Proper Error Handling Techniques in JavaScript
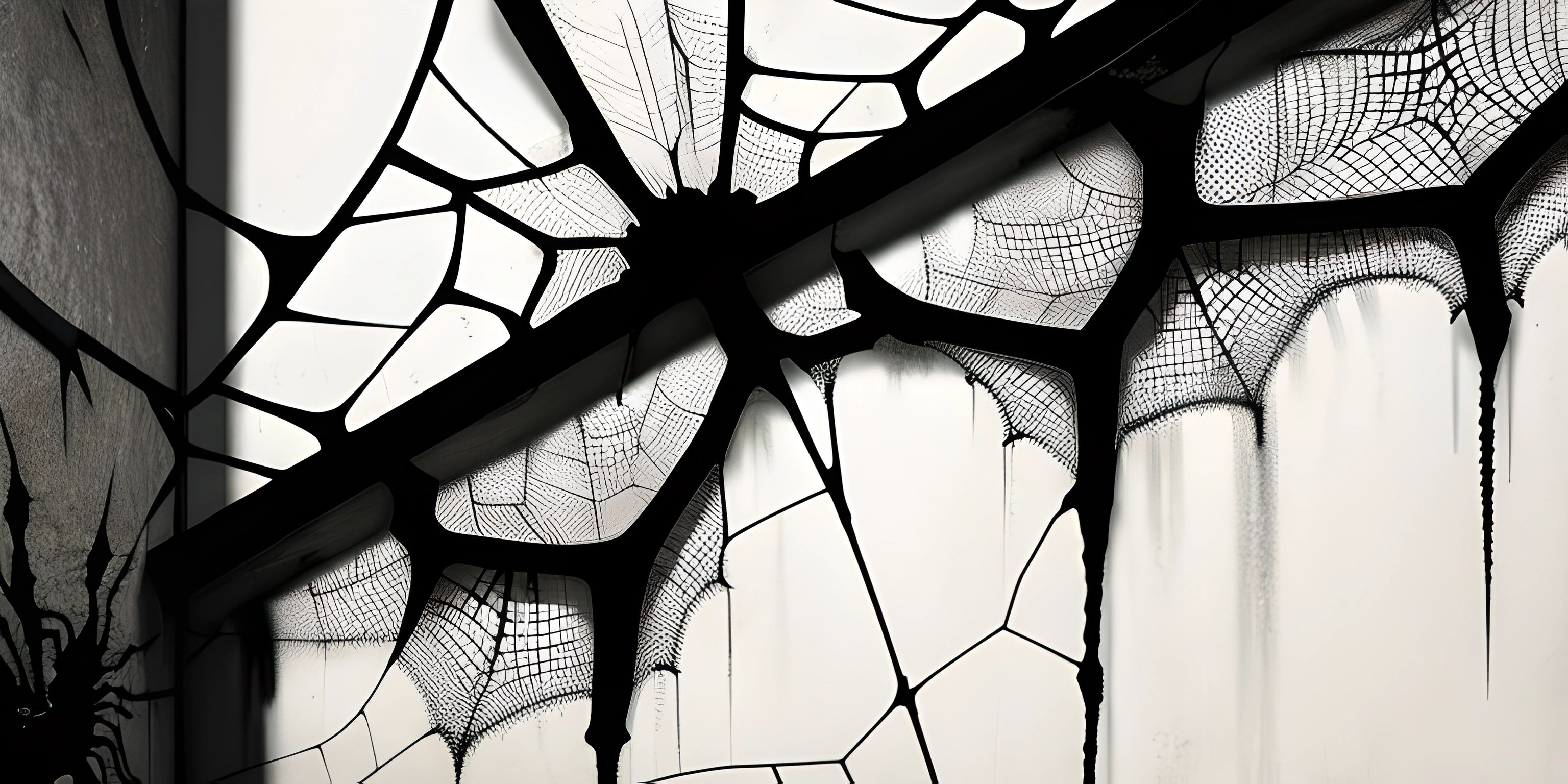
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In JavaScript, errors can occur during runtime, causing the program to stop executing. Proper error handling techniques can help you write more robust and maintainable code by anticipating and dealing with these errors gracefully. In this article, we will explore some common error handling techniques in JavaScript, such as:
- Using try-catch blocks
- Handling errors in promises
- Error handling with async-await
Using try-catch blocks
One of the most basic error handling techniques in JavaScript is the use of try-catch
blocks. The try
block contains the code that may throw an error, while the catch
block handles the error if it occurs.
try { // Code that may throw an error const result = riskyOperation(); console.log("Result:", result); } catch (error) { console.error("An error occurred:", error.message); }
Handling errors in promises
Promises are a common way to handle asynchronous operations in JavaScript. To handle errors when using promises, you can use the catch
method, which is called when the promise is rejected.
performAsyncOperation() .then(result => { console.log("Result:", result); }) .catch(error => { console.error("An error occurred:", error.message); });
You can also handle both fulfilled and rejected promises using the then
method with two arguments: the first one is a callback for the fulfilled promise, and the second one is a callback for the rejected promise.
performAsyncOperation() .then( result => { console.log("Result:", result); }, error => { console.error("An error occurred:", error.message); } );
Error handling with async-await
async-await
is a modern way to handle asynchronous code in JavaScript. To handle errors when using async-await
, you can use a try-catch
block inside an async
function.
async function performAsyncTask() { try { const result = await performAsyncOperation(); console.log("Result:", result); } catch (error) { console.error("An error occurred:", error.message); } } performAsyncTask();
By using these error handling techniques, you can write more robust and maintainable JavaScript code that gracefully handles errors during runtime.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Chat App Backend (psst, it's free!).
FAQ
What are the most common error handling techniques in JavaScript?
The most common error handling techniques in JavaScript include:
- Try-catch-finally block: This allows you to handle exceptions by wrapping your code inside a
try
block, catching any errors with acatch
block, and executing cleanup code in afinally
block. - Callbacks: You can pass callback functions as arguments to other functions, and invoke them when an error or exceptional situation occurs.
- Promises: Promises are a more modern approach to handling asynchronous operations and errors, with the
then
,catch
, andfinally
methods. - Async/await: This is a more recent addition to JavaScript, which allows you to write asynchronous code in a more synchronous manner, making it easier to handle errors with try-catch blocks.
How do I use a try-catch-finally block in JavaScript?
You can use a try-catch-finally block like this:
try { // Your code that might throw an error } catch (error) { // Handle the error } finally { // Cleanup code, executed regardless of an error being thrown or not }
Place the code that might throw an error inside the try
block. If an error is thrown, the catch
block will be executed with the error object. The finally
block is optional and will always be executed after the try and catch blocks, whether an error was thrown or not.
How can I handle errors with callbacks?
When using callbacks to handle errors, you generally follow the "error-first" pattern, where the first argument of the callback function is reserved for an error object. Here's an example:
function doSomething(callback) { // Perform an operation that might result in an error if (error) { callback(error); } else { callback(null, result); } } doSomething((error, result) => { if (error) { // Handle the error } else { // Process the result } });
What are the benefits of using Promises and async/await for error handling?
Promises and async/await provide a more structured and readable way of handling errors, especially in asynchronous code. Promises allow you to chain then
and catch
methods to handle success and error cases in a more linear fashion. Async/await, on the other hand, makes your asynchronous code look more like synchronous code, allowing you to use try-catch blocks for error handling. This can make it easier to understand and maintain your code.