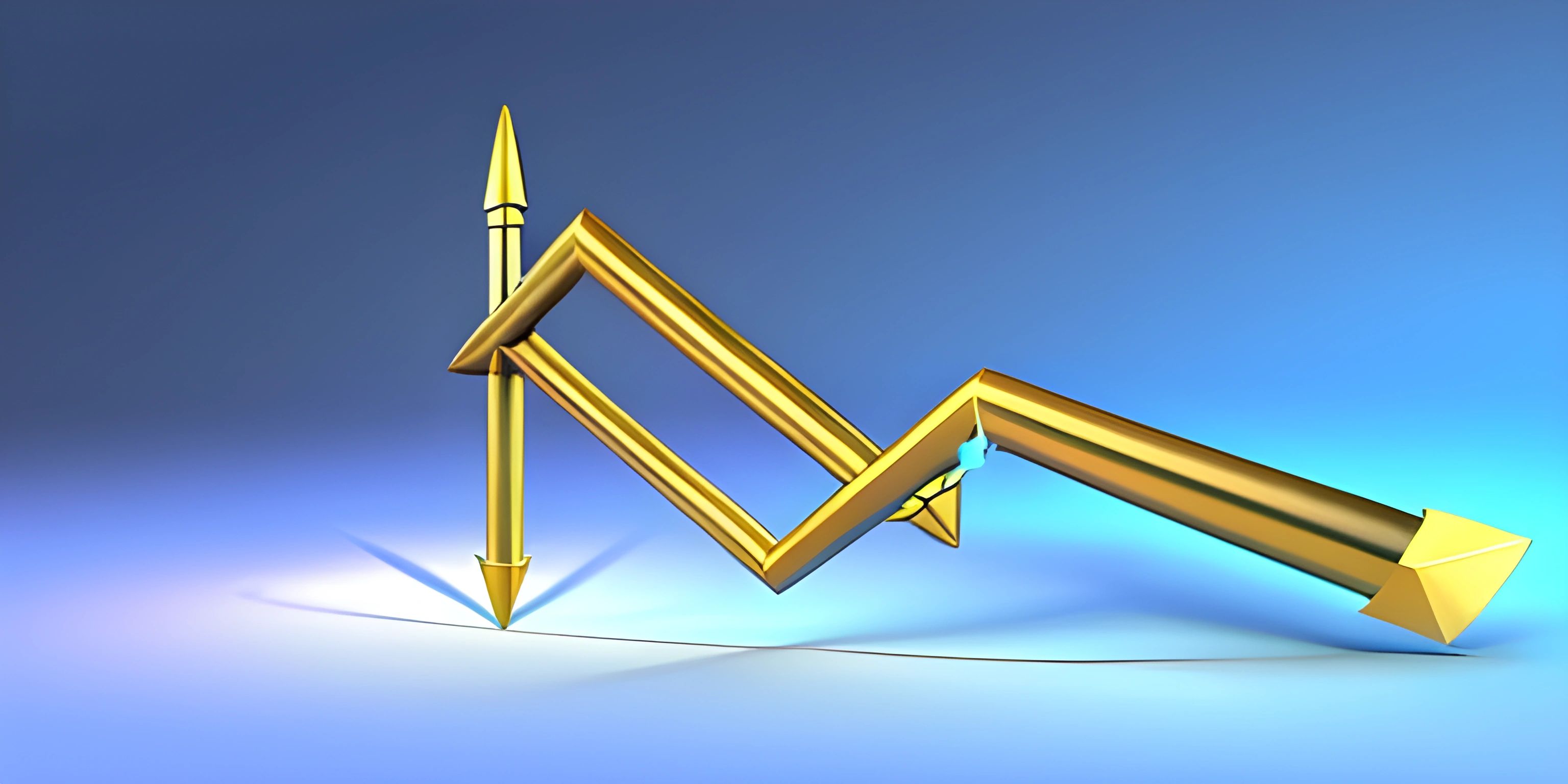
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ever wished you could write functions in JavaScript with less typing and more readability? That's where arrow functions, introduced in ES6, come into play. These nifty little additions offer a more concise syntax and some unique behavior that can make your code cleaner and more expressive. Ready to dive in? Let’s sharpen our arrows and hit the target!
What Are Arrow Functions?
Arrow functions (also known as arrow function expressions) are a shorter way to write function expressions. They use the =>
syntax, which might remind you of a bow and arrow — and they’re just as swift!
Here’s a quick comparison to show the difference:
Traditional Function Expression
var add = function(a, b) { return a + b; };
Arrow Function
const add = (a, b) => { return a + b; };
Notice the brevity? Let's explore further.
Arrow Function Syntax
The basic syntax of an arrow function looks like this:
(param1, param2, ..., paramN) => { statements }
If the function has only one parameter, you can skip the parentheses:
param => { // statements }
For single-line statements, you can even omit the curly braces and the return
keyword:
const multiply = (a, b) => a * b;
The above function multiplies two numbers and returns the result. Neat, right?
Contextual "This" Binding
One of the standout features of arrow functions is their handling of the this
keyword. Unlike traditional functions, arrow functions do not have their own this
context; they inherit this
from the parent scope.
Here's an example to illustrate:
Traditional Function and This
function Person() { this.age = 0; setInterval(function growUp() { this.age++; }, 1000); } const p = new Person();
In the above code, this.age
inside growUp
does not refer to the Person
object, but to the global object (or undefined
in strict mode). We can fix this with arrow functions:
Arrow Function and This
function Person() { this.age = 0; setInterval(() => { this.age++; }, 1000); } const p = new Person();
Now, this.age
correctly refers to the Person
object. The arrow function ensures that this
is lexically bound.
Use Cases and Examples
Shorter Function Expressions
Arrow functions are perfect for situations where you need short, inline function expressions. For example, in array methods like map
, filter
, and reduce
:
const numbers = [1, 2, 3, 4, 5]; const doubled = numbers.map(n => n * 2); console.log(doubled); // [2, 4, 6, 8, 10]
No arguments
Object
Arrow functions do not have their own arguments
object. If you need access to the arguments
object, use a rest parameter:
const sum = (...args) => args.reduce((acc, val) => acc + val, 0); console.log(sum(1, 2, 3, 4)); // 10
Methods in Objects
Arrow functions are not suited for defining methods in objects, as they do not have their own this
. Instead, use traditional function expressions for methods:
const obj = { value: 42, getValue: function() { return this.value; } }; console.log(obj.getValue()); // 42
Callback Functions
Arrow functions are particularly useful for callbacks, as they reduce boilerplate code and make the intent clearer:
setTimeout(() => { console.log("Time's up!"); }, 1000);
Common Pitfalls
While arrow functions are powerful, there are some caveats to be aware of:
- Constructor Functions: Arrow functions cannot be used as constructors and will throw an error if used with
new
. - Method Definitions: Avoid using arrow functions for defining methods in objects or classes, as they do not have their own
this
.
Conclusion
Arrow functions are a fantastic addition to JavaScript, offering a concise and readable way to write function expressions, alongside some useful behavior with the this
keyword. They’re not a one-size-fits-all solution, but they’re invaluable for making code cleaner and more modern. So next time you find yourself writing a simple function, consider reaching for an arrow function!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Taking User Input (psst, it's free!).
FAQ
What are arrow functions in JavaScript?
Arrow functions are a more concise way to write function expressions in JavaScript, introduced in ES6. They use the =>
syntax and offer benefits like shorter syntax and lexical this
binding.
Can arrow functions be used as constructors?
No, arrow functions cannot be used as constructors and will throw an error if you try to use them with the new
keyword.
How do arrow functions handle the `this` keyword?
Arrow functions do not have their own this
context. Instead, they inherit this
from the surrounding lexical context, making them useful in scenarios where you want to preserve the this
value.
Are arrow functions suitable for defining methods in objects?
No, arrow functions are not suitable for defining methods in objects because they do not have their own this
. Use traditional function expressions for object methods instead.
Do arrow functions have an `arguments` object?
No, arrow functions do not have their own arguments
object. If you need access to the arguments passed to an arrow function, use the rest parameter syntax (...args
).