Creating and Using Function Callbacks in JavaScript
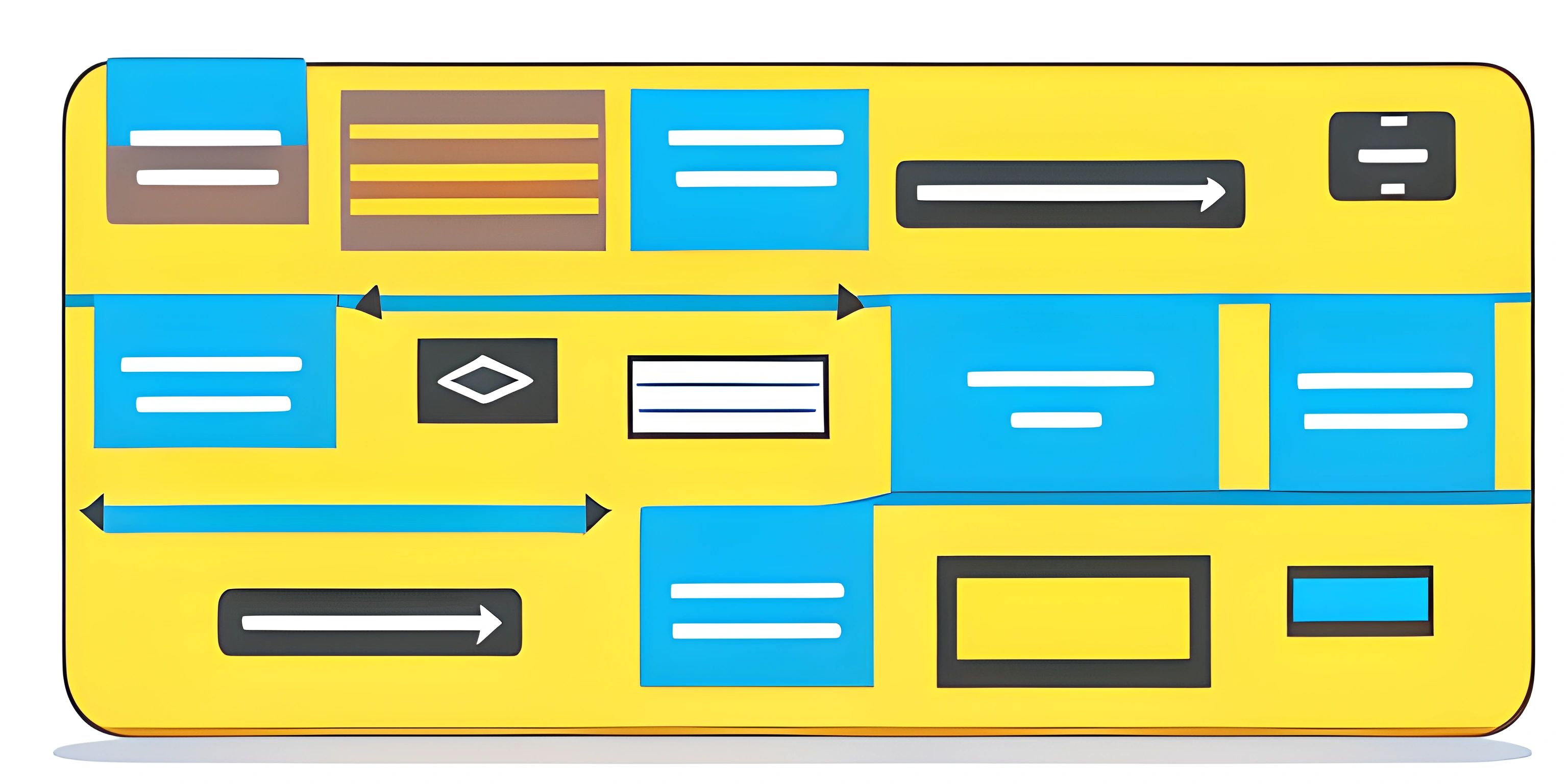
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Function callbacks are an essential concept in JavaScript that allows you to pass functions as arguments to other functions. Callbacks are particularly useful for handling asynchronous operations and organizing your code more efficiently.
What is a Callback Function?
A callback function is a function that is passed as an argument to another function, and it is executed after the completion of the other function. Callbacks are a way to ensure that certain code doesn't execute until other code has already finished execution.
Creating a Callback Function
Let's create a simple example to understand how callback functions work. We'll create a function called processData
that takes two arguments: a string and a callback function. The processData
function will convert the string to uppercase and then call the callback function with the modified string as an argument.
function processData(input, callback) { const output = input.toUpperCase(); callback(output); }
Now, let's create a callback function called displayData
that takes a string as an argument and logs it to the console.
function displayData(data) { console.log("Data: " + data); }
To use the processData
function with the displayData
callback, we simply pass the displayData
function as an argument when calling processData
.
processData("hello world", displayData); // Output: Data: HELLO WORLD
Callbacks in Asynchronous Operations
Callbacks are often used in asynchronous operations, such as reading a file, making API calls, or handling user input. Let's create an example using the setTimeout
function, which is an asynchronous function that executes a callback after a specified delay.
function delayedGreeting(name, callback) { setTimeout(() => { const greeting = "Hello, " + name; callback(greeting); }, 3000); } function displayGreeting(greeting) { console.log(greeting); } delayedGreeting("John", displayGreeting); // Output (after 3 seconds): Hello, John
In this example, the delayedGreeting
function takes a name and a callback function as arguments. It then uses setTimeout
to wait for 3 seconds before executing the callback with a greeting message.
Conclusion
Callback functions in JavaScript are a powerful way to manage asynchronous operations and improve code organization. By passing functions as arguments, you can control the execution order of your code and handle events more efficiently. Practice using callbacks in your JavaScript projects to get a better understanding of this essential concept.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Palindromes (psst, it's free!).
FAQ
What is a callback function in JavaScript?
A callback function is a function that gets passed as an argument to another function and is executed after the main function completes its operation. Callbacks are particularly useful in asynchronous operations, allowing your code to continue processing while waiting for a specific task to complete.
How do I create a callback function in JavaScript?
Creating a callback function in JavaScript is simple. You just define a function and pass it as an argument to another function. Here's an example:
function greeting(name, callback) { console.log("Hello, " + name); callback(); } function sayGoodbye() { console.log("Goodbye!"); } greeting("John", sayGoodbye);
In this example, sayGoodbye
is passed as a callback function to greeting
.
Can I use anonymous functions as callbacks in JavaScript?
Absolutely! You can use anonymous functions (also known as "lambda functions" or "arrow functions") as callbacks in JavaScript. Here's an example:
function greeting(name, callback) { console.log("Hello, " + name); callback(); } greeting("Jane", function() { console.log("Goodbye!"); });
In this case, the anonymous function function() { console.log("Goodbye!"); }
is passed as a callback to the greeting
function.
How do callback functions help with asynchronous operations?
Callback functions are a useful way to handle asynchronous operations because they allow your code to continue processing other tasks while waiting for a specific operation to complete. Once the operation is done, the callback function is executed, ensuring that any dependent code runs at the correct time. For example, if you're fetching data from an API, you can use a callback function to process and display the data once it's received. This prevents your code from blocking, or waiting idly, while the data is being fetched.
How can I handle errors in callback functions?
One common approach to handling errors in callback functions is to include an additional parameter in the callback function to represent an error. If an error occurs, this parameter will be populated with error information. Here's an example:
function fetchData(callback) { // Simulate an API call setTimeout(function() { const error = null; // or an Error object if something goes wrong const data = "Sample data"; callback(error, data); }, 1000); } fetchData(function(err, data) { if (err) { console.error("An error occurred:", err); } else { console.log("Data received:", data); } });
In this example, the fetchData
function simulates an API call using setTimeout
. It then calls the callback function with an error
and data
parameters. The callback function checks for an error and handles it accordingly.