Mastering Asynchronous Programming with Promises, Async, and Await
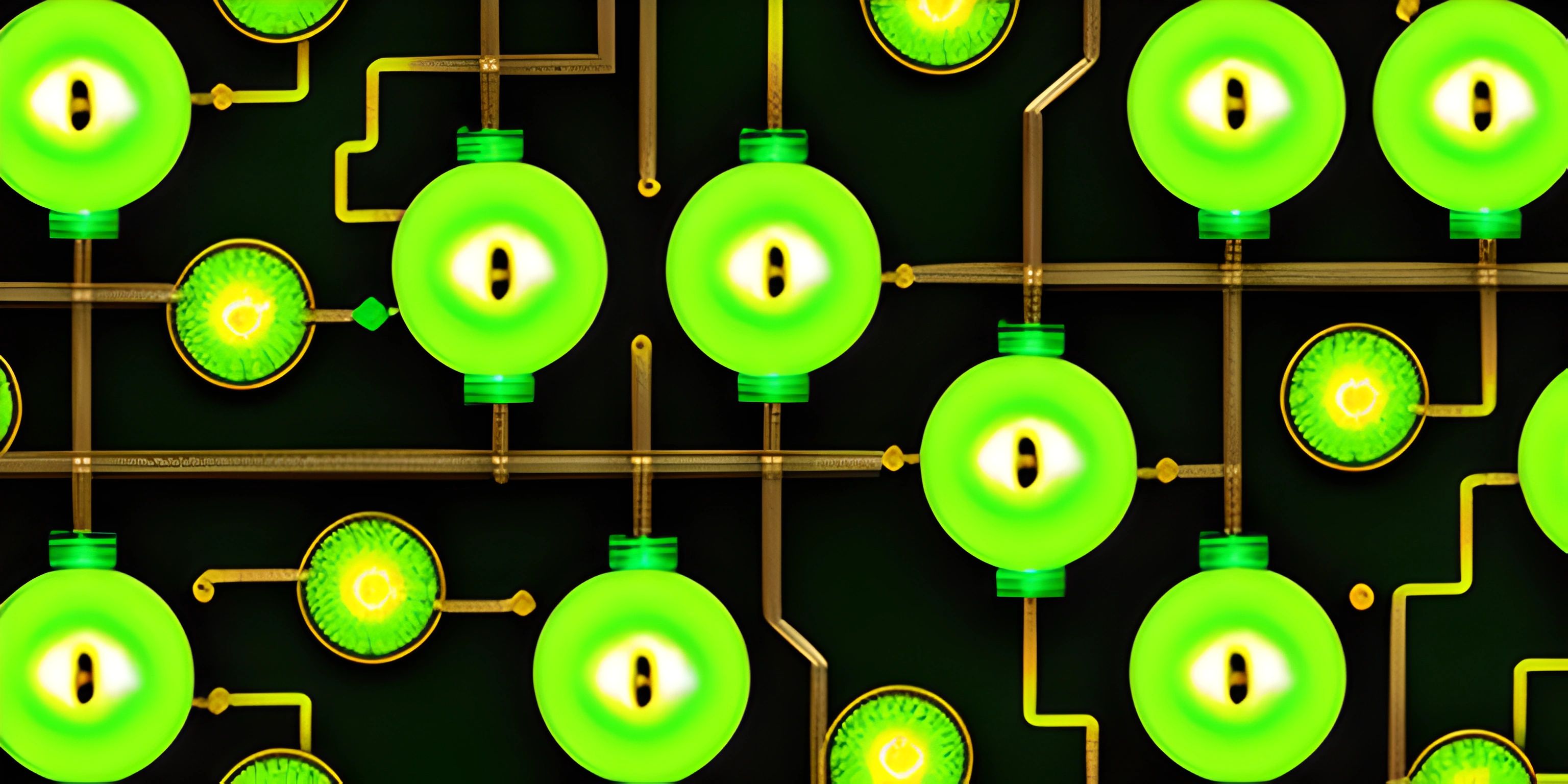
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Asynchronous programming is a fundamental concept in JavaScript, allowing you to perform tasks without blocking the main thread. This article will cover how to use Promises, async, and await to handle asynchronous programming effectively.
Promises
Promises in JavaScript are objects that represent the eventual completion (or failure) of an asynchronous operation and its resulting value. A Promise is said to be "settled" if it has either been fulfilled (completed successfully) or rejected (completed with an error).
Here's a simple example of a Promise:
const promise = new Promise((resolve, reject) => { setTimeout(() => { resolve("Promise resolved!"); }, 2000); }); promise.then(result => { console.log(result); // "Promise resolved!" });
In this example, we create a Promise that resolves after 2 seconds. The then
method is used to attach a callback function that will be executed when the Promise is resolved.
Async and Await
Async and await are keywords in JavaScript that work together with Promises to make asynchronous programming more readable and easier to write. The async
keyword is used to declare an asynchronous function, which returns a Promise.
The await
keyword can only be used inside an async function and makes JavaScript wait until the Promise settles (either fulfilled or rejected) before continuing the execution of the async function.
Here's an example of using async and await:
async function fetchData() { const response = await fetch("https://api.example.com/data"); const data = await response.json(); console.log(data); } fetchData();
In this example, we create an async function called fetchData
. Inside the function, we use the await
keyword to wait for the fetch
and response.json()
Promises to settle before moving on to the next line of code.
Error Handling with Async and Await
Error handling with async and await is very similar to handling errors with Promises. You can use a try-catch block to catch any errors that may occur during the execution of the async function.
Here's an example of error handling with async and await:
async function fetchDataWithErrorHandling() { try { const response = await fetch("https://api.example.com/data"); const data = await response.json(); console.log(data); } catch (error) { console.error("Error fetching data:", error); } } fetchDataWithErrorHandling();
In this example, we wrap the fetch and response.json() calls inside a try block. If an error occurs, the catch block will be executed, and the error message will be logged.
In conclusion, Promises, async, and await are powerful tools for handling asynchronous programming in JavaScript. They make your code more readable and easier to write, allowing you to create more efficient and maintainable applications.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Async Rust (psst, it's free!).
FAQ
What is asynchronous programming and why is it important in JavaScript?
Asynchronous programming is a programming paradigm that allows multiple tasks to be performed concurrently, without waiting for one task to complete before starting another. In JavaScript, it's essential because it helps prevent blocking the main thread, which could lead to performance issues and unresponsive user interfaces. Asynchronous operations are commonly used for tasks such as fetching data from a server or reading from a file.
How do Promises help with asynchronous programming in JavaScript?
Promises are a way to handle asynchronous operations in JavaScript by providing a clean, simple, and powerful API. A Promise represents the eventual result of an asynchronous operation, and it can be in one of three states: pending, fulfilled, or rejected. Promises allow you to attach callbacks that will be called when the operation is completed or when an error occurs. This helps you avoid the "callback hell" that can arise from deeply nested callbacks in asynchronous code.
What is the purpose of the `async` keyword in JavaScript?
The async
keyword is used to declare a function as asynchronous. This means that the function will return a Promise, allowing you to use the await
keyword inside the function to pause the execution of the code until a Promise is resolved. This makes your asynchronous code look and behave more like synchronous code, making it easier to read, debug, and maintain.
How does the `await` keyword work in asynchronous JavaScript code?
The await
keyword is used inside an async
function to pause the execution of the code until a Promise is resolved or rejected. When a Promise is resolved, the await
keyword returns the fulfilled value, allowing you to continue with the rest of the code. If the Promise is rejected, an error is thrown and can be caught using a try
-catch
block. This makes asynchronous code easier to understand and manage.
Can I use `async` and `await` with regular callbacks or only with Promises?
The async
and await
keywords are specifically designed to work with Promises. If you have a callback-based function, you can easily convert it to a Promise-based function using the Promise
constructor. This will allow you to take advantage of async
and await
to simplify your asynchronous code.