JavaScript Switch Statements
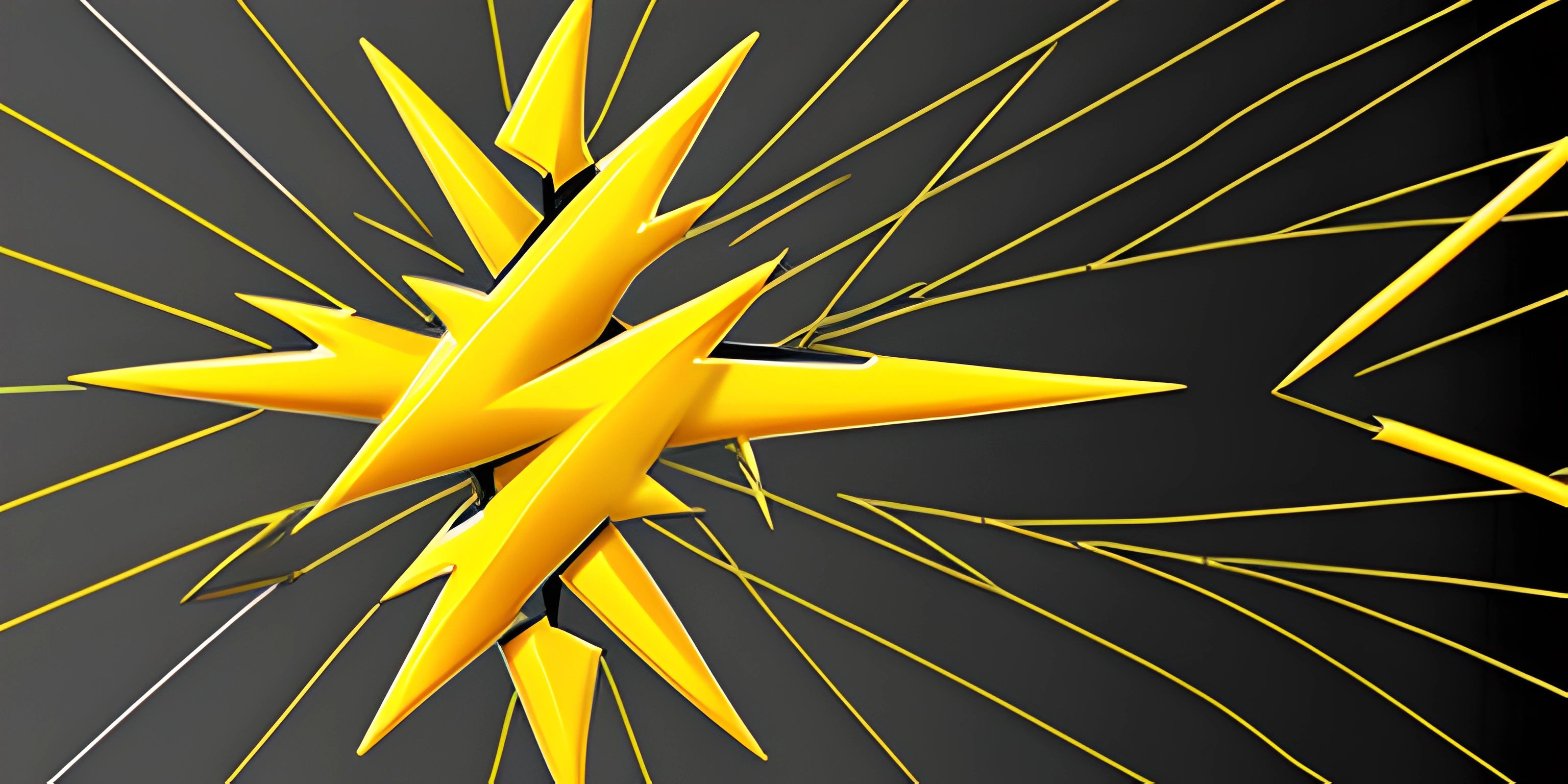
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Switch statements are a powerful way to handle multiple conditions in JavaScript. They are an alternative to using a series of if
, else if
, and else
statements. In this article, we will explore how to use switch statements effectively in your JavaScript code.
Syntax
A switch statement consists of an expression that is evaluated, followed by multiple case clauses that specify the code to execute if the expression matches a specific value. The basic syntax of a switch statement is as follows:
switch (expression) { case value1: // Code to execute if expression matches value1 break; case value2: // Code to execute if expression matches value2 break; // ... default: // Code to execute if none of the cases match }
Example
Let's say we want to display a message based on the day of the week. We can use a switch statement to efficiently handle this:
const day = new Date().getDay(); switch (day) { case 0: console.log("Sunday"); break; case 1: console.log("Monday"); break; case 2: console.log("Tuesday"); break; case 3: console.log("Wednesday"); break; case 4: console.log("Thursday"); break; case 5: console.log("Friday"); break; case 6: console.log("Saturday"); break; default: console.log("Invalid day"); }
In this example, the switch
statement evaluates the day
variable and compares it to each case. If the day matches a case, the corresponding message is displayed, and the break
statement prevents the code from continuing to the next case.
Tips
-
Don't forget to add a
break
statement after each case. If you don't include it, the code will continue to execute the next case even if the condition is not met, which can lead to unexpected results. -
Use the
default
case to specify code that should run if none of the cases match the expression. This is similar to using anelse
statement in anif
/else
structure. -
You can use any expression as the switch expression, not just simple variables. For example, you can use a function call, a calculation, or a string concatenation.
Now you know how to use switch statements in JavaScript to handle multiple conditions efficiently. Happy coding!
FAQ
What is a switch statement in JavaScript?
A switch statement in JavaScript is a form of conditional logic that allows you to efficiently execute different blocks of code based on the value of a particular expression. It is an alternative to using multiple if-else statements.
How do I write a switch statement in JavaScript?
To write a switch statement in JavaScript, use the following syntax:
switch (expression) { case value1: // code to be executed if the expression matches value1 break; case value2: // code to be executed if the expression matches value2 break; // and so on for other cases default: // code to be executed if the expression doesn't match any case }
Can I use multiple case values for a single block of code?
Yes, you can use multiple case values for a single block of code. To do this, simply list the case values one after another, without any code or break statements between them. For example: switch (expression) { case value1: case value2: case value3: // code to be executed if the expression matches any of the three values break; default: // code to be executed if the expression doesn't match any case }
How does the default case work in a switch statement?
The default case in a switch statement is executed when the given expression does not match any of the specified case values. It acts as a fallback and is optional. If there is no default case and none of the case values match the expression, the switch statement will simply do nothing. The default case can be placed anywhere in the switch statement but is often placed at the end for better readability.
Can I use switch statements with strings or other data types?
Yes, you can use switch statements with strings and other data types in JavaScript, not just numbers. The expression and case values can be any data type, as long as they can be compared using strict equality (===). For example: switch (someString) { case "apple": // code for apple break; case "banana": // code for banana break; default: // code for other fruits }