Using Browser JavaScript to Create Elements on the Fly
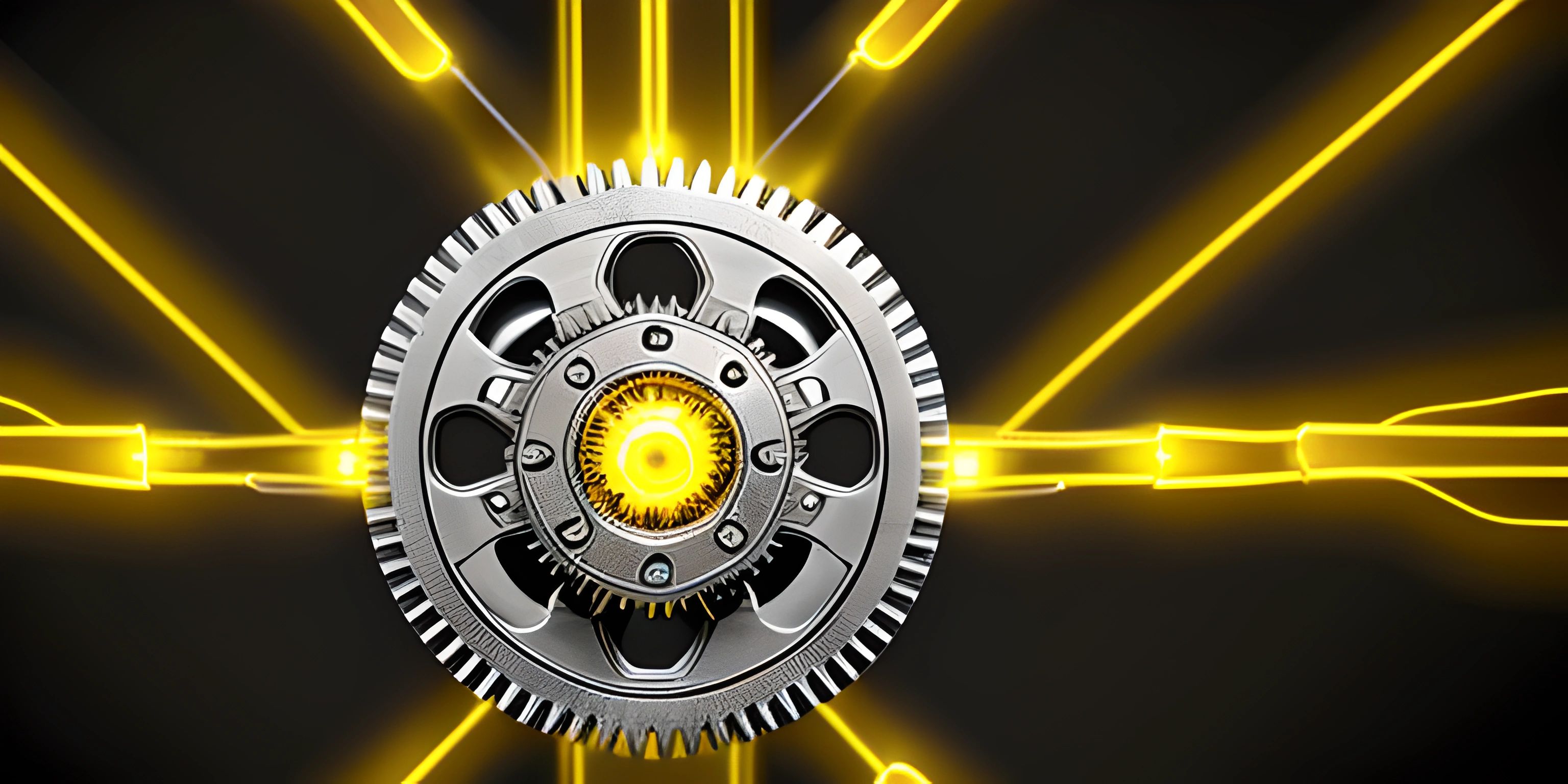
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In this article, we will learn how to create and manipulate HTML elements dynamically using JavaScript in the browser. This can be useful for adding new content, modifying existing content, or creating interactive web pages.
Creating an Element
To create an element, you can use the document.createElement()
method. This method accepts a single argument, the tag name of the element you want to create. Here's an example of creating a new <div>
element:
const newDiv = document.createElement("div");
Setting Attributes and Content
Once you have created an element, you can set its attributes and content using various properties and methods. For example, you can set the id
, class
, or style
attributes like this:
newDiv.id = "myDiv"; newDiv.className = "myClass"; newDiv.style.color = "red";
To set the content of the element, you can either set its textContent
property or use the innerHTML
property to include HTML markup:
newDiv.textContent = "Hello, World!"; // or newDiv.innerHTML = "<strong>Hello, World!</strong>";
Appending the Element to the DOM
After creating an element and setting its attributes and content, you need to append it to the DOM to make it visible on the web page. To do this, you can use the appendChild()
method on an existing element:
const container = document.getElementById("container"); container.appendChild(newDiv);
In this example, we assume that there is an element with the ID container
in our HTML document. The new <div>
element will be appended as a child of this element.
Removing an Element
To remove an element from the DOM, you can use the removeChild()
method on its parent element. You'll need to first get a reference to the parent element, and then call the method with the element you want to remove as an argument:
const elementToRemove = document.getElementById("elementToRemove"); elementToRemove.parentNode.removeChild(elementToRemove);
In this example, we assume that there is an element with the ID elementToRemove
in our HTML document.
Conclusion
You now know how to create and manipulate HTML elements dynamically using JavaScript in the browser. This can be useful for building interactive web pages, adding new content, or modifying existing content on the fly. Remember to practice these techniques and experiment with different elements and attributes to create more complex and engaging web experiences.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Using JavaScript (psst, it's free!).
FAQ
How do I create a new HTML element using JavaScript?
To create a new HTML element using JavaScript, you can use the document.createElement()
method. For example, to create a new div
element, you would write:
const newDiv = document.createElement("div");
How do I add the newly created element to the DOM?
You can add the newly created element to the DOM using the appendChild()
method. For example, to add the newDiv
created earlier to the body of the HTML document, you would write:
document.body.appendChild(newDiv);
How can I set attributes for the newly created element?
You can set attributes for the newly created element using the setAttribute()
method. For example, to set an id
attribute for the newDiv
created earlier, you would write:
newDiv.setAttribute("id", "myNewDiv");
How do I add text content to the newly created element?
You can add text content to the newly created element using the textContent
property. For example, to add text to the newDiv
created earlier, you would write:
newDiv.textContent = "This is a new div element!";
Can I create and add multiple elements at once using JavaScript?
Yes, you can create and add multiple elements by using loops or creating multiple instances of the createElement()
method. For example, to create three new paragraphs and add them to a div
element, you would write:
const containerDiv = document.createElement("div"); for (let i = 0; i < 3; i++) { const newParagraph = document.createElement("p"); newParagraph.textContent = `Paragraph ${i + 1}`; containerDiv.appendChild(newParagraph); } document.body.appendChild(containerDiv);