Mastering Async, Await, and Promises in Node.js
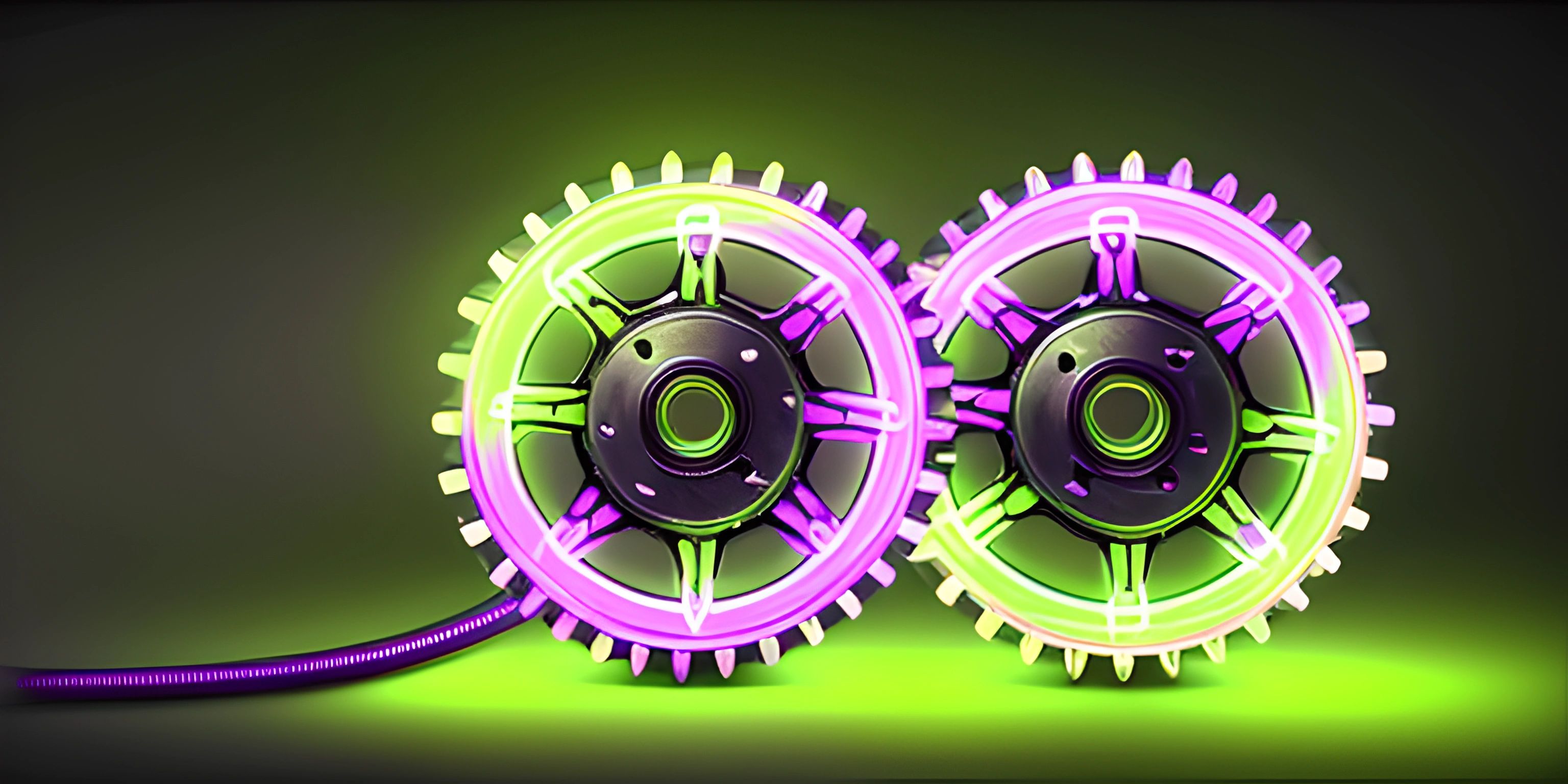
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Asynchronous programming is a fundamental concept in JavaScript and Node.js. It allows you to perform non-blocking operations, which means that your code can continue running while waiting for other tasks to complete. In this article, we'll explore async, await, and promises in Node.js and how to use them effectively.
Promises
A Promise is a JavaScript object that represents the eventual completion (or failure) of an asynchronous operation and its resulting value. Promises have three states:
- Pending: The initial state; neither fulfilled nor rejected.
- Fulfilled: The operation completed successfully, and the promise has a resulting value.
- Rejected: The operation failed, and the promise has a reason for the failure.
Promises can be created using the Promise
constructor, which takes a single argument: a function called the "executor". The executor function takes two arguments: a resolve
function and a reject
function. The resolve
function is used to fulfill the promise, while the reject
function is used to reject it.
Here's an example:
const myPromise = new Promise((resolve, reject) => { setTimeout(() => { resolve("Promise fulfilled!"); }, 1000); });
You can use the .then()
method to attach callbacks that will be called when the promise is fulfilled, and the .catch()
method to attach callbacks that will be called when the promise is rejected.
myPromise .then((value) => { console.log(value); // "Promise fulfilled!" }) .catch((error) => { console.log(error); });
Async/Await
Async/await is a more modern and cleaner way to work with promises. It makes your asynchronous code look and behave more like synchronous code.
To use async/await, you need to declare a function as async
:
async function myAsyncFunction() { // ... }
Inside an async
function, you can use the await
keyword before a promise to wait for its resolution. The function will be paused until the promise is either fulfilled or rejected.
Here's an example using the previous myPromise
:
async function myAsyncFunction() { try { const value = await myPromise; console.log(value); // "Promise fulfilled!" } catch (error) { console.log(error); } } myAsyncFunction();
Note that we've used a try
/catch
block to handle promise rejections. This is a more familiar way of handling errors compared to using .catch()
.
Conclusion
Async/await and promises are powerful tools for handling asynchronous operations in Node.js. They allow you to write cleaner, more readable, and maintainable code. Practice using them in your projects to make your asynchronous code more efficient and easier to work with.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Upgrading our Calculator (psst, it's free!).
FAQ
What are async, await, and promises in Node.js?
Async, await, and promises are features in Node.js that help handle asynchronous operations more effectively. They allow you to write cleaner, more maintainable, and more readable code when dealing with tasks that might take some time to complete, such as reading files, making API calls, or querying a database.
How do promises work in Node.js?
Promises in Node.js represent the eventual completion (or failure) of an asynchronous operation and its resulting value. A Promise
is an object that has a then
method for attaching callbacks to be called when the promise is resolved, and a catch
method for attaching callbacks to be called when the promise is rejected. Promises help you avoid callback hell by providing a more concise and consistent way to manage asynchronous code.
function myAsyncFunction() { return new Promise((resolve, reject) => { // Asynchronous operation here if (/* success */) { resolve("Success!"); } else { reject("Error!"); } }); } myAsyncFunction() .then(result => console.log(result)) .catch(error => console.error(error));
How do async and await simplify working with promises in Node.js?
The async
and await
keywords in Node.js make working with promises even easier by allowing you to write asynchronous code that looks and behaves like synchronous code.
To use await
, you need to be inside a function that's marked with the async
keyword. You can then use await
before any expression that returns a promise, and the function will pause until the promise is resolved or rejected.
async function myAsyncFunction() { try { const result = await fetchSomeData(); console.log(result); } catch (error) { console.error(error); } }
Can I use async/await and promises with error handling?
Yes, you can use error handling with async/await and promises. When using promises, you can use the catch
method to handle rejections. With async/await, you can use a try
/catch
block to handle errors that might occur while awaiting a promise.
async function myAsyncFunction() { try { const result = await somePromiseFunction(); console.log(result); } catch (error) { console.error(error); } }
How do I handle multiple asynchronous operations using async/await and promises?
You can handle multiple asynchronous operations using Promise.all()
or Promise.race()
when working with promises. With async/await, you can use await
inside a loop, or combine it with Promise.all()
or Promise.race()
to handle multiple async operations.
// Using Promise.all() with async/await async function myAsyncFunction() { try { const [result1, result2] = await Promise.all([fetchData1(), fetchData2()]); console.log(result1, result2); } catch (error) { console.error(error); } } // Using a loop with async/await async function processItems(items) { for (const item of items) { const result = await processItem(item); console.log(result); } }