Using LocalStorage and SessionStorage in Browser JavaScript
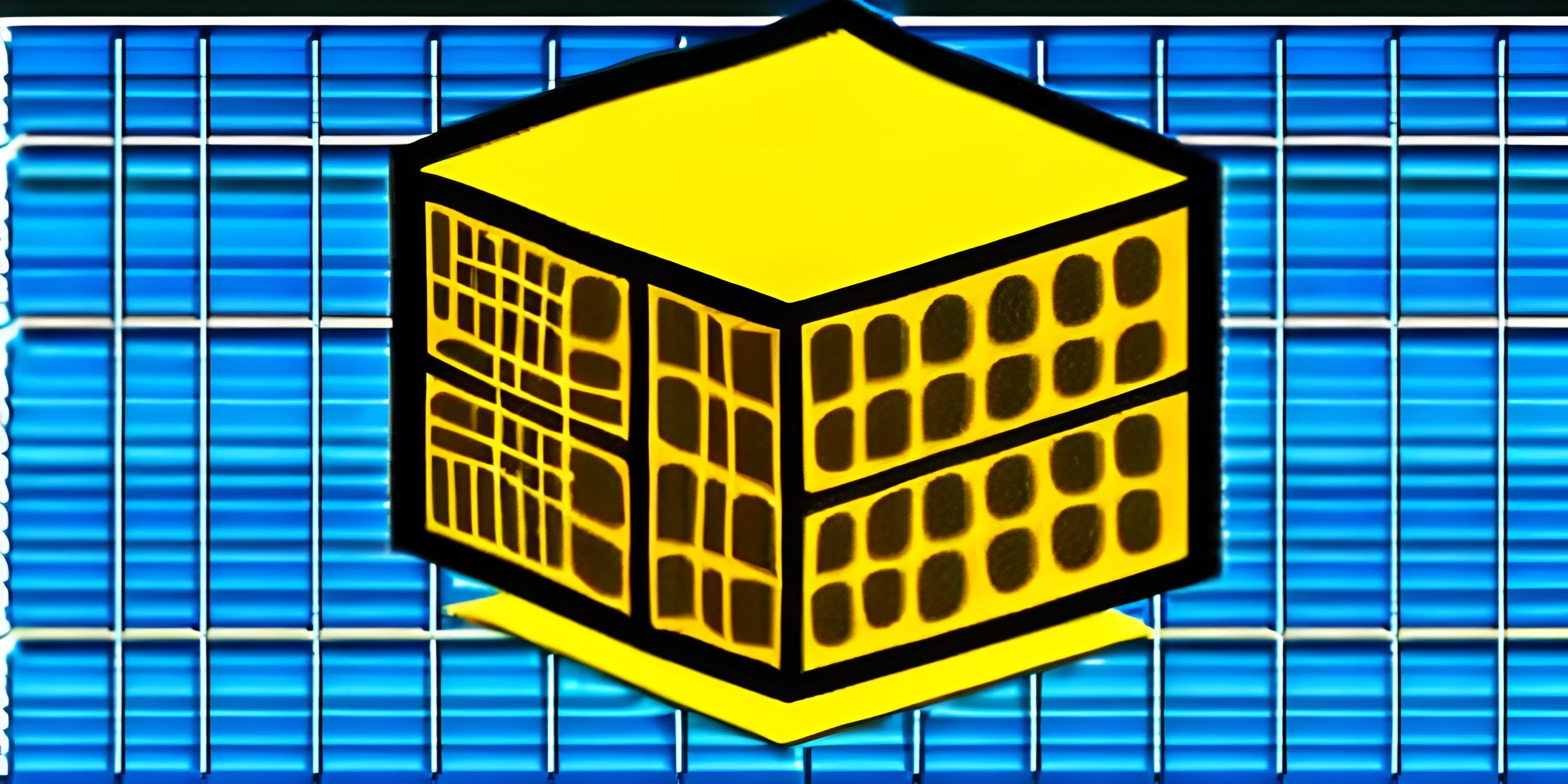
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In modern web applications, it is often necessary to store data on the client side to improve the user experience. Two popular options for client-side storage are LocalStorage
and SessionStorage
. Both of these storage mechanisms are part of the Web Storage API and are available in most modern browsers.
LocalStorage
and SessionStorage
differ in their storage duration:
LocalStorage
: The data is stored with no expiration time, and it remains in the browser even after the user closes the browser or navigates away from the site.SessionStorage
: The data is stored only for the duration of the page session, and it is cleared when the user closes the browser tab or window.
Basic Usage of LocalStorage and SessionStorage
Storing Data
To store data in LocalStorage
, use the setItem()
method:
localStorage.setItem("key", "value");
To store data in SessionStorage
, use the setItem()
method:
sessionStorage.setItem("key", "value");
Retrieving Data
To retrieve data from LocalStorage
, use the getItem()
method:
let value = localStorage.getItem("key");
To retrieve data from SessionStorage
, use the getItem()
method:
let value = sessionStorage.getItem("key");
Removing Data
To remove data from LocalStorage
, use the removeItem()
method:
localStorage.removeItem("key");
To remove data from SessionStorage
, use the removeItem()
method:
sessionStorage.removeItem("key");
Clearing All Data
To clear all data from LocalStorage
, use the clear()
method:
localStorage.clear();
To clear all data from SessionStorage
, use the clear()
method:
sessionStorage.clear();
Example: Storing and Retrieving User Preferences
Suppose you want to store a user's preferred theme (light or dark) in LocalStorage
. You can store the preference like this:
localStorage.setItem("theme", "dark");
Later, you can retrieve the user's preferred theme and apply it to your application:
let theme = localStorage.getItem("theme"); if (theme) { // Apply the theme to your application }
Keep in mind that both LocalStorage
and SessionStorage
store data as strings. If you need to store more complex data types, you can use JSON.stringify()
and JSON.parse()
to convert between strings and JavaScript objects.
Now you should have a basic understanding of how to use LocalStorage
and SessionStorage
for client-side storage in browser JavaScript. These storage mechanisms can be very useful for improving the user experience of your web applications.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What are the differences between LocalStorage and SessionStorage?
Both LocalStorage and SessionStorage are used for storing data on the client side in browser JavaScript. However, there are some key differences between the two:
- Persistence: Data saved in LocalStorage is persistent and will remain even after the browser is closed, whereas data in SessionStorage is temporary and will be deleted once the browser session ends (e.g., when the browser is closed or the page is refreshed).
- Scope: LocalStorage is shared across all windows or tabs with the same origin (domain), while SessionStorage is unique per window or tab.
How can I store an item in LocalStorage or SessionStorage?
To store an item in LocalStorage or SessionStorage, you can use the setItem
method. Here's an example for both:
// Store data in LocalStorage
localStorage.setItem("key", "value");
// Store data in SessionStorage
sessionStorage.setItem("key", "value");
Replace "key" with the name of the key you want to use, and "value" with the data you want to store.
How can I retrieve data from LocalStorage or SessionStorage?
To retrieve data from LocalStorage or SessionStorage, use the getItem
method. Here's how to do it for both:
// Retrieve data from LocalStorage
let data = localStorage.getItem("key");
// Retrieve data from SessionStorage
let data = sessionStorage.getItem("key");
Replace "key" with the name of the key you want to retrieve. If the key doesn't exist, getItem
will return null
.
How do I remove an item from LocalStorage or SessionStorage?
To remove an item from LocalStorage or SessionStorage, use the removeItem
method. Here's an example for both:
// Remove an item from LocalStorage
localStorage.removeItem("key");
// Remove an item from SessionStorage
sessionStorage.removeItem("key");
Replace "key" with the name of the key you want to remove.
How can I clear all data from LocalStorage or SessionStorage?
To clear all data from LocalStorage or SessionStorage, use the clear
method. Here's how to do it for both:
// Clear all data from LocalStorage
localStorage.clear();
// Clear all data from SessionStorage
sessionStorage.clear();
This will remove all key-value pairs stored in LocalStorage or SessionStorage, respectively.